Implementing CQRS (Command Query Responsibility Segregation) in Golang with Domain-Driven Design
"CQRS separates read and write operations in Golang applications, improving performance and scalability. Learn how to implement CQRS with DDD in this blog post."
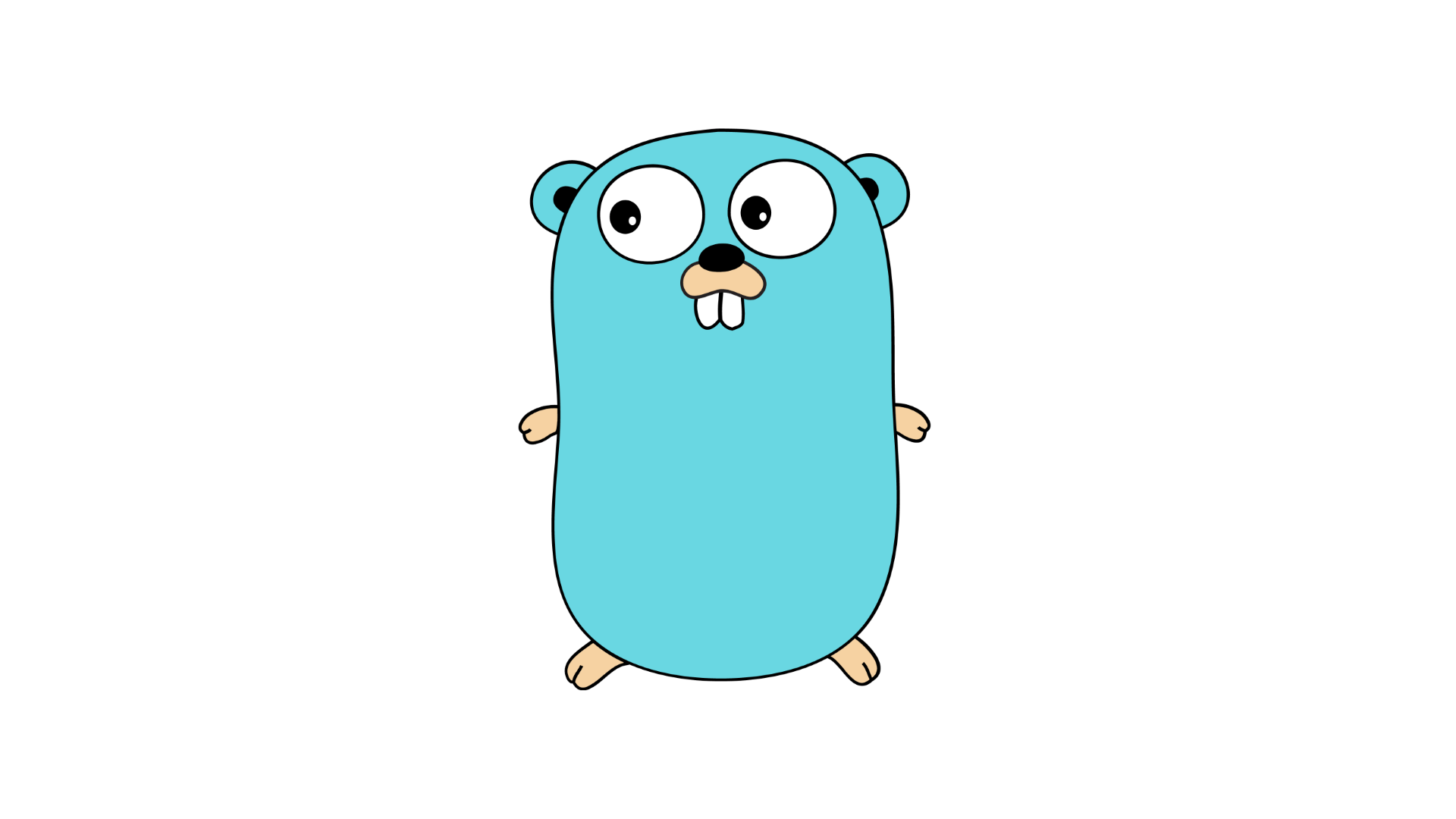
Implementing CQRS (Command Query Responsibility Segregation) in Golang with Domain-Driven Design
In this blog post, we will explore the concept of Command Query Responsibility Segregation (CQRS) in the context of building applications using Golang and Domain-Driven Design (DDD). We will discuss what CQRS is, its benefits, and how to implement it effectively in Golang.
Introduction to CQRS
CQRS is a design pattern that separates the read and write operations into separate models, each with its own set of responsibilities. The main idea behind CQRS is to optimize the performance and scalability of applications by tailoring the data access patterns to their specific needs.
Traditionally, most applications follow a CRUD (Create, Read, Update, Delete) model where a single data model handles all types of operations. However, in complex business domains, this approach can result in performance bottlenecks and difficulties in maintaining code.
CQRS solves these problems by segregating the read and write operations into separate models:
- Command Model: Handles write operations and enforces business rules and validations.
- Query Model: Handles read operations and provides optimized data access for query-intensive operations.
This separation allows each model to be optimized for its specific role, resulting in improved performance, scalability, and maintainability.
Benefits of CQRS
CQRS offers several benefits, including:
- Improved Performance: By separating read and write operations, you can optimize data access patterns, resulting in faster response times for read-intensive or write-intensive operations.
- Scalability: CQRS allows you to scale read and write operations independently, which can be crucial for high-demand applications.
- Better Domain Modeling: CQRS facilitates building a more accurate and expressive domain model by separating the concerns of reading and writing data.
- Flexibility: With CQRS, you can choose different persistence mechanisms for the write and read models, allowing you to use the most suitable technology for each case.
- Maintainability: The separation of concerns in CQRS leads to smaller, more focused codebases, making it easier to understand, test, and maintain the code.
Implementing CQRS in Golang
Golang is a great language for implementing CQRS due to its simplicity, concurrency support, and performance. Let's explore the steps to implement CQRS in a Golang application.
1. Define the Domain Model
The first step in implementing CQRS is to define your domain model. This model should capture the essential entities, value objects, and business rules of your application domain. Start by identifying the main aggregates and their relationships.
For example, let's consider a simple e-commerce application with two aggregates: Order
and Product
. The Order
aggregate represents an order placed by a customer, and the Product
aggregate represents a product available for sale.
Define the structures representing these aggregates, along with their methods for performing business logic.
type Order struct {
ID string
UserID string
// ... other order fields
}
type Product struct {
ID string
Name string
Price float64
// ... other product fields
}
2. Segregate Commands and Queries
In CQRS, we separate commands (write operations) and queries (read operations). This segregation can be achieved using separate packages or directory structures.
Create a package or directory for commands and another for queries. In each package, define the necessary interfaces and structs to perform the operations.
Command Package:
package command
type OrderCommandService interface {
CreateOrder(order Order) error
UpdateOrder(order Order) error
// ... other order write operations
}
Query Package:
package query
type OrderQueryService interface {
GetOrder(orderID string) (Order, error)
GetAllOrders(userID string) ([]Order, error)
// ... other order read operations
}
3. Implement the Command Handler
The command handler is responsible for processing the commands and updating the write models accordingly. It should validate the commands, enforce business rules, and persist the changes.
Create a new package or directory for the command handlers and define the necessary structs and methods. Implement the command handler methods as per your business requirements.
package handler
type OrderCommandHandler struct {
orderService OrderCommandService
}
func (h *OrderCommandHandler) CreateOrder(order Order) error {
// Validate the order
if err := validateOrder(order); err != nil {
return err
}
// Enforce business rules
// ...
// Persist the changes
if err := h.orderService.CreateOrder(order); err != nil {
return err
}
// Publish events or trigger other actions
// ...
return nil
}
// ... other command handler methods
4. Implement the Query Handler
The query handler is responsible for retrieving data from the read models based on queries. It should provide optimized data retrieval methods for various read operations.
Create a new package or directory for the query handlers and define the necessary structs and methods. Implement the query handler methods as per your business requirements.
package handler
type OrderQueryHandler struct {
orderService OrderQueryService
}
func (h *OrderQueryHandler) GetOrder(orderID string) (Order, error) {
return h.orderService.GetOrder(orderID)
}
func (h *OrderQueryHandler) GetAllOrders(userID string) ([]Order, error) {
return h.orderService.GetAllOrders(userID)
}
// ... other query handler methods
5. Wire Up the Dependencies
Finally, wire up the dependencies required for the command and query handlers. Implement the required services and repositories based on your chosen persistence mechanism.
Consider using a dependency injection framework like Wire or manually wire up the dependencies in your main function.
func main() {
// Create and initialize the command and query handlers
orderCommandService := // Initialize your order command service
orderQueryService := // Initialize your order query service
orderCommandHandler := &handler.OrderCommandHandler{
orderService: orderCommandService,
}
orderQueryHandler := &handler.OrderQueryHandler{
orderService: orderQueryService,
}
// Start your application and handle incoming commands and queries
// ...
}
Conclusion
In this blog post, we explored the concept of Command Query Responsibility Segregation (CQRS) and its benefits. We also discussed how to implement CQRS in a Golang application using domain-driven design principles.
CQRS provides a powerful way to optimize performance, scalability, and maintainability of applications by segregating the read and write operations. By implementing CQRS in Golang, you can leverage its simplicity, concurrency support, and performance to build robust and efficient applications.
Remember that CQRS is not a silver bullet and should be used judiciously based on your application's specific requirements. However, when properly applied, it can offer significant advantages in complex domains.
Happy coding!