Explaining Zero Allocation in Golang: Technical Insights and Examples
Zero allocation is crucial for performance optimization in Go programming. By minimizing memory allocation, you can improve efficiency, reduce garbage collection pressure, and achieve faster execution. Learn important techniques and see their impact through benchmarking.
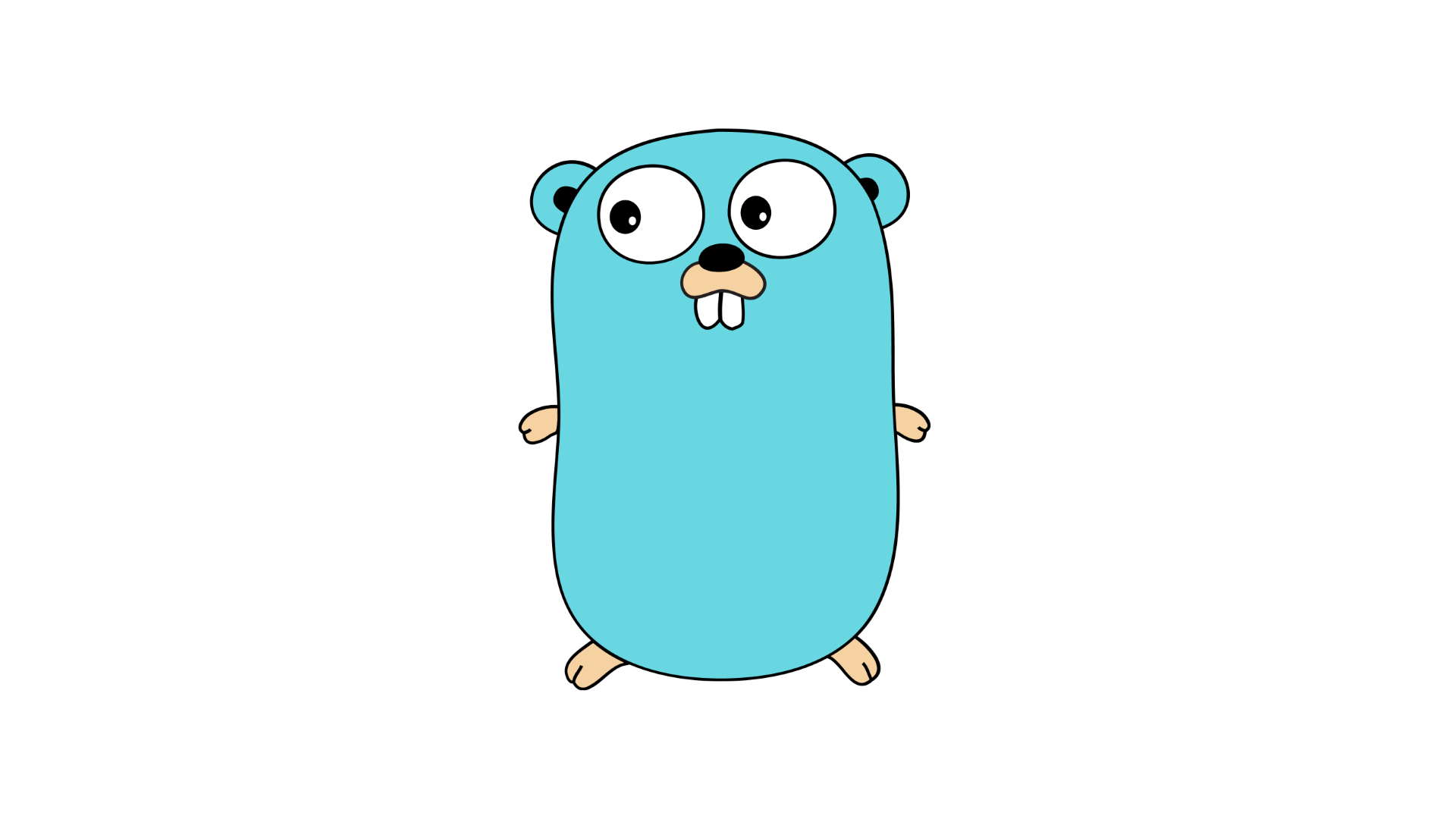
Introduction
When it comes to performance optimization in Go programming, understanding and minimizing memory allocation is crucial. One of the key concepts for achieving this is zero allocation, which refers to the avoidance of unnecessary memory allocation during program execution. In this blog post, we'll dive into the technical details of zero allocation in Golang and explore some examples to showcase its significance and benefits.
What is Zero Allocation?
In Go, zero allocation refers to the practice of minimizing or completely eliminating the creation of new memory allocations (Heap Objects) during the execution of a program. This is accomplished by utilizing zero value initialization and by leveraging stack allocation instead of heap allocation for variables whenever possible. By reducing allocations, you can optimize your code's memory usage and improve overall performance.
Importance of Zero Allocation
Minimizing memory allocation is important for a number of reasons:
- Efficient memory usage: By avoiding unnecessary allocations, you can keep your memory footprint small, which can be critical when dealing with resource-constrained systems and applications.
- Reduced garbage collection (GC) pressure: Garbage collection is a process that automatically reclaims memory that is no longer in use. By minimizing allocations, you decrease the frequency and impact of garbage collection, resulting in better performance and lower CPU utilization.
- Faster execution: Allocating memory on the heap can be a relatively expensive operation, especially when compared to stack allocations. By minimizing heap allocations, you can reduce the time spent on memory management, leading to faster program execution.
Techniques for Achieving Zero Allocation
Now that we understand the importance of zero allocation, let's explore some techniques that can help us achieve it:
1. Use Zero Value Initialization
Go initializes variables with their zero values by default. This means that you don't need to explicitly initialize variables to zero or any other value. By utilizing zero value initialization, you can avoid unnecessary memory allocations.
Consider the following example:
var s []int // The variable 's' is already initialized with a zero value of 'nil'
var x int // The variable 'x' is initialized with a zero value of '0'
It's important to note that stack-allocated variables already have zero values, so initializing them to zero is redundant and adds unnecessary complexity to your code.
2. Use Stack-Allocated Variables
Variables allocated on the stack have significantly lower overhead compared to heap-allocated variables. In many cases, it's possible to leverage stack allocation instead of heap allocation.
Consider the following example:
func processString(s string) {
// ...
}
func main() {
str := "Hello, world!" // The variable 'str' is stack-allocated
processString(str)
// ...
}
In this example, the string variable 'str' is stack-allocated within the 'main' function, and it's then passed to the 'processString' function by value. Since the string is not modified in the 'processString' function, there is no need for heap allocation. This not only reduces memory usage but also improves performance.
3. Use Slice Tricks
In Go, slices are typically backed by arrays, and creating a new slice often results in an allocation. However, there are some slice tricks that can help you avoid these allocations.
One common technique is to use the 'append' function with an existing slice and a length argument equal to the current length of the slice, forcing the append function to reuse the existing array if it has enough capacity.
Consider the following example:
existingSlice := []int{1, 2, 3, 4, 5}
newSlice := append(existingSlice[:len(existingSlice)], 6)
In this example, we have an existing slice 'existingSlice' with some elements. By using a slice expression with a length argument equal to the current length of 'existingSlice', we force the 'append' function to reuse the underlying array. This avoids unnecessary memory allocation.
Benchmarking Zero Allocation
Now that we understand the techniques for achieving zero allocation, let's explore their impact on performance by benchmarking some examples. Benchmarking allows us to compare the performance of different approaches and measure the speed and memory consumption of our code.
We'll use Go's built-in benchmarking functionality along with the 'testing' package to measure the performance of our code. Below is an example:
import (
"testing"
)
const (
benchmarkSize = 1000000
)
func BenchmarkZeroAllocation(b *testing.B) {
for i := 0; i < b.N; i++ {
// Perform operations that minimize or avoid allocations
}
}
func BenchmarkWithAllocation(b *testing.B) {
for i := 0; i < b.N; i++ {
// Perform operations that result in allocations
}
}
In this example, we define two benchmark functions: 'BenchmarkZeroAllocation' and 'BenchmarkWithAllocation'. We can then use the 'go test' command with the '-bench' flag to run the benchmarks and compare their performance.
Conclusion
Zero allocation is a powerful technique for optimizing performance and memory usage in Go programs. By understanding the importance of zero allocation and implementing the techniques we've explored, you can significantly improve the efficiency and speed of your code.
Remember, achieving zero allocation requires careful consideration and attention to detail. Benchmarks can help you identify performance bottlenecks and measure the impact of your optimizations.
By applying zero allocation principles and leveraging the performance tools provided by Go, you'll be well-equipped to build high-performance and memory-efficient applications.