Implementing Domain-Driven Design in Legacy Golang Applications: Challenges and Strategies
Learn how to implement Domain-Driven Design (DDD) in legacy Golang applications. Overcome challenges, refactor code, and align with business needs.
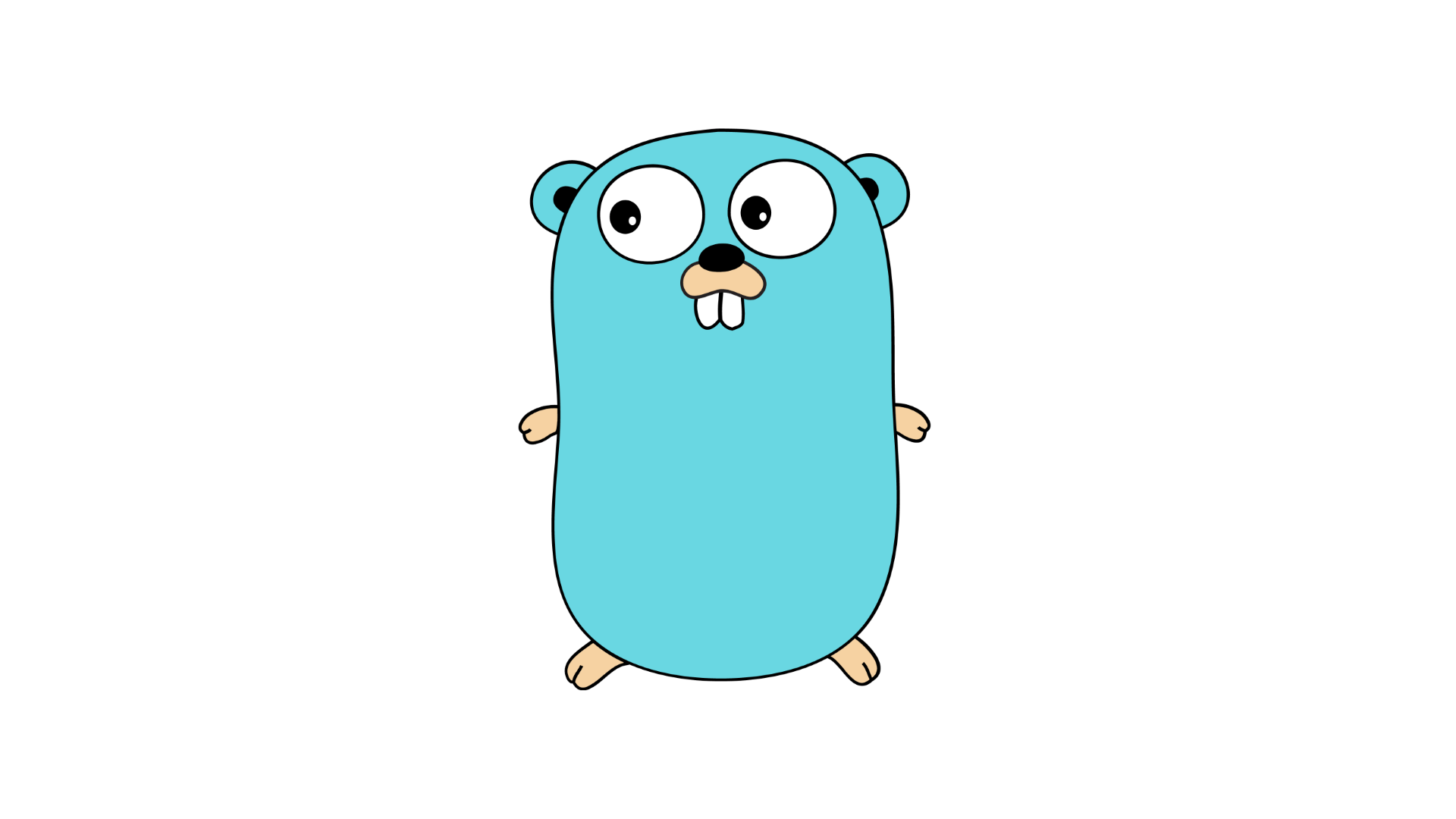
Introduction
Legacy Golang applications are those that have been in use for a while and have gradually become difficult to maintain, understand, and extend. The architecture and design decisions made in the past may no longer align with the principles and practices of Domain-Driven Design (DDD), which is a powerful approach for building complex software systems.
In this blog post, we'll explore the challenges of implementing Domain-Driven Design in legacy Golang applications and discuss strategies to overcome them. By adopting DDD principles and refactoring your legacy codebase, you can improve the maintainability, testability, and scalability of your application.
Understanding Domain-Driven Design
Domain-Driven Design is an approach to software development that emphasizes a deep understanding of the business domain. It provides a set of principles and patterns to structure applications based on the core business concepts.
The key concepts in DDD include:
- Domain: The subject area of the software system. It represents the business model, rules, and processes.
- Ubiquitous Language: A language shared by all team members, including developers and domain experts, to discuss the domain concepts and processes.
- Aggregates: Consistent and atomic units of data and behavior within the domain that are transactionally consistent.
- Entities: Objects with a unique identity that are mutable and have a lifecycle.
- Value Objects: Objects that represent a concept, are immutable, and do not have an identity.
- Repositories: Abstractions that provide a mechanism to store and retrieve domain entities.
- Services: Components that encapsulate domain operations that do not belong to a specific entity or value object.
- Domain Events: Messages that are emitted during domain operations to communicate and trigger side effects.
- Bounded Contexts: Segments of the domain with clear boundaries that enforce consistency.
By adopting DDD, you can create software systems that are highly maintainable, loosely coupled, and more aligned with the business needs.
Challenges of Implementing DDD in Legacy Golang Applications
When introducing Domain-Driven Design principles into legacy Golang applications, several challenges may arise. Let's discuss some of the common challenges:
1. Lack of Domain Knowledge and Ubiquitous Language
In many legacy applications, the original domain knowledge may have been lost or diluted over time. This makes it difficult to identify the business concepts and define a consistent ubiquitous language that is shared by the development team and domain experts.
Strategy: To overcome this challenge, it's essential to involve domain experts in the software development process. Conduct workshops or interviews to understand the core concepts and clarify the language used to describe them. Document the shared vocabulary and make it the foundation for all discussions and code.
2. Tightly Coupled Components and Dependencies
In legacy applications, components and modules are often tightly coupled and rely on each other's internal implementation details. This makes it difficult to isolate and test individual components, leading to a lack of modularity and flexibility.
Strategy: To address this challenge, start by identifying the core domains and separating them into independent packages. Define clear boundaries between packages using interfaces and ensure loose coupling between them. Use dependency injection to manage dependencies and facilitate testing.
3. Lack of Unit Tests and Automated Tests
Legacy applications often lack comprehensive unit tests and automated integration tests. This makes it challenging to validate changes and ensure that existing functionality remains intact throughout refactoring.
Strategy: Begin by identifying critical components and writing unit tests for them. Gradually increase the test coverage by adding tests for the most vulnerable areas of the codebase. Run automated tests frequently to catch and resolve issues early.
4. Complex Business Logic
In legacy applications, business logic tends to be scattered across various components, making it challenging to understand the overall flow and behavior of the system.
Strategy: Start by identifying the core business processes and consolidating the business logic within the appropriate domain objects and services. Ensure that each component has a clear responsibility and represents a meaningful concept in the domain. Refactor complex logic into smaller, more manageable units.
Strategies for Implementing Domain-Driven Design in Legacy Golang Applications
Now that we've discussed the common challenges, let's explore some strategies to implement Domain-Driven Design principles in legacy Golang applications:
1. Gradual Refactoring
Instead of attempting a complete overhaul of the system, adopt a gradual refactoring approach. Start by identifying the most critical and stable parts of the application and refactor them using DDD principles. This iterative process allows for incremental improvements while minimizing the risk of introducing new bugs or disrupting business operations.
2. Identifying Core Domains and Bounded Contexts
Analyze the existing application and identify the core domains or areas with the most business value. Define bounded contexts around these domains and ensure that each context represents a cohesive business area with clear boundaries. Apply DDD principles specifically to these bounded contexts to gradually improve the overall architecture.
3. Applying DDD Patterns
Utilize DDD patterns such as aggregates, entities, value objects, repositories, and services to structure the codebase. Introduce domain events to enable communication between components and enforce consistency.
4. Minimizing Dependencies
Identify and minimize dependencies between components and packages. Use dependency injection and inversion of control to decouple modules, making them easier to test, maintain, and extend.
5. Improving Test Coverage
Focus on increasing the test coverage of critical components and enhancing the automated test suite. Write unit tests to ensure the correctness of individual components and integration tests to validate the behavior of the system as a whole.
Conclusion
Implementing Domain-Driven Design in legacy Golang applications is a challenging but rewarding process. By gradually refactoring the codebase, applying DDD principles, and adhering to the ubiquitous language, you can significantly improve the maintainability, testability, and scalability of your application.
Remember to involve domain experts, minimize dependencies, and invest in comprehensive test coverage. These strategies will help you adapt your legacy codebase to a more domain-centric approach, aligning your application more closely with the core business requirements.
Embrace the challenges and embark on the journey of refactoring your legacy Golang applications with Domain-Driven Design!