Golang Reactive Programming: Event-Driven Development with Channels
Learn how to harness the power of Golang's channels to implement event-driven development with reactive programming. Discover the benefits of concurrency and build highly responsive applications.
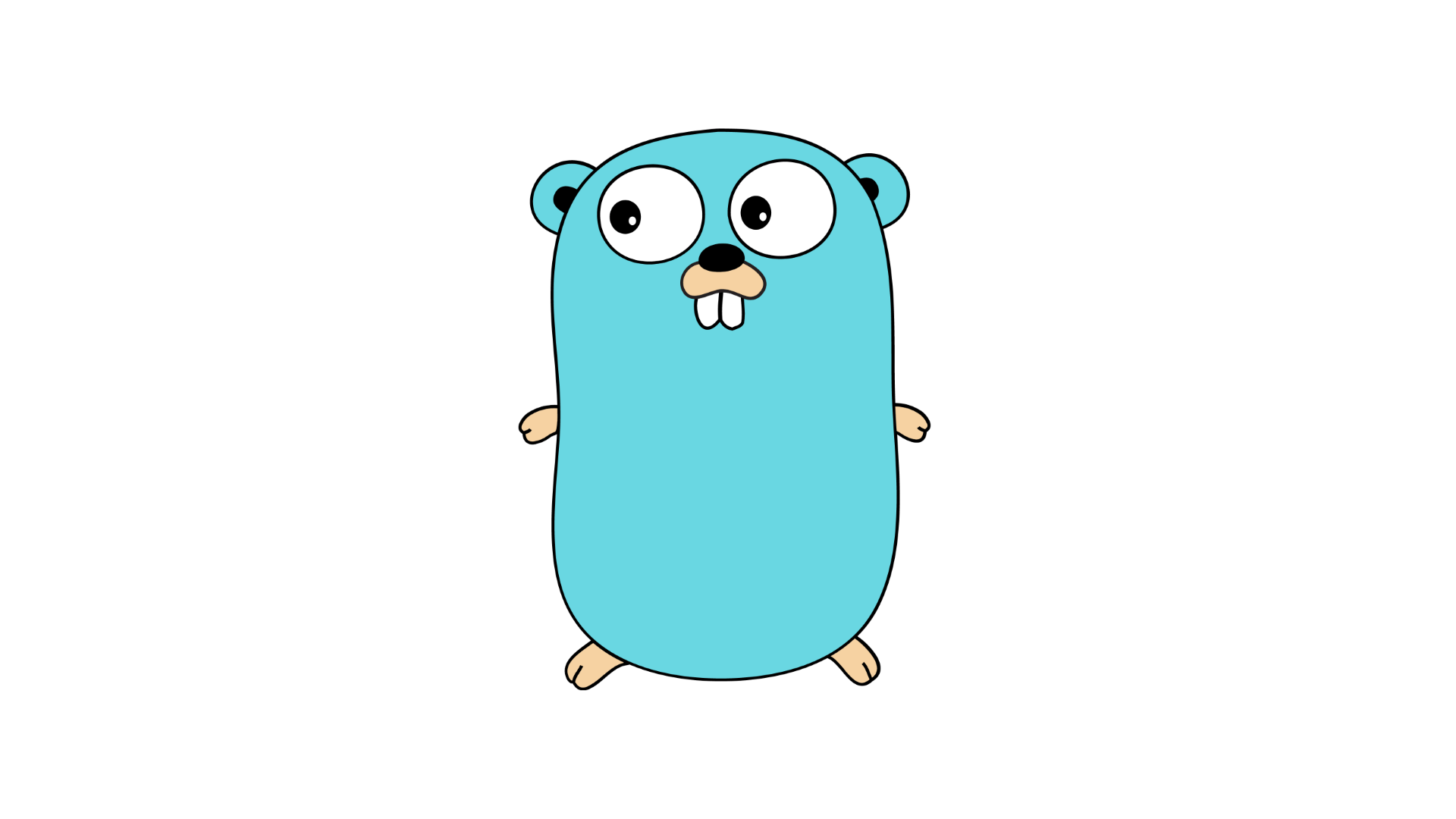
Introduction
Golang Reactive Programming: Event-Driven Development with Channels is a powerful concept in Go programming that allows developers to build scalable and highly responsive applications. By leveraging channels, developers can implement event-driven architectures that enable concurrent processing and communication between various components of an application.
What is Reactive Programming?
Reactive programming is an approach to software development that focuses on asynchronous and event-driven programming paradigms. It is designed to handle and react to a large number of events, making it particularly suitable for building real-time, highly scalable applications.
Why Use Reactive Programming with Golang?
Golang, also known as Go, is a programming language developed by Google that is known for its simplicity, efficiency, and built-in support for concurrency. These features make it an ideal choice for building event-driven applications using reactive programming.
Event-Driven Development
Event-driven development is a software development approach that focuses on producing code that responds to specific events or actions. In this approach, the behavior of the application is determined by the events that occur, rather than a predefined sequence of operations.
Channels in Golang
Channels are a key feature of Go's concurrency model and are essential for implementing event-driven development in Go. Channels allow for safe communication and synchronization between concurrent goroutines, enabling the seamless flow of data and events between different parts of the application.
Creating a Channel
To create a channel in Go, you can use the following syntax:
ch := make(chan dataType)
This syntax creates a channel of the specified data type, which can be used to send and receive values between goroutines.
Sending and Receiving Data
Once a channel is created, you can use the channel operator `<-
` to send and receive data through the channel. The `<-
` operator is used to send data into the channel, while `<-channelName
` is used to receive data from the channel.
// Sending data into the channel
ch <- data
// Receiving data from the channel
receivedData := <-ch
Channel Buffering
By default, channels in Go are unbuffered, which means that they only accept a value when a corresponding receiver is ready to receive it. However, you can also create buffered channels that can store a specified number of values, allowing the sender to continue sending data even if there is no immediate receiver.
To create a buffered channel, you can use the following syntax:
ch := make(chan dataType, bufferSize)
Closing a Channel
You can close a channel in Go to indicate that no more values will be sent on the channel. This can be useful to communicate that a channel has finished its work and no more data will be available.
// Closing a channel
close(ch)
Implementing Event-Driven Development with Channels
Now that you have a basic understanding of channels in Go, let's see how you can implement event-driven development using channels.
Step 1: Defining Events
The first step in implementing event-driven development is to define the events that your application will handle. Events can be specific actions, user interactions, or any other occurrence that triggers a response in your application.
type Event struct {
// Define event properties here
}
type EventHandler func(event Event)
Step 2: Listening for Events
Once you have defined the events, you can create a goroutine that listens for these events and processes them accordingly. This goroutine can use a select statement to handle multiple channels and respond to events as they occur.
func eventListener(ch chan Event, handler EventHandler) {
for event := range ch {
handler(event)
}
}
func main() {
eventChannel := make(chan Event)
go eventListener(eventChannel, handleEvent)
// Start other goroutines or perform other tasks
}
Step 3: Responding to Events
The handler function that you pass to the eventListener Goroutine will be responsible for processing the events and taking appropriate actions.
func handleEvent(event Event) {
// Process event and take appropriate actions
}
Conclusion
Golang Reactive Programming: Event-Driven Development with Channels allows developers to build highly responsive and concurrent applications. By leveraging channels, developers can implement event-driven architectures that enable efficient communication and processing between different components of the application.
By following the steps outlined in this guide, you can start building event-driven applications with Go and take advantage of Go's simplicity, efficiency, and built-in support for concurrency.
Stay tuned for more articles on Go programming and reactive programming concepts. Happy coding!