Implementing Authentication and Authorization in Golang: A Practical Guide
Learn how to implement authentication and authorization in Golang with our practical guide. Secure your applications and control user access with step-by-step instructions.
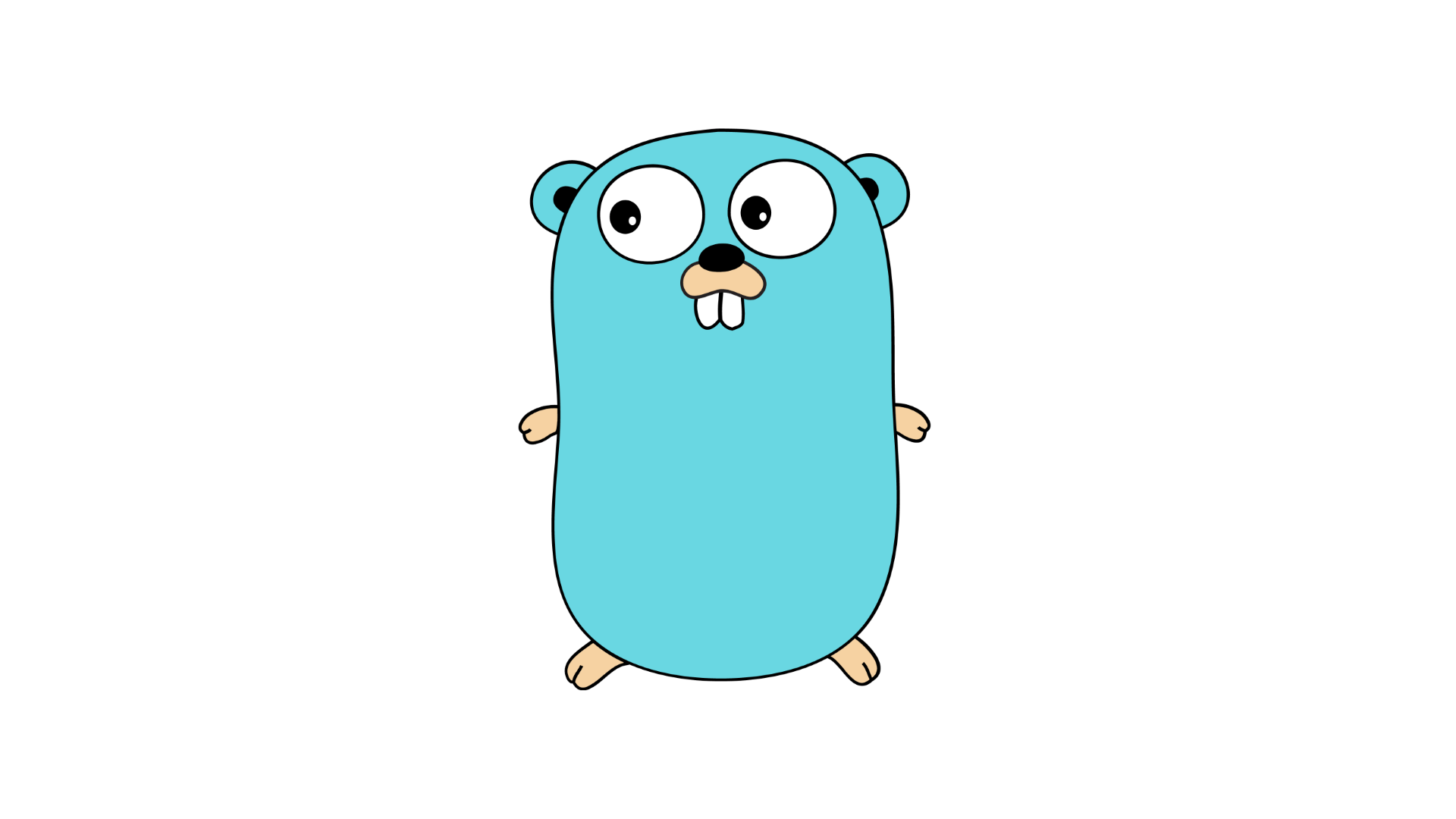
Introduction
Welcome to our practical guide on implementing authentication and authorization in Golang. In this guide, we will explore the intricacies of securing your Golang applications by adding authentication and authorization functionalities. Whether you are a beginner or have some experience with Golang, this guide will provide you with a step-by-step approach to implementing these crucial security features.
Understanding Authentication and Authorization
Before we dive into the implementation details, let's first understand the concepts of authentication and authorization.
Authentication: Authentication is the process of verifying the identity of a user or system. It ensures that the user or system accessing the application is who they claim to be. Common authentication mechanisms include username and password, tokens, certificates, and biometrics.
Authorization: Authorization is the process of granting or denying access to specific resources or functionalities based on the authenticated user's permissions. It ensures that only authorized users can perform certain actions within the application. Authorization is usually implemented using roles, permissions, or access control lists.
Implementing Authentication
In this section, we will walk you through the process of implementing authentication in your Golang application. Follow these steps:
Step 1: Choose an Authentication Mechanism
Before you start, you need to decide which authentication mechanism best suits your application's requirements. Here are a few commonly used authentication mechanisms in Golang:
- Username and Password: The user provides a username and password combination to authenticate.
- Tokens: Tokens are issued to authenticated users and are used for subsequent requests.
- OAuth 2.0: OAuth 2.0 is an industry-standard protocol that allows users to authenticate with third-party providers like Google, Facebook, or GitHub.
Step 2: Add Authentication Endpoints
Next, you need to add the necessary authentication endpoints to your Golang application. These endpoints will handle the authentication process and generate the necessary tokens or session cookies.
Step 3: Validate User Credentials
Once the user submits their credentials, validate them against the stored user credentials. This step ensures that the user is providing correct credentials and is authorized to access the application.
Step 4: Generate and Manage Tokens
If the user authentication is successful, you need to generate a token that can be used for subsequent requests. Tokens can be in the form of JSON Web Tokens (JWT) or session cookies. Implement logic to manage the expiration, revocation, and renewal of these tokens.
Implementing Authorization
Once you have implemented authentication, it's time to move on to authorization. Implementing authorization involves the following steps:
Step 1: Define Roles and Permissions
Start by defining the roles and permissions that will be used to control access to your application's resources. Roles define the user's level of access, while permissions define the specific actions a user can perform.
Step 2: Associate Roles and Permissions
Associate the defined roles with the appropriate permissions. This association can be stored in a database or defined in code, depending on your application's requirements.
Step 3: Check Authorization for Secured Endpoints
For secured endpoints, you need to validate the user's authorization before allowing access. This validation involves checking if the user's role has the necessary permissions to access the specific resource or perform the requested action.
Conclusion
Congratulations! You have successfully implemented authentication and authorization in your Golang application. By following the steps outlined in this practical guide, you have taken a significant step towards securing your application and protecting your users' data. Remember to test your implementation thoroughly and keep an eye on any emerging security vulnerabilities or best practices.
Stay tuned for more articles where we will dive deeper into Golang security best practices and explore advanced authentication and authorization techniques. Happy coding!