Golang Unit Testing: Frameworks and Strategies
Unit testing is critical for code quality and reliability. Go provides its own testing package, but third-party frameworks like testify and goblin enhance its capabilities. Effective strategies like table-driven tests and test coverage analysis further improve testing.
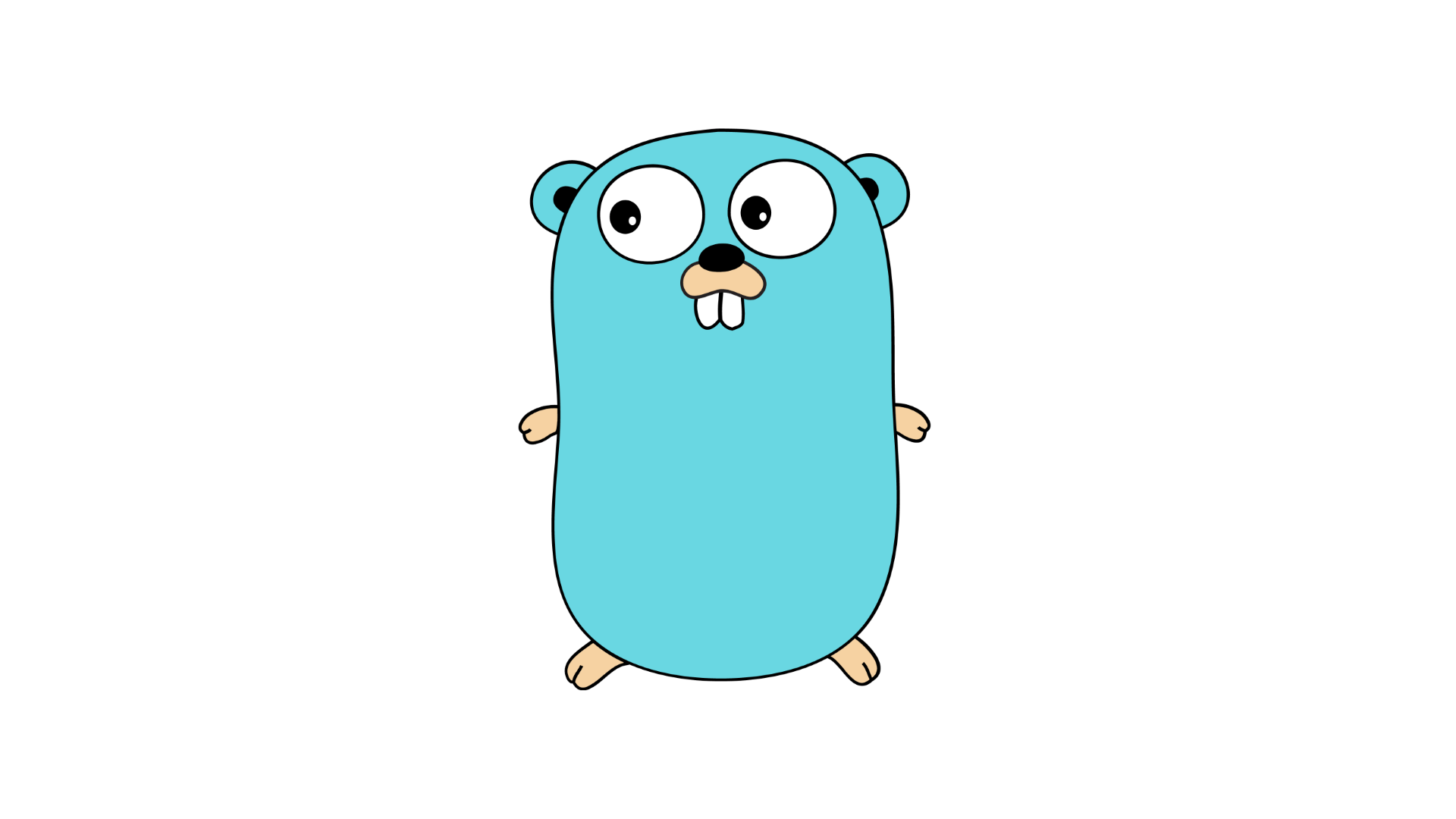
Golang Unit Testing: Frameworks and Strategies
Unit testing is a critical aspect of software development, as it helps ensure the quality and reliability of your code. Go (Golang) has built-in support for unit testing, making it easy to write tests for your applications. In this blog post, we'll explore the various frameworks and strategies available for unit testing in Go.
Why Unit Testing is Important
Unit testing involves testing individual units of code, such as functions, methods, or classes, in isolation to verify their correctness. It offers several advantages:
- Identify Bugs Early: Unit tests can catch bugs at the earliest stages of development, allowing you to fix them before they propagate to other parts of your codebase.
- Ensure Code Coverage: By writing comprehensive tests for your code, you can ensure that all aspects of your application are thoroughly tested.
- Regression Testing: Unit tests act as a safety net while refactoring or making changes to your code. They help catch any regressions or unintended side effects.
- Improved Design: Writing testable code often leads to better overall design and modularity.
Unit Testing Frameworks
Go provides a robust testing framework in its standard library called testing
. However, there are several third-party libraries and frameworks available that extend and enhance the Go testing capabilities. Let's take a look at some popular ones:
1. Testing Package
The testing
package included in the Go standard library is a lightweight and easy-to-use testing framework. It follows a simple convention for organizing tests. Here's an example:
package mypackage
import (
"testing"
)
func TestAdd(t *testing.T) {
result := Add(2, 3)
if result != 5 {
t.Errorf("Add(2, 3) = %d; want 5", result)
}
}
Running tests with the standard testing package is as simple as executing the go test
command in your test package directory.
While the standard package provides basic testing capabilities, there are some limitations. You may find yourself needing additional functionality or convenience offered by third-party testing frameworks.
2. testify
testify is a popular third-party testing framework that provides additional assertion and mocking capabilities. It enhances the standard testing package and simplifies common testing tasks. You can install it using the following command:
go get github.com/stretchr/testify
Here's an example of how to use testify:
package mypackage
import (
"testing"
"github.com/stretchr/testify/assert"
)
func TestAdd(t *testing.T) {
result := Add(2, 3)
assert.Equal(t, 5, result, "Add(2, 3) should equal 5")
}
With testify, you gain access to a variety of helpful assertions, such as Equal
, True
, False
, Nil
, NotNil
, and many more. These assertions make your tests more expressive and readable.
In addition, testify provides mocking capabilities through its Mock
package, allowing you to create mock objects for easier testing of complex dependencies.
3. goblin
goblin is another popular testing framework for Go, known for its BDD (Behavior-Driven Development) style syntax. It offers a clean and fluent way to write tests.
You can install goblin using the following command:
go get github.com/franela/goblin
Here's an example of how to use goblin:
package mypackage
import (
"testing"
. "github.com/franela/goblin"
)
func TestAdd(t *testing.T) {
g := Goblin(t)
g.Describe("Add", func() {
g.It("should return the sum of two numbers", func() {
result := Add(2, 3)
g.Assert(result).Equal(5)
})
})
}
goblin provides a descriptive and concise syntax for defining test cases, making it easy to understand what each test is validating.
It also offers intuitive assertions through the Assert
method, making your tests more readable and self-explanatory.
Testing Strategies
While choosing a testing framework is essential, employing effective testing strategies is equally important. Here are a few strategies to consider when writing unit tests in Go:
1. Table-Driven Tests
Table-driven tests involve defining a set of input parameters and expected output values in a data table. These tests allow you to test multiple scenarios with minimal code duplication. Here's an example:
package mypackage
import (
"testing"
)
func TestAdd(t *testing.T) {
testCases := []struct {
name string
a, b int
expected int
}{
{"Test Case 1", 2, 3, 5},
{"Test Case 2", -2, 3, 1},
{"Test Case 3", 0, 0, 0},
}
for _, tc := range testCases {
t.Run(tc.name, func(t *testing.T) {
result := Add(tc.a, tc.b)
if result != tc.expected {
t.Errorf("Add(%d, %d) = %d; want %d", tc.a, tc.b, result, tc.expected)
}
})
}
}
Table-driven tests make it easy to extend and maintain your tests as new scenarios arise.
2. Test Coverage
Test coverage is the measure of which parts of your code are exercised by your unit tests. Ensuring a high level of test coverage is crucial to identify untested code and ensure proper validation. Go provides a built-in tool called go test --cover
to calculate the test coverage.
As a best practice, aim for high test coverage to minimize the risk of undiscovered bugs and to improve overall code quality.
3. Test Naming Conventions
Following consistent and meaningful naming conventions for your tests is essential. Use descriptive names that clearly convey the purpose of each test case. This makes it easier to understand the purpose of the test and to identify which parts of your code are covered by the tests.
For example, prefixing your test functions with the word "Test" and using camel-cased names helps to identify tests easily and follow common testing conventions.
Conclusion
Unit testing plays a vital role in maintaining the quality and reliability of software applications. In Go, you have several options for testing frameworks, including the built-in testing
package, as well as third-party frameworks like testify and goblin. These frameworks provide additional capabilities and improved syntax for writing tests.
Along with choosing the right framework, adopting effective testing strategies such as table-driven tests, test coverage analysis, and meaningful test naming conventions helps improve the quality of your unit tests.
By incorporating these best practices and strategies into your testing workflow, you can confidently write robust and reliable code in Go. Happy testing!