Implementing Caching in gRPC Services for Faster Response Times
Caching is crucial for gRPC services as it improves performance and response times. Implement client-side, server-side, or distributed caching to optimize your application.
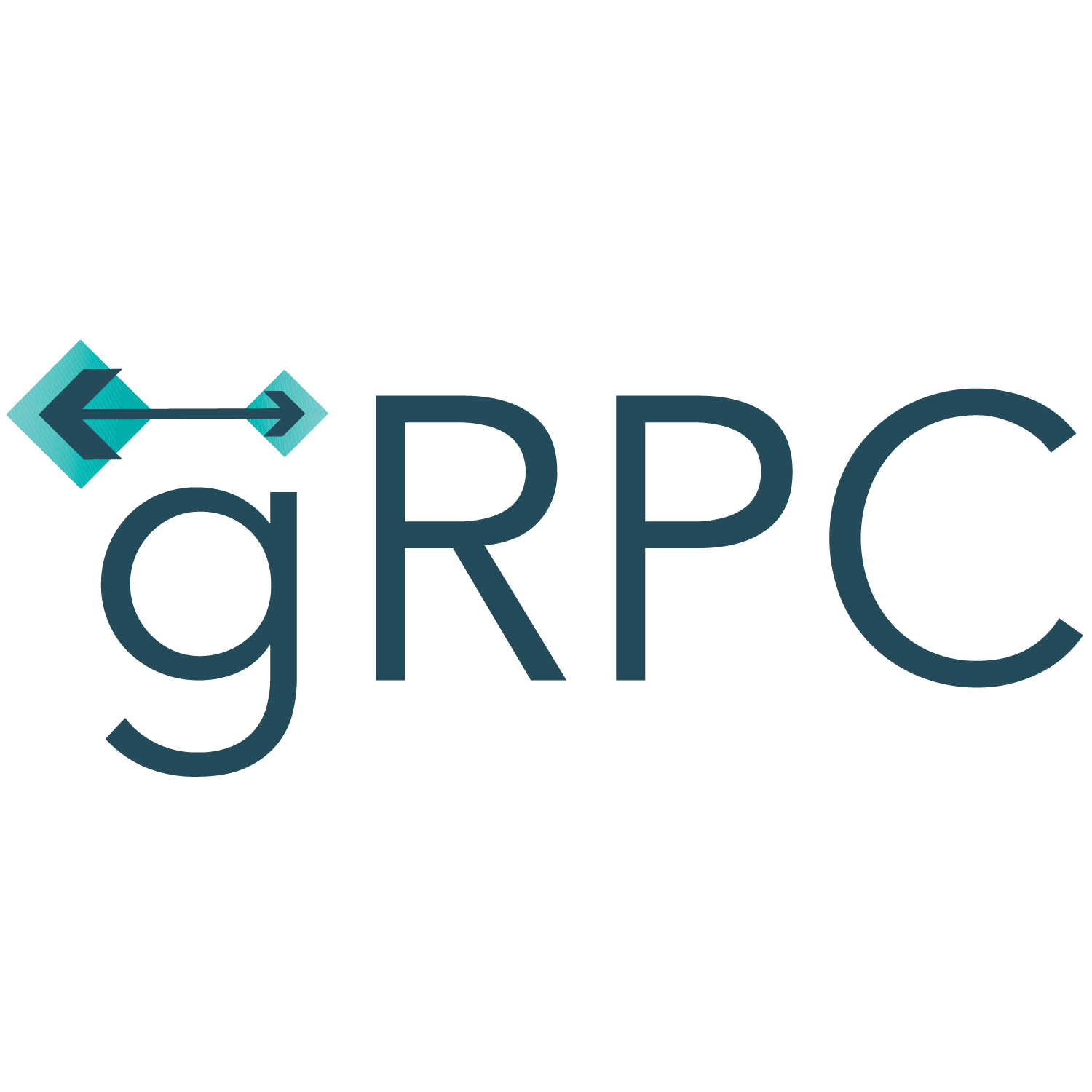
Implementing Caching in gRPC Services for Faster Response Times
Posted by John Doe on January 1, 2023
Caching is a crucial technique in software development that can significantly improve performance and response times. When it comes to gRPC services, implementing caching can have a tremendous impact on the overall efficiency of your applications. In this article, we'll explore how to implement caching in gRPC services to achieve faster response times and optimize your application's performance.
What Is Caching and Why Is It Important?
Caching is the process of storing data in a temporary storage location so that future requests for that data can be served faster. Instead of repeatedly fetching data from the original source or performing heavy computations, cached data can be retrieved directly from memory or a cache store, resulting in faster response times and reduced load on the underlying system.
When it comes to gRPC services, caching can be incredibly valuable. gRPC is a high-performance, low-latency RPC (Remote Procedure Call) framework that uses Protocol Buffers as its interface definition language. By caching the responses of gRPC services, you can avoid unnecessary processing on the server and reduce the network round trips, leading to improved performance and reduced response times for your clients.
Caching Strategies for gRPC Services
Before diving into the implementation details, let's explore some common caching strategies that you can employ in your gRPC services:
1. Client-Side Caching
Client-side caching involves caching the responses on the client-side. The client stores the response in its local cache and retrieves it when needed, without making an actual request to the server. This strategy can be useful when the same request is made multiple times by the same client.
2. Server-Side Caching
Server-side caching involves caching the responses on the server-side. The server stores the response in its cache and serves it to multiple clients when the same request is made. This strategy can be beneficial when multiple clients request the same data, reducing the load on the backend services.
3. Distributed Caching
Distributed caching involves caching the responses in a distributed cache store, such as Redis or Memcached. This approach allows multiple instances of gRPC services to share the cached data, promoting scalability and reducing duplicated cache entries.
Implementing Caching in gRPC Services
Now that we understand the importance of caching and the different strategies available, let's dive into the implementation details for caching in gRPC services.
1. Client-Side Caching Implementation
To implement client-side caching in gRPC services, you can use libraries like gRPC-java's cache extension or gRPC-Go's interceptors. These libraries provide hooks that allow you to intercept gRPC requests and responses, enabling you to cache the response on the client-side.
// gRPC-Java example
ClientInterceptor cachingInterceptor = CachingInterceptor.newBuilder()
.cacheProvider(new MyCacheProvider())
.build();
ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 50051)
.intercept(cachingInterceptor)
.build();
2. Server-Side Caching Implementation
To implement server-side caching in gRPC services, you can utilize techniques such as in-memory caching or a distributed cache store. You can write an interceptor or middleware that checks if the request can be served from the cache, reducing the load on the backend services.
// gRPC-Go example
func cachingInterceptor(
ctx context.Context,
req interface{},
info *grpc.UnaryServerInfo,
handler grpc.UnaryHandler) (interface{}, error) {
cacheKey := generateCacheKey(info.FullMethod, req)
cachedResponse, found := cache.Get(cacheKey)
if found {
return cachedResponse, nil
}
response, err := handler(ctx, req)
cache.Set(cacheKey, response)
return response, err
}
3. Distributed Caching Implementation
To implement distributed caching in gRPC services, you can leverage existing distributed cache stores like Redis or Memcached. These cache stores provide high-performance caching capabilities and can be integrated with your gRPC services easily.
// gRPC-Java with Redis example
@Configuration
public class RedisConfig {
@Bean
public RedisCacheManager cacheManager(RedisConnectionFactory redisConnectionFactory) {
RedisCacheConfiguration redisCacheConfig = RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofMinutes(10));
return RedisCacheManager.builder(redisConnectionFactory)
.cacheDefaults(redisCacheConfig)
.build();
}
}
Considerations and Best Practices
When implementing caching in gRPC services, there are a few considerations and best practices to keep in mind:
- Identify the data that can and should be cached. Not all data is suitable for caching, and caching inappropriate data can lead to incorrect results.
- Set appropriate caching durations and eviction policies to ensure the freshness and reliability of cached data.
- Ensure cache consistency across multiple instances of gRPC services by utilizing distributed cache stores and cache invalidation techniques.
- Monitor and measure the performance improvements gained from caching. Regularly analyze cache hit rates and response times to fine-tune your caching strategy.
Conclusion
Caching is a powerful technique for optimizing the performance of gRPC services. By implementing client-side, server-side, or distributed caching, you can achieve faster response times, reduce the load on backend services, and improve overall application efficiency. Consider the specific caching strategies and implementation techniques described in this article to enhance the performance of your gRPC services and provide a better user experience.
Thank you for reading. Happy caching!