Bounded Contexts in Golang: Isolating and Managing Complexity in Domain-Driven Design
Learn how to implement bounded contexts in Golang and manage complexity in your applications using domain-driven design principles.
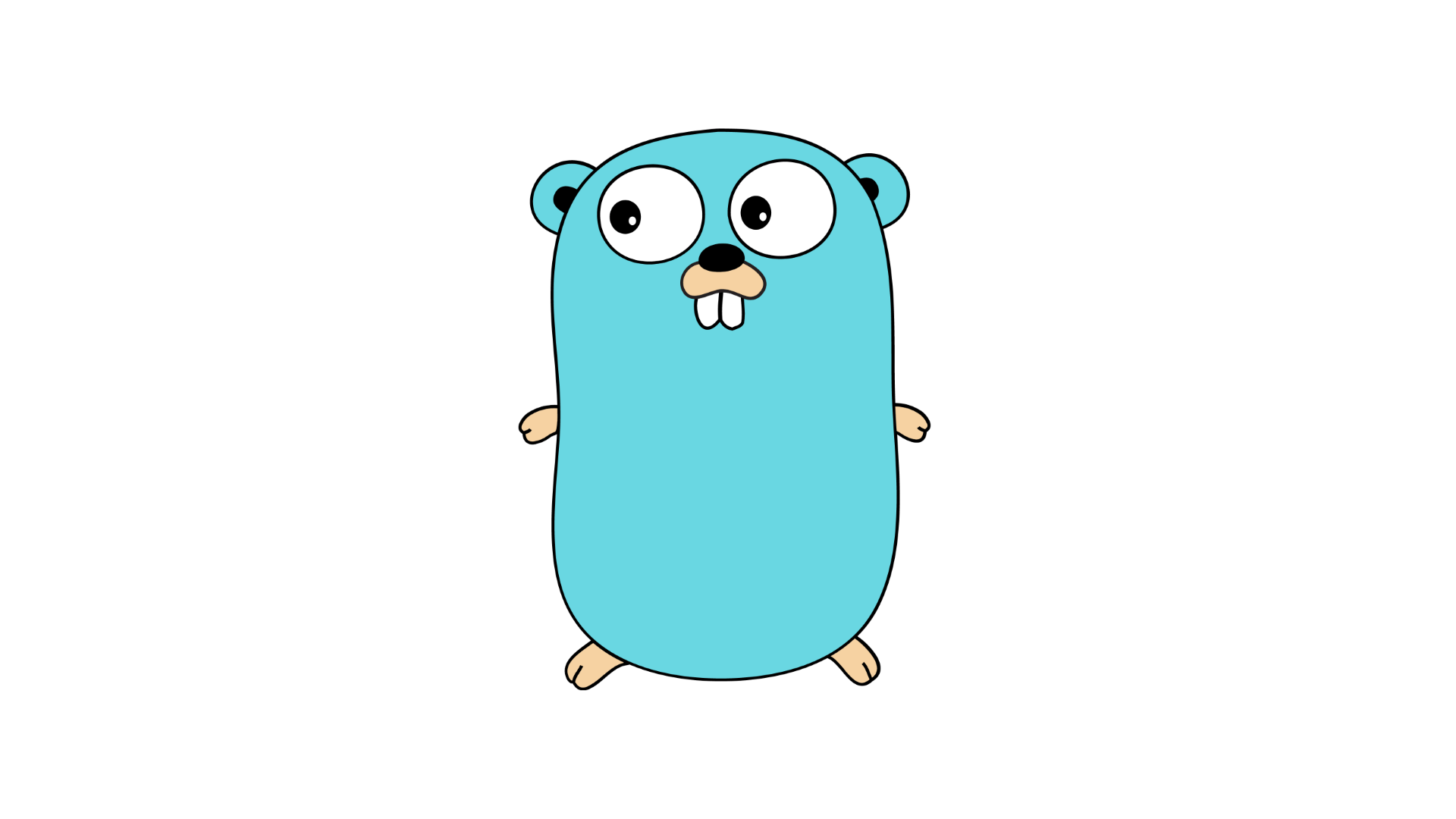
Introduction
Welcome to another blog post in our series on domain-driven design in Golang. In this article, we're going to explore the concept of bounded contexts and how they can help in isolating and managing complexity in your applications. Understanding bounded contexts is essential for building maintainable and scalable software systems using domain-driven design principles.
What are Bounded Contexts?
In domain-driven design (DDD), a bounded context is a language and organizational boundary within which a particular model is defined and applies. It allows for different models, concepts, and terminology to exist in different parts of your application, based on the specific domain and requirements.
In simpler terms, a bounded context encapsulates a specific part of your application where a specific set of domain concepts and rules apply. It helps to isolate and manage complexity by breaking down a large software system into smaller, more manageable and understandable parts.
Why are Bounded Contexts Important?
When building complex software systems, it's common to have multiple subdomains or areas of functionality that have different requirements, rules, and constraints. Trying to tackle all of these together in a monolithic manner can lead to confusion, maintenance issues, and unnecessary complexity.
Bounded contexts enable you to create separate models and designs for different parts of your system, allowing you to focus and reason about each area independently. This isolation simplifies understanding, testing, and maintaining the codebase in the long-term.
Additionally, bounded contexts also help to align the development process with the organizational structure. Different teams or individuals can work on different bounded contexts independently, reducing coordination efforts and allowing for faster development cycles.
Implementing Bounded Contexts in Golang
Now that we understand the importance of bounded contexts, let's see how we can implement them in Golang. Golang's strong type system, package management, and design principles make it an excellent language choice for building complex systems using domain-driven design.
1. Organize Your Golang Projects
When implementing bounded contexts in Golang, it's crucial to have a well-organized project structure. Start by creating a separate directory for each bounded context within your project's source directory.
Example Project Structure:
.
└── project-name
├── cmd
│ ├── main.go
│ └── ...
├── internal
│ ├── context1
│ │ └── ...
│ ├── context2
│ │ └── ...
│ └── ...
└── ...
In the above example, we created separate directories for different bounded contexts under the internal directory. This structure allows us to isolate and manage the code related to each bounded context effectively.
2. Modularize Your Codebase
Within each bounded context, you can further divide your code into modules or packages based on the functionality and domain concepts they represent. Modularization helps in keeping the codebase manageable and allows for better separation of concerns.
Consider a bounded context that deals with managing user authentication and another bounded context that handles order processing. You can have separate modules or packages for authentication, user management, and order processing, within their respective bounded context directories.
Example Modularized Codebase:
.
└── project-name
├── internal
│ ├── authentication
│ │ └── ...
│ ├── usermanagement
│ │ └── ...
│ ├── orderprocessing
│ │ └── ...
│ └── ...
└── ...
By breaking down your code into smaller, modular components, you can focus on specific business concepts within each bounded context, making the development process more efficient and maintainable.
3. Define Clear Interfaces
In Golang, interfaces are a powerful tool for defining contracts between different parts of your system. By explicitly defining interfaces for communication between bounded contexts, you can enforce clear communication boundaries and ensure that each bounded context works in isolation.
Example Interface Definition:
package usermanagement
type UserRepository interface {
CreateUser(user *User) error
GetUserByID(userID string) (*User, error)
...
}
In the above example, we defined an interface UserRepository within the usermanagement package. This interface defines the contract for interacting with the user repository within the user management bounded context.
Other bounded contexts can leverage this interface to interact with the user management functionality without being tightly coupled to its implementation details.
4. Use Good Naming Conventions
When implementing bounded contexts in Golang, it's important to use clear and meaningful names for your packages, directories, files, and variables. This helps in understanding the codebase without the need for extensive documentation and reduces confusion when working with multiple bounded contexts.
Follow package and variable naming conventions recommended by the Golang community to ensure consistency and improve code readability.
5. Communication and Testing
For effective communication between bounded contexts, you can use techniques like API contracts, event-driven architectures, or message queues. These approaches help in decoupling different parts of your system and promoting loose coupling between bounded contexts.
Additionally, unit testing and integration testing play a vital role in ensuring the correctness and compatibility of your bounded contexts. Write tests that cover both individual bounded contexts and the interaction between different bounded contexts.
Conclusion
Bounded contexts are a powerful tool in domain-driven design that help in isolating and managing complexity in your applications. By breaking down your software system into smaller, understandable parts, you can enhance maintainability, scalability, and teamwork.
In this article, we explored how to implement bounded contexts in Golang, from organizing your projects and modularizing your codebase to defining clear interfaces and using good naming conventions. By following these best practices, you'll be able to build maintainable and scalable software systems using domain-driven design principles in Golang.
Stay tuned for the next article in our series on domain-driven design, where we'll dive deeper into the world of aggregates and aggregate roots. Happy coding!