Zero Allocation in Golang: An Efficient Memory Management Technique
"Zero Allocation is a technique used in Golang to minimize memory allocation on the heap. By reusing memory, it improves performance and reduces memory footprint, resulting in better scalability and efficiency."
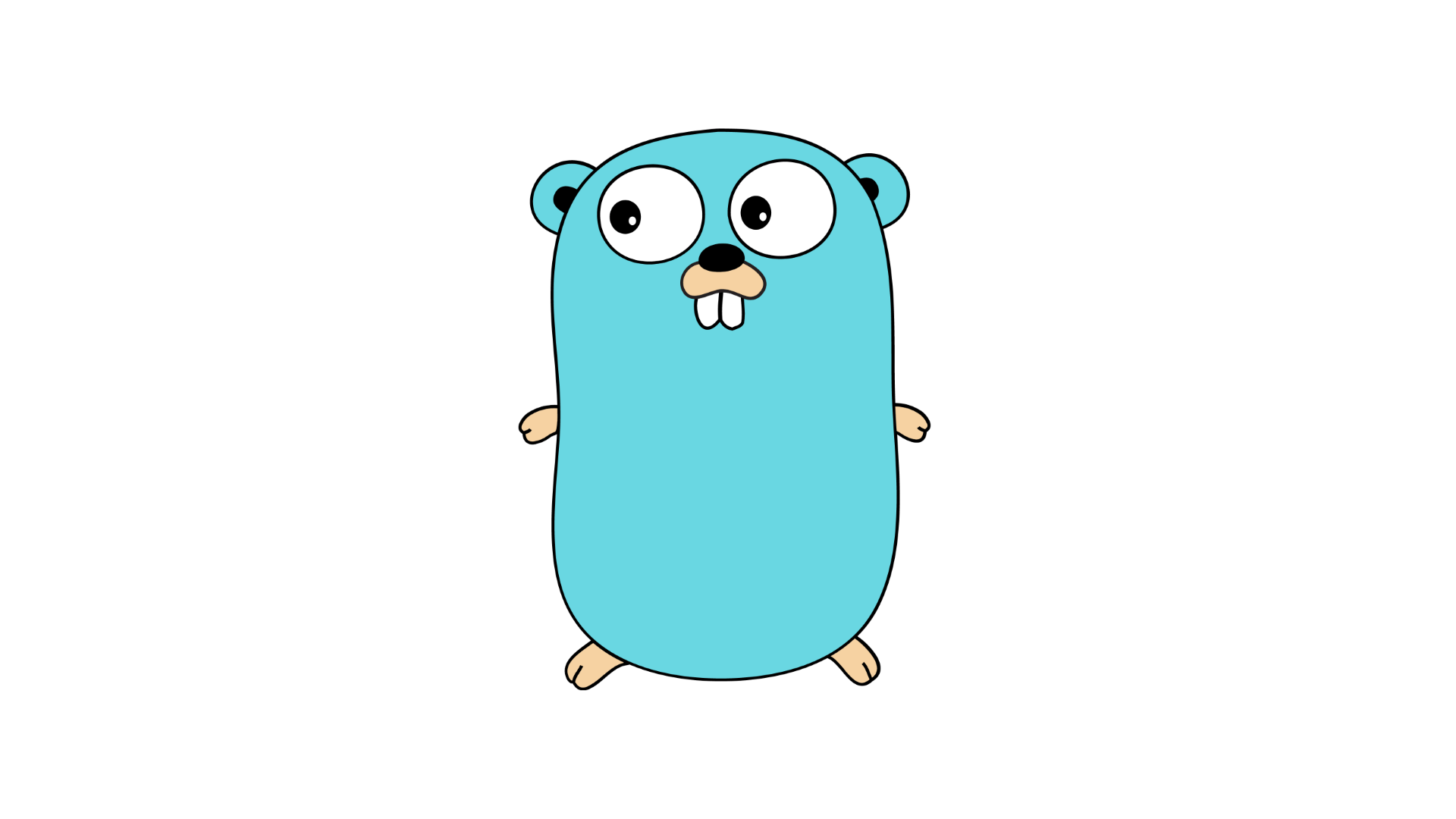
Introduction
Golang, also known as Go, is a powerful programming language that prioritizes efficiency and simplicity. One key aspect of efficiency is memory management. In this blog post, we will explore "Zero Allocation," a memory management technique used in Golang that helps eliminate unnecessary allocations and reduces the overall memory footprint of your programs.
What is Zero Allocation?
Zero Allocation is a technique used in Golang to minimize the allocation of memory on the heap, ultimately resulting in more efficient memory usage. In Golang, memory can be allocated on the stack or the heap. Allocations on the heap require more overhead than stack allocations, such as garbage collection and longer access times.
The idea behind Zero Allocation is to reuse memory whenever possible instead of allocating new memory on the heap. By reusing memory, we can significantly reduce the number of allocations and deallocations, resulting in improved performance and reduced memory pressure on the garbage collector.
Benefits of Zero Allocation
Using Zero Allocation techniques can provide several benefits:
- Improved Performance: By reducing the number of heap allocations, the overall performance of your Golang program can be improved. Fewer allocations mean less time spent on garbage collection and less memory fragmentation.
- Reduced Memory Footprint: With Zero Allocation, you can minimize the amount of memory your program consumes. This is especially beneficial in resource-constrained environments or when working with large-scale applications.
- Better Scalability: Zero Allocation allows your program to handle more requests or process more data without overwhelming the garbage collector. This can result in improved scalability and responsiveness.
Techniques for Zero Allocation
1. Using sync.Pool
The sync.Pool
package provides a built-in mechanism for object pooling in Golang. It allows you to reuse allocated memory by providing a pool of objects that can be borrowed and returned. The pool internally manages the allocation and reuse of objects, reducing the need for frequent heap allocations.
Here's an example of using sync.Pool
:
import (
"sync"
)
type MyObject struct {
// Define your object fields here
}
var myObjectPool = sync.Pool{
New: func() interface{} {
return &MyObject{}
},
}
func GetMyObject() *amp;MyObject {
return myObjectPool.Get().(*MyObject)
}
func ReleaseMyObject(obj *amp;MyObject) {
myObjectPool.Put(obj)
}
In the above code, we define an object pool using the sync.Pool
package. The New
field of the pool specifies a function to create a new object if the pool is empty. The GetMyObject
function retrieves an object from the pool, and the ReleaseMyObject
function returns the object to the pool for future reuse.
2. Using Pool of Buffers for String Operations
In Golang, string concatenation can result in a new string being allocated on each concatenation operation. To eliminate these allocations, you can use a pool of buffers to perform string operations. By reusing buffers, you can avoid the overhead of memory allocation each time you concatenate strings.
Here's an example of using a pool of buffers for string operations:
import (
"bytes"
"sync"
)
var bufferPool = sync.Pool{
New: func() interface{} {
return bytes.NewBuffer(make([]byte, 0, 1024))
},
}
func ConcatStrings(strings []string) string {
buffer := bufferPool.Get().(*bytes.Buffer)
defer bufferPool.Put(buffer)
buffer.Reset()
for _, str := range strings {
buffer.WriteString(str)
}
return buffer.String()
}
In the above code, we define a pool of buffers using sync.Pool
. The New
field of the pool is set to a function that creates a new bytes.Buffer
with an initial capacity of 1024 bytes. The ConcatStrings
function borrows a buffer from the pool, resets it, and performs string concatenation on the borrowed buffer. Finally, the function returns the concatenated string.
Best Practices for Zero Allocation
1. Profile and Benchmark Your Code
Before applying any optimization techniques, it is essential to profile and benchmark your code to identify performance bottlenecks. Tools like go test -bench
and go test -benchmem
can help you measure the performance and memory usage of your Golang programs. By understanding the areas that can be optimized, you can apply Zero Allocation techniques more effectively.
2. Reuse Objects Wherever Possible
Instead of constantly creating new objects, try to reuse existing objects. For example, if you have a struct or array that no longer serves its initial purpose, consider repurposing it instead of allocating a new one. Reusing objects not only reduces allocations but also improves cache locality and reduces garbage collection pressure.
3. Avoid Unnecessary Conversions and Copies
Each conversion or copy operation in Golang can result in memory allocations. If possible, avoid unnecessary conversions between types or copying of data. Instead, consider using pointers or references to share data between functions or goroutines, minimizing memory allocations.
4. Limit the Use of Reflection
Reflection in Golang allows you to inspect and manipulate variables at runtime, but it comes with a performance cost. The reflect
package often involves heap allocations and type conversions. While reflection can be powerful, it should be used judiciously to minimize unnecessary memory allocations.
Conclusion
Zero Allocation is an essential technique for efficient memory management in Golang. By minimizing heap allocations and reusing memory wherever possible, you can significantly improve the performance and memory footprint of your programs. Remember to profile and benchmark your code to identify areas that can benefit from Zero Allocation techniques, and apply best practices to leverage the full power of Golang's memory management capabilities.
Happy coding!