Applying Hexagonal Architecture with Domain-Driven Design in Golang Applications
Learn how to apply hexagonal architecture with domain-driven design in Golang applications. Build modular, testable, and maintainable applications with robust architecture.
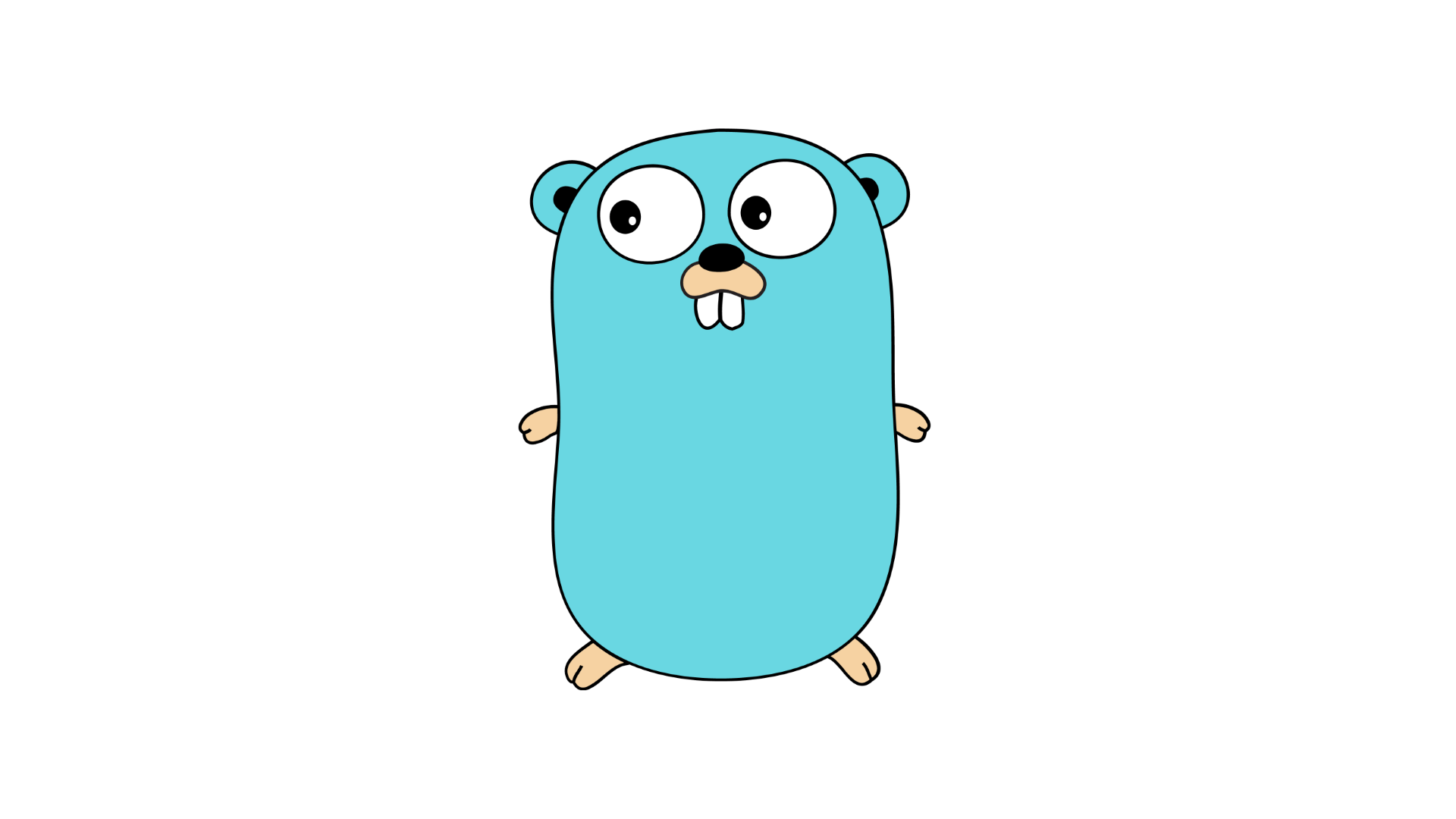
Introduction
When building complex applications, it's crucial to have a robust and scalable architecture in place. Hexagonal architecture, also known as Ports and Adapters architecture, provides an elegant solution to this problem. By applying the principles of hexagonal architecture alongside domain-driven design (DDD), you can create applications that are modular, testable, and maintainable.
In this article, we'll explore how to apply hexagonal architecture with domain-driven design in Golang applications. We'll discuss the key concepts and principles behind this architectural style and provide practical examples to help you implement it in your own projects. Let's get started!
What is Hexagonal Architecture?
Hexagonal architecture, introduced by Alistair Cockburn, is an architectural pattern that aims to separate the core business logic of the application from the surrounding infrastructure and technology concerns. This separation allows for better modularity and testability, as well as the ability to swap out and replace external dependencies without affecting the core functionality.
The key idea behind hexagonal architecture is to design the application as a series of hexagonal layers, with the core domain layer at the center. Each layer has its own responsibilities and dependencies, and they communicate with each other through ports and adapters.
Key Concepts of Hexagonal Architecture
1. Domain Layer
The domain layer is the heart of the application, where the core business logic resides. It consists of entities, value objects, and domain services that encapsulate the business rules and behavior of the application. The domain layer is technology-agnostic and does not depend on any external frameworks or libraries.
2. Ports and Adapters
Ports and adapters are the communication interfaces between the domain layer and the external world. Ports define the contract that the domain layer expects from the outside world, while adapters implement these contracts and connect the application to the infrastructure, such as databases, web frameworks, or external services.
There are two types of ports:
- Inbound ports: Also known as primary or driving adapters, these ports allow external systems to interact with the application. Inbound ports receive input from the outside world and pass it to the appropriate use cases in the domain layer.
- Outbound ports: Also known as secondary or driven adapters, these ports allow the application to interact with external systems. Outbound ports define the contract that the application expects from external dependencies, such as databases or third-party services.
3. Use Cases
Use cases represent the specific actions or operations that the application can perform. They encapsulate the business logic and orchestrate the collaboration between different entities and value objects in the domain layer. Use cases are triggered by the inbound ports and are responsible for validating inputs, executing the necessary business rules, and producing outputs.
Applying Hexagonal Architecture in Golang Applications
Golang, with its simplicity, performance, and strong ecosystem, is a great choice for building applications following hexagonal architecture principles. Let's walk through the steps to apply hexagonal architecture in Golang applications:
1. Create the Directory Structure
Start by creating the directory structure for your project:
myapp/
- cmd/
- api/
- cli/
- internal/
- app/
- usecase/
- domain/
- adapter/
- repository/
- http/
- grpc/
- pkg/
- mypkg/
- web/
- static/
- templates/
- docs/
- configs/
- tests/
This directory structure separates the different layers and components of your application, following the principles of hexagonal architecture.
2. Define the Domain Model
In the internal/app/domain
directory, define the entities, value objects, and domain services that represent the core business logic of your application. These should be independent of any external dependencies and frameworks.
internal/
- app/
- domain/
- entity/
- user.go
- order.go
- valueobject/
- money.go
- address.go
- service/
- payment.go
- shipping.go
3. Define the Use Cases
In the internal/app/usecase
directory, define the use cases that represent the specific actions or operations of your application. Each use case should have an interface that defines the contract and a corresponding implementation that contains the business logic.
internal/
- app/
- usecase/
- user/
- user.go
- user_service.go
- order/
- order.go
- order_service.go
4. Define the Ports and Adapters
In the internal/adapter
directory, define the ports and adapters that connect your application to the external world. Each adapter should implement the corresponding port interface defined in the domain or use case layer.
internal/
- adapter/
- repository/
- user_repository.go
- order_repository.go
- http/
- user_handler.go
- order_handler.go
- grpc/
- user_service.go
- order_service.go
5. Implement the Use Cases
In the use case implementation files (e.g., internal/app/usecase/user/user_service.go
), implement the business logic of each use case. Use the domain entities, value objects, and domain services to perform the necessary operations.
package user
import (
"github.com/myapp/internal/app/domain/entity"
"github.com/myapp/internal/app/domain/user"
)
type UserService struct {
userRepository user.Repository
}
func NewUserService(userRepository user.Repository) *UserService {
return &UserService{userRepository: userRepository}
}
func (s *UserService) CreateUser(name string, email string) (*entity.User, error) {
// Implement the business logic to create a new user
// Validate inputs, apply business rules, interact with repositories, etc.
// Use the user repository to save the new user
newUser := entity.NewUser(name, email)
err := s.userRepository.Save(newUser)
if err != nil {
return nil, err
}
return newUser, nil
}
// Other use case methods...
6. Wire Up the Dependencies
In the main file of your application (e.g., cmd/api/main.go
), wire up the dependencies by initializing the required ports and adapters and injecting them into the use case implementations.
package main
import (
"github.com/myapp/internal/adapter/http"
"github.com/myapp/internal/adapter/repository"
"github.com/myapp/internal/app/usecase/user"
)
func main() {
// Create the repository implementations
userRepository := repository.NewUserRepository()
// Create the use case implementations and inject the dependencies
userService := user.NewUserService(userRepository)
// Create the HTTP handler and wire up the routes
userHandler := http.NewUserHandler(userService)
userHandler.WireRoutes()
// Start the HTTP server
// ...
}
Benefits of Hexagonal Architecture
Applying hexagonal architecture with domain-driven design in Golang applications offers several benefits:
- Modularity and testability: The separation of concerns in hexagonal architecture makes it easier to test individual components in isolation and swap out dependencies for testing purposes, resulting in highly modular and testable code.
- Scalability and maintainability: The clear separation between the core domain logic and external dependencies makes it easier to scale and maintain the application over time. When changes are required, only the affected components need to be modified.
- Technology agnosticity: The core domain of the application remains independent of any specific technology or framework, allowing for easier migration or replacement of infrastructure components.
Conclusion
Applying hexagonal architecture with domain-driven design in Golang applications can significantly improve the quality, maintainability, and scalability of your codebase. By separating concerns, defining clear interfaces, and following the principles of hexagonal architecture, you can build applications that are modular, testable, and easy to maintain.
Remember, hexagonal architecture is not limited to Golang—it can be applied to any programming language or technology stack. So, whether you're working on a small project or a large-scale application, consider incorporating hexagonal architecture principles into your design for better long-term success.
Happy coding!