gRPC Streaming: Building Robust and Efficient Data Streaming Applications
Learn about gRPC streaming, a powerful feature of gRPC that enables real-time bidirectional communication. Discover the types of streaming, build a chat app, and optimize streaming performance.
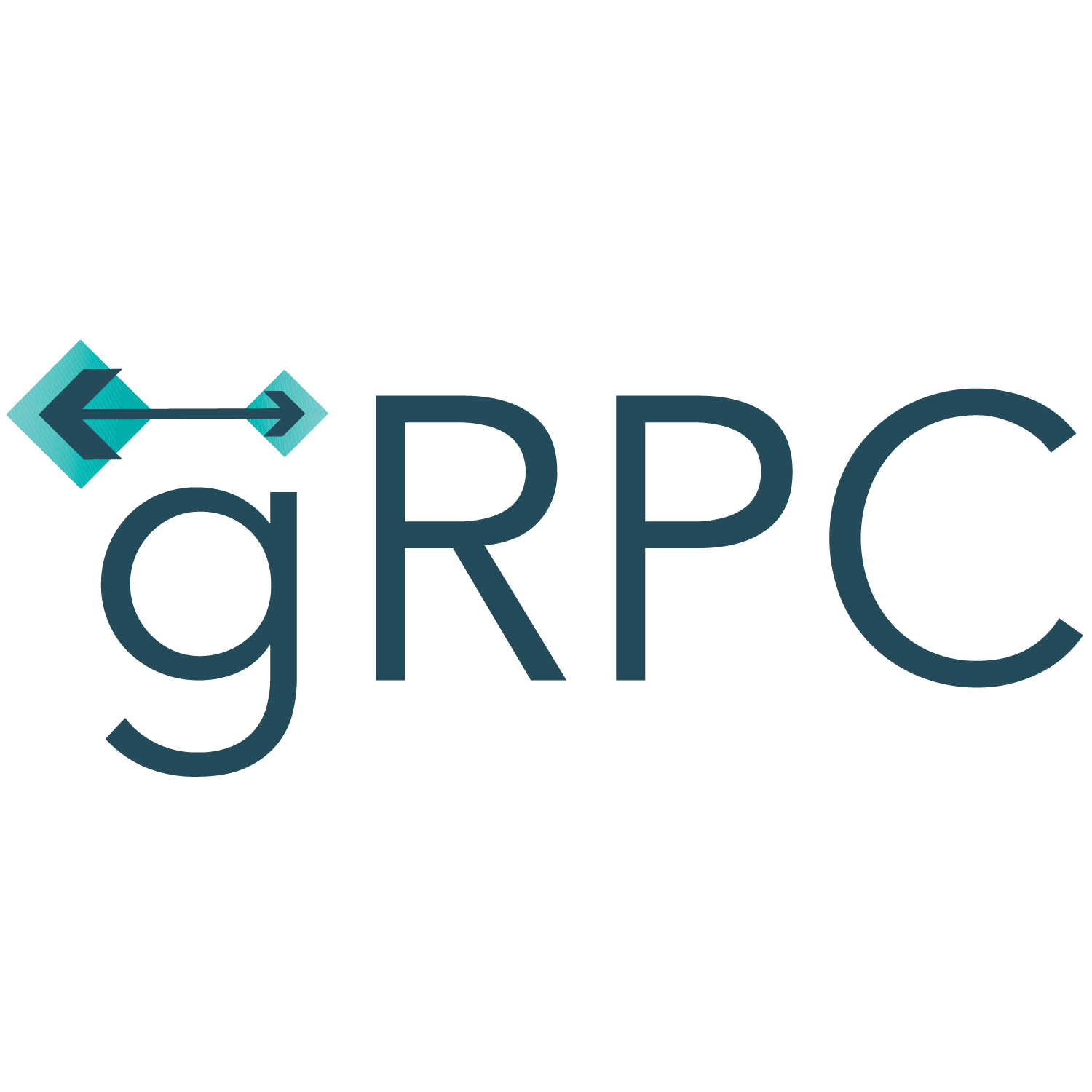
Introduction
Streaming data is a critical aspect of many modern applications, enabling real-time communication, data processing, and analytics. Traditional request-response models may not always be suitable for scenarios that involve continuous data transmission or bidirectional communication. This is where gRPC streaming comes to the rescue.
In this blog post, we will explore gRPC streaming and its significance in building robust and efficient data streaming applications. We'll dive into the different types of gRPC streaming, demonstrate practical examples, and provide tips for optimizing streaming performance. Let's get started!
What is gRPC Streaming?
gRPC is an open-source remote procedure call (RPC) framework developed by Google. It allows developers to build high-performance and scalable distributed systems effortlessly. One of the key features of gRPC is its support for streaming, which enables the efficient transfer of large volumes of data.
With gRPC streaming, you can establish long-lived bidirectional communication channels between a client and a server. This means that both the client and the server can send and receive multiple messages over the same connection, eliminating the need for repeated requests and reducing network overhead.
There are two types of gRPC streaming:
1. Unary RPC
In Unary RPC, the client sends a single request to the server and receives a single response in return. This is the conventional request-response mechanism.
2. Server Streaming RPC
In Server Streaming RPC, the client sends a single request to the server, but the server responds with a stream of messages. The client reads the messages sequentially as they arrive.
3. Bidirectional Streaming RPC
In Bidirectional Streaming RPC, both the client and the server can send and receive a stream of messages independently. This allows for real-time bidirectional communication between the client and the server.
Building a gRPC Streaming Application
To demonstrate the power of gRPC streaming, let's build a simple real-time chat application. We will use Node.js for the server-side implementation and JavaScript for the client-side implementation.
1. Set up the Environment
Before we start, ensure that you have Node.js and npm (Node Package Manager) installed on your machine. You can download them from the official Node.js website, nodejs.org.
2. Create a new directory and initialize a new npm project
Open your terminal and navigate to the desired directory where you want to create your project. Run the following command to create a new npm project:
mkdir grpc-chat-app
cd grpc-chat-app
npm init -y
3. Install Required Dependencies
To set up the server-side implementation, we need to install the following dependencies:
npm install grpc @grpc/proto-loader
For the client-side implementation, we need the following dependencies:
npm install grpc-web
4. Define the Protobuf Service
gRPC uses Protocol Buffers (protobuf) to define the service and the data structures. Create a new file called chat.proto
in the project root directory and add the following code:
syntax = "proto3";
package chat;
message Message {
string body = 1;
}
service Chat {
rpc SendMessage(Message) returns (stream Message);
rpc ReceiveMessage(stream Message) returns (stream Message);
}
5. Implement the Server
Create a new file called server.js
and add the following code:
// Import the required modules
const grpc = require('grpc');
const protoLoader = require('@grpc/proto-loader');
// Define the server implementation
const server = new grpc.Server();
// Load the protobuf definition
const PROTO_PATH = __dirname + '/chat.proto';
const packageDefinition = protoLoader.loadSync(PROTO_PATH);
const chat = grpc.loadPackageDefinition(packageDefinition).chat;
// Start the gRPC server
server.bind('0.0.0.0:50051', grpc.ServerCredentials.createInsecure());
server.start();
console.log('gRPC server started on port 50051');
6. Implement the Client
Create a new file called client.js
and add the following code:
// Import the required modules
const grpc = require('grpc');
const grpcWeb = require('grpc-web');
// Load the protobuf definition
const PROTO_PATH = 'chat.proto';
const ChatService = grpc.load(PROTO_PATH).chat.Chat;
// Create a gRPC-Web client
const client = new ChatService('http://localhost:8080', null, null);
// Initialize the chat
const chat = document.getElementById('chat');
const input = document.getElementById('input');
const form = document.getElementById('form');
// Send message to the server
form.addEventListener('submit', (e) => {
e.preventDefault();
const message = {
body: input.value,
};
client.sendMessage(message, {}, (err, response) => {
if (err) {
console.error(err);
} else {
console.log('Message sent successfully:', response);
}
input.value = '';
});
});
// Receive messages from the server
const stream = client.receiveMessage({});
stream.on('data', (message) => {
console.log('Received message from server:', message);
const listItem = document.createElement('li');
listItem.innerText = message.body;
chat.appendChild(listItem);
});
7. Start the Server and Run the Client
Open two terminals. In one terminal, run the following command to start the gRPC server:
node server.js
In the other terminal, run the following command to start the gRPC client:
npm run start
Open your browser and navigate to http://localhost:8080
. You should see a chat interface. Enter a message in the input field and press enter. The message will be sent to the server and displayed in the chat window. You can open multiple browser windows to simulate multiple clients.
Optimizing gRPC Streaming Performance
When building data streaming applications using gRPC, it's essential to optimize the performance for efficient data transmission. Here are some tips to enhance gRPC streaming performance:
1. Use Efficient Serialization
Choose a serialization format that suits your data streaming needs. Protocol Buffers (protobuf) is the recommended serialization format for gRPC, as it offers efficient data encoding and decoding, resulting in reduced payload size and improved performance.
2. Stream Batching
If your application involves sending a large number of small messages, consider grouping them into batches before sending them over the network. This reduces the overhead of individual message headers, resulting in improved network efficiency.
3. Compression
Understand the nature of your data and consider compressing it if it's suitable for your use case. Compression significantly reduces the data size and improves network performance. gRPC supports various compression algorithms, such as gzip and deflate.
4. Concurrency Control
Optimize the concurrency settings of your gRPC server to handle streaming requests efficiently. Use parallel processing techniques and consider utilizing gRPC's built-in flow control mechanisms, such as flow windowing, to manage the rate of data transfer.
Conclusion
gRPC streaming provides a powerful mechanism for building robust and efficient data streaming applications. Whether you need to establish bidirectional communication channels, perform real-time analytics, or process continuous data streams, gRPC streaming can cater to your requirements.
In this blog post, we explored the different types of gRPC streaming, built a real-time chat application using gRPC, and discussed tips for optimizing streaming performance. Armed with this knowledge, you can leverage gRPC streaming to create seamless data streaming solutions.
Happy streaming!