Server-side Rendering with Angular Universal: Boosting Performance and SEO
Learn how to implement server-side rendering (SSR) in your Angular applications using Angular Universal. Improve performance and SEO with pre-rendered content and faster time-to-first-content. Boost your Angular app's accessibility and visibility with SSR.
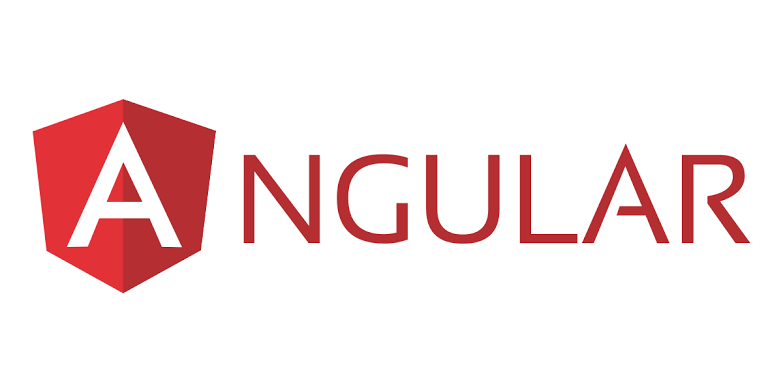
Introduction
Server-side rendering (SSR) is a technique that can significantly boost the performance and improve the search engine optimization (SEO) of your Angular applications. By rendering the web page on the server and sending the fully rendered HTML to the client, SSR reduces the time-to-first-content and ensures that search engines can easily crawl and index your site.
In this guide, we'll explore Angular Universal, the official solution for SSR in Angular applications. We'll learn how to set up, configure, and implement server-side rendering in our projects, and we'll also discuss the benefits of SSR for performance and SEO. Let's dive in!
What is Angular Universal?
Angular Universal is a package provided by the Angular team that brings server-side rendering to Angular applications. It allows you to run your Angular app on the server and generate a fully rendered HTML response that can be sent to the client.
By pre-rendering your Angular app on the server, Angular Universal improves the initial page load time, as users get to see the pre-rendered content while the JavaScript bundles are being downloaded and executed. This results in faster time-to-first-content and a better user experience.
Setting up Angular Universal
To use Angular Universal, you need to set up a few things in your Angular project. Follow these steps:
1. Create a new Angular project
If you haven't already created an Angular project, you can start by generating a new project using the Angular CLI:
npx @angular/cli new my-app
Replace my-app
with the desired name for your project.
2. Install Angular Universal CLI
To install the Angular Universal CLI, run the following command:
ng add @nguniversal/express-engine
This command will install the necessary dependencies and configure your project for Angular Universal.
3. Generate server-side rendering code
Next, generate the server-side rendering code by running the following command:
ng generate universal --clientProject=my-app
Replace my-app
with the name of your Angular project.
This command will generate the server-side rendering code in the server
directory of your project.
4. Build and serve your Angular app on the server
To build and serve your Angular app on the server, use the following command:
npm run build:ssr && npm run serve:ssr
This command will build your Angular app for server-side rendering and start a server to serve the rendered pages.
Your Angular app is now set up for server-side rendering using Angular Universal!
Implementing Server-side Rendering
Now that we have set up Angular Universal, let's implement server-side rendering in our Angular application. Follow these steps:
1. Update the App Routing Module
In your app-routing.module.ts
file, import the RouterModule
from @angular/router
and use the RouterModule.withServerTransition()
method to set up the server transition configuration:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
const routes: Routes = [
// Your routes here
];
@NgModule({
imports: [RouterModule.forRoot(routes, {
initialNavigation: 'enabled'
})],
exports: [RouterModule]
})
export class AppRoutingModule { }
2. Update the App Module
In your app.module.ts
file, import the BrowserModule
from @angular/platform-browser
and the BrowserAnimationsModule
from @angular/platform-browser/animations
. Then, import the ServerModule
and the ServerTransferStateModule
from @angular/platform-server
:
import { BrowserModule } from '@angular/platform-browser';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { NgModule } from '@angular/core';
import { ServerModule } from '@angular/platform-server';
import { ServerTransferStateModule } from '@angular/platform-server';
Remove any mention of the app.component.css
file from the styles
array.
In the imports
array, replace BrowserModule
with BrowserModule.withServerTransition({ appId: 'my-app' })
. Add the BrowserAnimationsModule
, ServerModule
, and ServerTransferStateModule
to the imports
array as well:
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule.withServerTransition({ appId: 'my-app' }),
BrowserAnimationsModule,
ServerModule,
ServerTransferStateModule
],
bootstrap: [AppComponent]
})
export class AppModule { }
3. Update the Main
In your main.ts
file, replace the platformBrowserDynamic().bootstrapModule()
method with platformBrowserDynamic().bootstrapModule(AppModule)
:
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './app/app.module';
platformBrowserDynamic().bootstrapModule(AppModule)
.catch(err => console.error(err));
4. Update the HTML
In the index.html
file, add the app-shell-fragment
component inside the app-root
tags:
<body>
<app-root>
<app-shell-fragment></app-shell-fragment>
</app-root>
</body>
5. Build and Serve Your Angular App on the Server
Finally, build and serve your Angular app on the server using the following command:
npm run build:ssr && npm run serve:ssr
Your Angular app is now rendering on the server, and you can access it through the provided server URL.
Benefits of Server-side Rendering for Performance and SEO
Implementing Angular Universal and server-side rendering in your Angular application brings several benefits for performance and SEO:
1. Improved Performance
With server-side rendering, your users will see the pre-rendered content while the JavaScript bundles are being downloaded and executed. This reduces the time-to-first-content and improves the perceived performance of your application.
2. Enhanced SEO
Search engines have a better ability to crawl and index your Angular app when it's pre-rendered on the server. SSR ensures that search engines can easily read and understand your content, leading to better search engine rankings and increased organic traffic.
By combining the benefits of performance and SEO, server-side rendering is a powerful technique to make your Angular application faster, more accessible, and more discoverable.
Conclusion
In this guide, we learned about Angular Universal and how it enables server-side rendering in Angular applications. We covered the steps to set up Angular Universal and implement server-side rendering, and we discussed the benefits of server-side rendering for performance and SEO.
By adopting server-side rendering with Angular Universal, you can significantly improve the performance and accessibility of your Angular applications, and ensure that they are optimized for search engine visibility.
Now it's time to take your Angular apps to the next level with server-side rendering!
Thank you for reading!