Golang for Web Scraping: Tools and Strategies
Golang is a powerful and efficient language for web scraping. With tools like Colly, GoQuery, and goroutines, you can easily build robust and concurrent scraping code. Remember to respect robots.txt and handle rate limits for a smooth scraping experience.
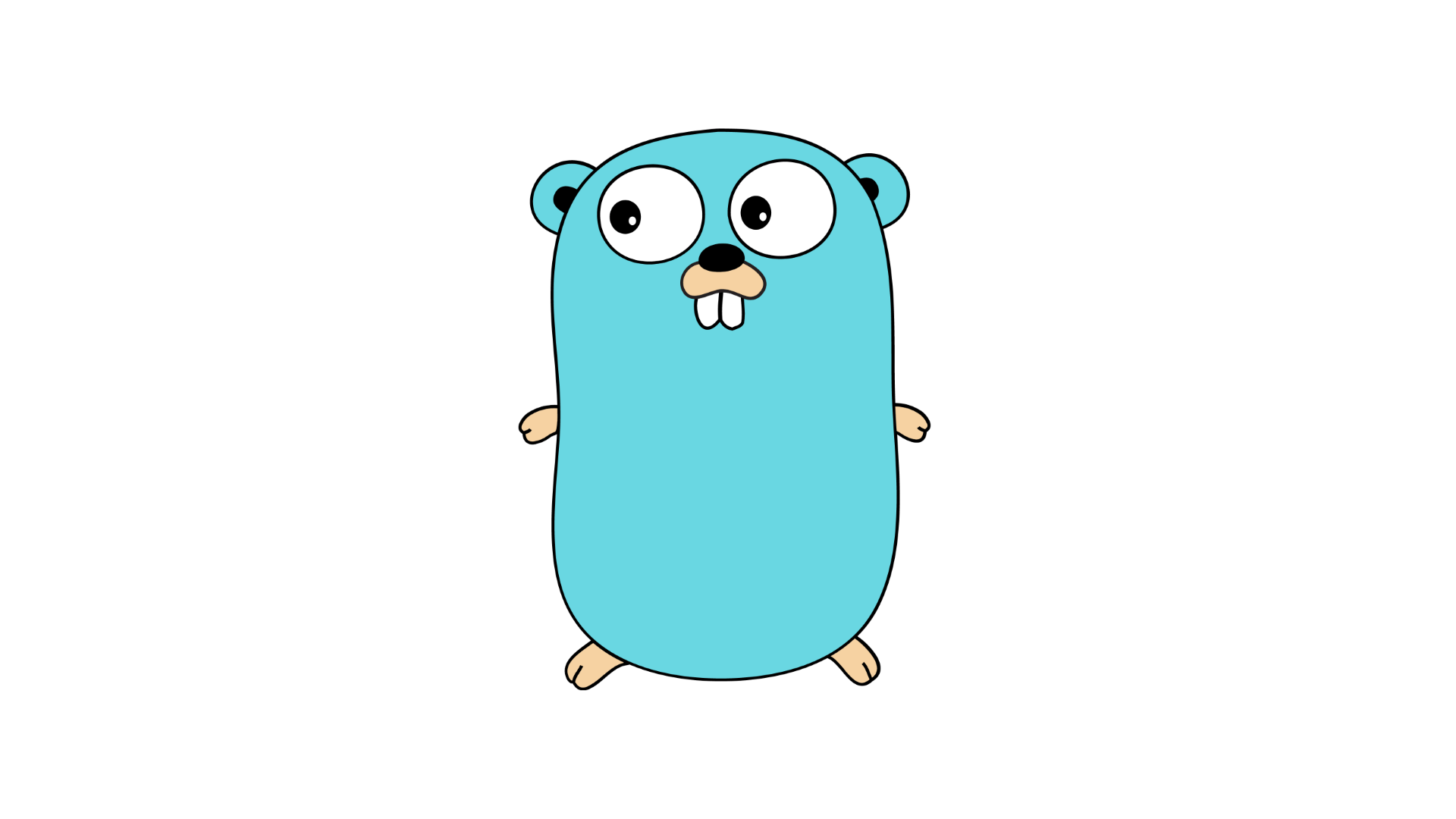
Introduction
Web scraping is a powerful technique for extracting data from websites. It allows you to automate the process of gathering valuable information, such as prices, reviews, and product details, from multiple websites. Go (Golang) is an efficient and versatile programming language that provides excellent tools and strategies for web scraping. In this blog post, we'll explore some of the best Golang tools and strategies for web scraping.
Why Use Golang for Web Scraping
Golang is a statically-typed, compiled language known for its simplicity and performance. It offers several advantages that make it an excellent choice for web scraping:
- Concurrency: Golang's built-in concurrency features, such as goroutines and channels, make it easy to write highly parallelized and efficient scraping code.
- Speed: Golang is known for its fast execution speed, allowing you to scrape websites quickly and efficiently.
- Robustness: Golang's strong typing and compiler-enforced error checking ensure that your scraping code is reliable and free from common runtime errors.
- Ecosystem: Golang has a rich ecosystem with many libraries and tools specifically designed for web scraping.
Golang Tools for Web Scraping
1. Colly
Colly is a popular Golang web scraping framework that provides a clean and simple API for building web crawlers. It supports features like automatic cookie and session handling, asynchronous requests, and customizable scraping rules. Let's see a simple example of how to use Colly:
package main
import (
"fmt"
"log"
"github.com/gocolly/colly/v2"
)
func main() {
c := colly.NewCollector()
c.OnHTML("h1", func(e *colly.HTMLElement) {
fmt.Println(e.Text)
})
err := c.Visit("https://example.com")
if err != nil {
log.Fatal(err)
}
}
In the above code, we create a new Colly collector, define a callback function to be executed when an HTML element with the h1
tag is found, and visit a website. When the specified HTML element is found, its text content is printed. Colly handles the HTTP requests and threading for us.
2. GoQuery
GoQuery is a powerful library that brings a jQuery-like syntax to Golang, making it easier to traverse and manipulate HTML documents. It provides methods for searching, filtering, and manipulating HTML elements. Here's an example of how to use GoQuery:
package main
import (
"fmt"
"log"
"github.com/PuerkitoBio/goquery"
)
func main() {
doc, err := goquery.NewDocument("https://example.com")
if err != nil {
log.Fatal(err)
}
doc.Find("h1").Each(func(i int, s *goquery.Selection) {
fmt.Println(s.Text())
})
}
In the above code, we create a new GoQuery document by providing a URL. We then use the Find
method to select all HTML elements with the h1
tag and print their text content. GoQuery's intuitive syntax simplifies the traversal and manipulation of HTML documents.
3. Goroutines and Channels
Goroutines and channels are powerful concurrency features in Golang that can greatly enhance your web scraping code. Goroutines allow you to perform multiple tasks concurrently, while channels enable safe communication and synchronization between goroutines. Here's an example that demonstrates the use of goroutines and channels for concurrent web scraping:
package main
import (
"fmt"
"log"
"net/http"
"github.com/PuerkitoBio/goquery"
)
func scrapeURL(url string, c chan<string) {
resp, err := http.Get(url)
if err != nil {
log.Fatal(err)
}
defer resp.Body.Close()
doc, err := goquery.NewDocumentFromReader(resp.Body)
if err != nil {
log.Fatal(err)
}
title := doc.Find("title").First().Text()
c <- title
}
func main() {
urls := []string{
"https://example.com",
"https://example.org",
"https://example.net",
}
c := make(chan string)
for _, url := range urls {
go scrapeURL(url, c)
}
for range urls {
fmt.Println(<-c)
}
}
In the above code, we define a scrapeURL
function that takes a URL and a channel as parameters. It fetches the HTML content of the URL, extracts the title from it using GoQuery, and sends the title through the channel. We then launch multiple goroutines to scrape different URLs concurrently. Finally, we receive and print the titles from the channel.
Strategies for Web Scraping with Golang
1. Robots.txt
Before scraping a website, it's important to check its robots.txt
file. The robots.txt
file is a standard used by websites to communicate which parts of the site can be crawled by search engines and web scrapers. Respect the directives in the robots.txt
file to avoid legal issues and be a responsible web scraper.
2. Pause Between Requests
To prevent overwhelming a website's server and to avoid being blocked, it's important to include a pause or delay between consecutive requests. You can use the time.Sleep
function to introduce a delay in your scraping code. A reasonable delay helps ensure smooth and uninterrupted scraping without causing excessive load on the server.
3. Handle Rate Limits
Some websites may impose rate limits to prevent excessive scraping. A rate limit is a specified number of requests allowed within a certain time period. To handle rate limits, you can use techniques like exponential backoff or implement a queue system with a maximum request rate. Be mindful of the rate limits imposed by each website and adapt your scraping code accordingly.
4. Parse Structured Data
If a website provides structured data, such as JSON or XML, it's often more efficient to retrieve and parse this data directly instead of scraping the HTML. Structure data is easier to work with and less likely to change than the HTML structure. Many websites provide APIs that return structured data, so consider using those if available.
Conclusion
Golang provides excellent tools and strategies for web scraping, making it a powerful choice for scraping projects. The Colly and GoQuery libraries, along with goroutines and channels, enable you to write efficient and concurrent scraping code. Remember to follow good scraping practices, respect robots.txt
, and handle rate limits appropriately. With Golang's simplicity, performance, and powerful features, you'll be able to build robust web scraping applications.