Golang for System Programming: Working with Low-Level APIs
Discover the power of Golang for system programming with low-level APIs. Learn how to work with hardware, optimize performance, and create efficient system-level applications. Master the art of concurrency, leverage static typing, and gain cross-platform support.
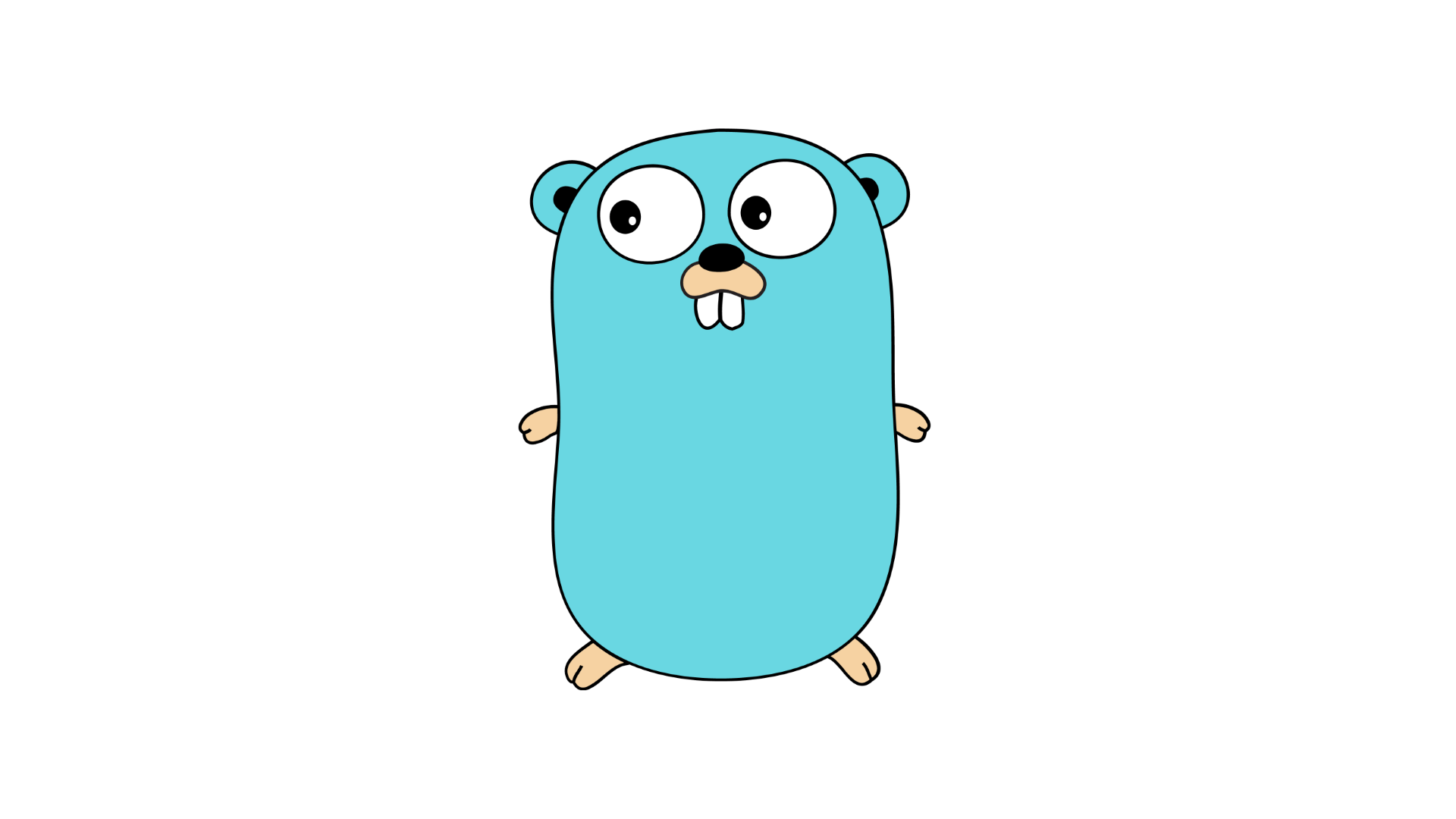
Introduction
Golang, also known as Go, is a powerful programming language that offers excellent capabilities for system programming. With its low-level APIs, Go allows developers to work closely with hardware and take control of system resources. In this article, we will explore how to use Go for system programming and work with low-level APIs.
What are Low-Level APIs?
Before we dive into Go's low-level APIs, let's briefly understand what low-level APIs are. Low-level APIs, also known as system-level APIs, provide low-level access to the underlying hardware and operating system. These APIs allow developers to interact with hardware devices, perform system-level operations, and optimize performance.
Why Use Golang for System Programming?
Golang is an excellent choice for system programming due to its combination of performance, simplicity, and productivity. Here are some reasons why you should consider using Go for system programming:
1. Concurrency
Golang provides built-in support for concurrency with its Goroutines and channels. This makes it easy to write concurrent programs and efficiently utilize system resources.
2. Performance
Go is known for its exceptional performance. It offers fast execution speed and efficient memory management, making it ideal for systems programming tasks that require high-performance.
3. Static Typing
Go is a statically typed language, which means that the type of variables is checked at compile-time. This helps catch errors early and ensures robustness in system-level programming.
4. Cross-Platform Support
Go supports multiple platforms, including Windows, Linux, macOS, and more. This makes it easy to develop system-level applications that can run on different operating systems.
Working with Low-Level APIs in Go
Now that we understand why Go is a great choice for system programming, let's explore how to work with low-level APIs in Go. Below are some key points to keep in mind:
1. Importing Low-Level Packages
To work with low-level APIs in Go, you need to import the relevant packages. These packages provide the necessary functions and data structures to interact with the low-level system resources. Some commonly used packages for system programming in Go include "syscall," "unsafe," and "reflect."
2. Working with Pointers
Pointers play a crucial role in low-level programming. They allow direct memory manipulation and enable efficient interaction with system resources. In Go, you can work with pointers using the "*" symbol.
3. Memory Management
When working with low-level APIs, it's essential to handle memory management carefully. Go provides garbage collection, which automatically frees unused memory. However, in some cases, you might need to manage memory manually using the "unsafe" package.
4. Calling C Functions
In certain situations, you may need to interact with existing C libraries or functions. Go allows you to seamlessly integrate C code using the "cgo" tool. This enables you to call C functions directly from your Go code.
Examples of Low-Level API Usage
Let's look at some examples to understand how low-level APIs can be used in Go:
1. Accessing System Registry
Using low-level APIs, you can access the system registry to read and modify system configurations. This can be useful for creating system utilities or configuration tools.
2. Interfacing with Hardware
Low-level APIs allow direct interaction with hardware devices such as sensors, GPIO pins, or network interfaces. This can be beneficial for building embedded systems or IoT applications.
3. System-Level File Operations
With low-level APIs, you can perform system-level file operations like reading, writing, or modifying files. This allows you to create file management tools or perform advanced file operations.
4. Optimizing System Performance
By utilizing low-level APIs, you can optimize system performance by fine-tuning memory allocation, multithreading, or other system-level operations. This can lead to faster and more efficient applications.
Conclusion
Golang provides powerful capabilities for system programming, thanks to its low-level APIs. By working with low-level APIs in Go, you can interact with system resources, optimize performance, and create efficient system-level applications. Whether you are building system utilities, managing hardware devices, or optimizing system performance, Go is an excellent choice for your system programming needs.
In the next part of our series, we will delve deeper into specific examples and demonstrate how to work with low-level APIs using Go. Stay tuned for more exciting content!