gRPC Performance Tuning: Tips and Techniques for Maximum Efficiency
Optimize the performance of your gRPC applications with these tips: efficient data serialization, protobuf compiler plugin, resource usage, streaming, compression, network settings, benchmarking. Boost your microservices' efficiency!
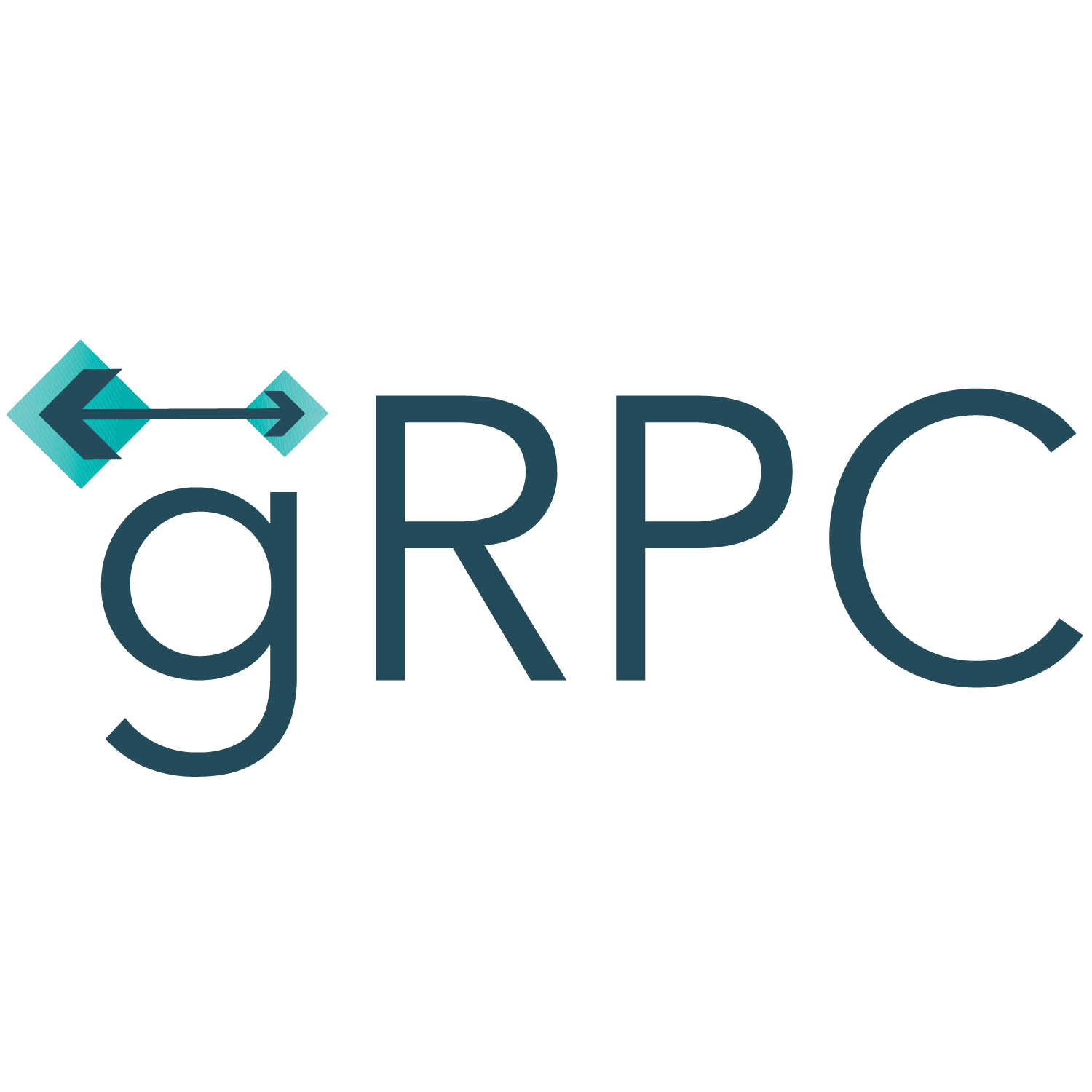
Introduction
gRPC, an open-source remote procedure call (RPC) framework, has gained significant popularity for its efficient and high-performance communication between microservices. However, to truly harness the power of gRPC, it's crucial to optimize its performance.
In this blog post, we'll explore tips and techniques for tuning the performance of gRPC applications. By understanding these optimization strategies, you'll be able to squeeze the maximum efficiency out of your gRPC-based microservices, resulting in better application performance and scalability.
1. Use Protocol Buffers for Efficient Data Serialization
Protocol Buffers (protobuf) is the default data serialization format used by gRPC. It enables efficient binary encoding of structured data, resulting in faster data transmission and reduced network overhead.
To maximize performance, it's essential to design your protobuf messages with efficiency in mind:
- Avoid including unnecessary data fields in your protobuf messages.
- Prefer using scalar types (such as int32, float, bool) over repeated or nested message fields when possible.
- Keep your message structures flat instead of deeply nested to improve serialization and deserialization performance.
2. Consider Using the Protobuf Compiler Plugin
The gRPC ecosystem provides a protobuf compiler plugin called "protoc-gen-go-grpc" that generates optimized gRPC code for Go applications. This plugin generates code that utilizes gRPC's internal APIs, resulting in better performance compared to the default code generated by "protoc-gen-go".
To use the "protoc-gen-go-grpc" plugin, you need to install it first:
```bash $ go install google.golang.org/grpc/cmd/protoc-gen-go-grpc ```
Once installed, update your protobuf file to use the plugin:
syntax = "proto3";
import "google/protobuf/empty.proto";
option go_package = "your/package";
service YourService {
rpc YourMethod(Empty) returns (Empty) {
option (google.api.http) = {
post: "/your-method"
body: "*"
};
}
}
```
Then, generate the gRPC code using the plugin:
```bash
$ protoc --go_out=. --go-grpc_out=. path/to/yourpb.proto
```
3. Optimize Server Resource Usage
To maximize gRPC server performance, it's crucial to optimize the usage of server resources:
Use connection pooling techniques, such as gRPC's connection keep-alive mechanism, to reuse connections and minimize connection setup overhead.
Configure gRPC server options, such as maximum concurrent connections and maximum message sizes, based on your application's requirements.
Implement proper error handling and graceful shutdown to prevent resource leaks and ensure efficient resource utilization.
4. Utilize Client Streaming and Server Streaming
gRPC supports client streaming and server streaming, which can significantly improve performance in certain scenarios:
Client Streaming: Use client streaming when the client needs to send a large amount of data to the server. Instead of sending multiple individual requests, the client can stream the data to the server, reducing the overhead of establishing multiple connections.
Server Streaming: Use server streaming when the server needs to send a large response to the client. Instead of sending the entire response in one go, the server can stream the response in chunks, allowing the client to process the data incrementally.
5. Enable Gzip Compression
Enabling gzip compression for gRPC payloads can significantly reduce the data size and improve network throughput. By compressing the data before transmission, you can achieve faster data transfer and lower network latency.
To enable gzip compression in gRPC, you can use interceptors or middleware, depending on your programming language and framework. These techniques allow you to dynamically compress and decompress gRPC messages on the fly.
6. Optimize Network Settings
To achieve optimal gRPC performance, consider fine-tuning your network settings:
Enable TCP window scaling to improve network transfer speeds.
Adjust TCP buffer sizes to accommodate your application's data transfer requirements.
Monitor and optimize network latency to minimize round-trip times between gRPC clients and servers.
7. Benchmark and Profile
Benchmarking and profiling your gRPC applications is essential to identify performance bottlenecks and optimize them. By measuring key performance metrics, such as latency, throughput, and resource utilization, you gain insights into your application's behavior under different load conditions.
Utilize gRPC-specific benchmarking tools, such as grpc_bench, ghz, or your preferred load testing tool, to stress test your gRPC services, simulate real-world scenarios, and identify potential performance issues.
Wrapping Up
By applying the tips and techniques outlined in this blog post, you can optimize the performance of your gRPC applications and unlock their full potential. Remember to benchmark and monitor your application's performance regularly to ensure continuous optimization as your application evolves.
So go ahead, apply these performance tuning strategies, and build highly efficient gRPC microservices!