How to Implement Domain-Driven Design in Golang Projects
Learn how to implement Domain-Driven Design (DDD) principles in Golang projects. Explore concepts like ubiquitous language, bounded contexts, aggregates, domain events, and repositories to create scalable and maintainable codebases.
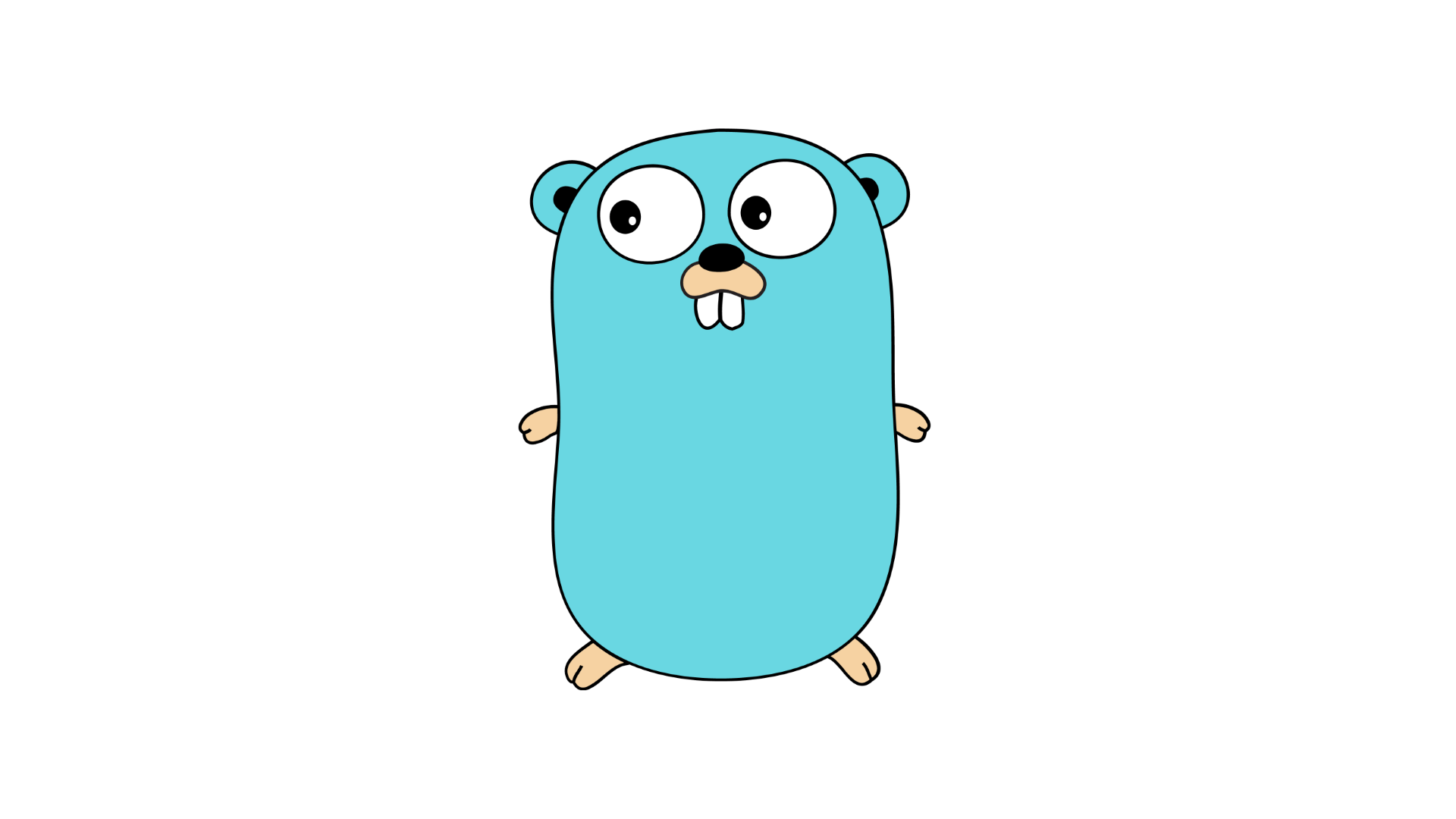
Introduction
Domain-Driven Design (DDD) is a software development approach that focuses on understanding and modeling the business domain of an application. By putting the domain at the center of the design process, DDD promotes a more maintainable and scalable codebase.
In this blog post, we'll explore how to implement Domain-Driven Design in Golang projects. We'll discuss the key principles of DDD and demonstrate how to apply them using Go's features and best practices. By the end of this tutorial, you'll have a solid understanding of how to incorporate DDD concepts into your Go projects.
What is Domain-Driven Design?
Domain-Driven Design is an approach to software development that emphasizes the importance of understanding and modeling the business domain. The domain is the core of the software application, encompassing the subject matter or problem space the software aims to solve.
DDD encourages developers to collaborate closely with domain experts, such as business analysts or domain specialists, to gain a deep understanding of the domain. By doing so, developers can create a model that accurately reflects the concepts, rules, and relationships within the domain.
Key Principles of Domain-Driven Design
Before we dive into implementing DDD in Golang, let's briefly explore the key principles of Domain-Driven Design:
1. Ubiquitous Language
Ubiquitous Language refers to a common language shared by developers and domain experts. By using a shared language, developers can bridge the gap between technical jargon and domain-specific terminology. This shared understanding helps ensure clear communication and alignment between all stakeholders.
2. Bounded Context
Bounded Context defines a specific boundary within which a model operates. The idea is to divide a complex system into smaller, more manageable contexts. Each bounded context has its own language, set of rules, and concepts that may differ from other contexts. This separation allows for better isolation and encapsulation.
3. Aggregates
Aggregates are collections of related objects that act as a single unit. They enforce consistency and protect the integrity of the domain model. Aggregates are responsible for ensuring that all invariants and business rules are maintained.
4. Domain Events
Domain Events represent significant occurrences within the domain. They capture changes and trigger side effects in other parts of the system. Domain Events enable loose coupling and provide a way to communicate and synchronize different parts of the system.
5. Repositories
Repositories provide an abstraction for storing and retrieving domain objects. They encapsulate the data access logic and provide a consistent interface for working with the data layer. Repositories decouple the domain model from the persistence details.
Implementing Domain-Driven Design in Golang Projects
Now that we have a basic understanding of the key principles of DDD, let's explore how to implement them in Golang projects:
1. Use Ubiquitous Language
The first step in implementing DDD in Go is to establish a ubiquitous language. To achieve this, collaborate closely with domain experts to develop a common vocabulary that accurately represents the domain. Use this shared language throughout your codebase, including variable and function names, comments, and documentation.
2. Define Bounded Contexts
Identify the bounded contexts within your domain. Each bounded context should have a separate package in your Go project. This promotes modularity and encapsulation, making it easier to reason about each context independently.
For example, if you are building an e-commerce application, you might have separate packages for "Order", "Payment", and "Inventory". Each of these packages represents a distinct bounded context with its own set of models, business logic, and repositories.
3. Design Aggregates
Identify the aggregates within each bounded context. An aggregate represents a group of related objects that are treated as a single unit. In Go, you can use structs to define aggregates along with their associated methods.
For example, in the "Order" bounded context, you might have an "Order" struct representing an individual order. The "Order" struct would contain all the necessary fields and methods to encapsulate the logic related to an order.
4. Work with Domain Events
Identify and define domain events within your model. Domain events represent significant occurrences within the domain and are used to communicate changes to other parts of the system. In Go, you can represent a domain event as a struct.
For example, in the "Order" bounded context, you might define a "OrderCreated" event struct that contains relevant information about an order creation. This event can be published when an order is created and subscribed to by other parts of the system that need to be notified of the event.
5. Abstract Data Access with Repositories
Decouple the domain model from the data access layer by using repositories. A repository provides an abstraction for storing and retrieving domain objects. In Go, you can define interfaces for repositories and their corresponding implementations.
For example, in the "Order" bounded context, you might define an "OrderRepository" interface with methods like "Save", "FindById", and "Delete". Implement the repository interface in a separate package that handles the data access logic specific to the chosen persistence mechanism (e.g., a PostgreSQL database).
Conclusion
By implementing Domain-Driven Design in your Golang projects, you can create more maintainable, scalable, and domain-focused codebases. By leveraging Go's features and best practices, such as struct composition and package design, you can effectively apply the key principles of DDD.
In this blog post, we explored the essential principles of DDD and demonstrated how to implement them in Go projects. We discussed using a ubiquitous language, defining bounded contexts, designing aggregates, working with domain events, and abstracting data access with repositories.
Now it's your turn to apply these concepts in your own Golang projects. Remember to continuously iterate and refine your domain model based on your evolving understanding of the domain. Happy coding!