Golang Performance Optimization: Analyzing Bottlenecks and Improving Speed
In this article, we explore Go performance optimization by analyzing bottlenecks, using profilers, concurrency, efficient data structures, compiler flags, and testing. Striking a balance between speed and maintainability is key in the ongoing process of enhancing Go application performance.
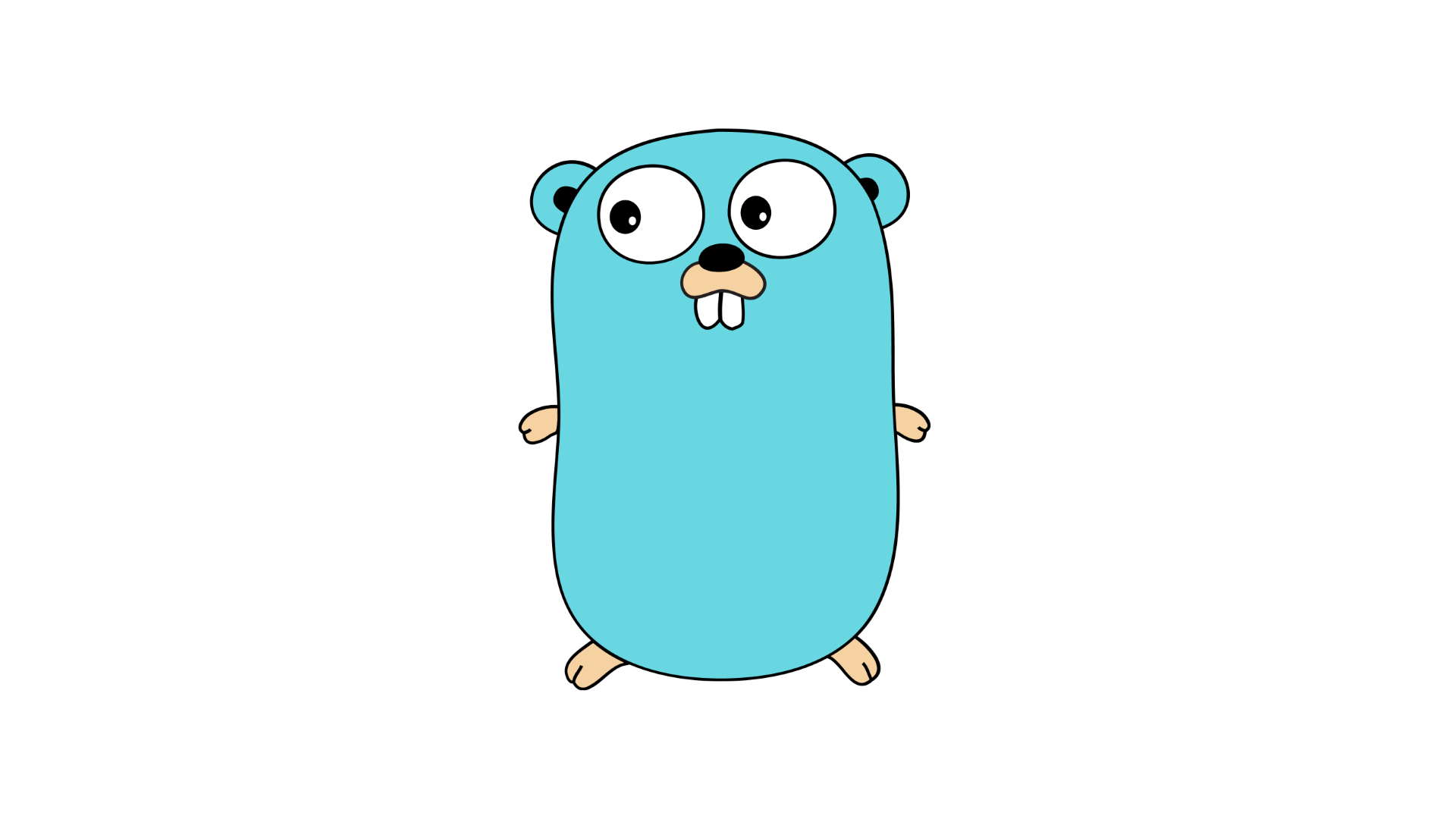
Introduction
Golang is a powerful programming language known for its efficiency, simplicity, and speed. However, even the most well-written Go programs can benefit from performance optimization. In this article, we will explore the process of analyzing bottlenecks in Go applications and implementing strategies to improve their speed.
Understanding Performance Bottlenecks
Before we dive into optimization techniques, let's understand what performance bottlenecks are. Performance bottlenecks are sections of code or system components that limit the overall speed or efficiency of an application. Identifying and addressing these bottlenecks is critical to improving the performance of your Go programs.
1. CPU Profiler
The first step in performance analysis is identifying the sections of code that consume the most CPU time. The CPU profiler is a powerful tool provided by the Go standard library that helps us analyze the CPU usage of a running program.
Here's an example of how to use the CPU profiler:
import (
"os"
"runtime/pprof"
)
func main() {
f, _ := os.Create("cpu_profile")
defer f.Close()
pprof.StartCPUProfile(f)
defer pprof.StopCPUProfile()
// Your code here
}
By running your program with the CPU profiler enabled, it will generate a CPU profile file that can be analyzed using various profiling tools.
2. Memory Profiler
Memory management is another crucial aspect of performance optimization. Identifying memory leaks or excessive memory usage can significantly impact the speed and efficiency of your Go applications.
Similar to the CPU profiler, Go provides a memory profiler that can be used to identify memory bottlenecks. Here's an example:
import (
"os"
"runtime/pprof"
)
func main() {
f, _ := os.Create("mem_profile")
defer f.Close()
pprof.WriteHeapProfile(f)
// Your code here
}
By running your program with the memory profiler enabled, it will generate a memory profile file that can be analyzed to identify memory usage patterns and potential memory leaks.
Optimization Techniques
Now that we have identified the bottlenecks in our code, it's time to implement strategies to optimize the performance of our Go applications.
1. Profiler Analysis
The first step in optimizing your Go program is to analyze the CPU and memory profiles generated by the profilers. This analysis will give you insights into the sections of code or system components that consume the most resources.
Look for functions or code blocks that have high CPU or memory usage and try to optimize them. Consider reducing unnecessary computations, minimizing allocations, or using more efficient algorithms.
2. Concurrency
Go is designed to support concurrency out of the box, and utilizing its goroutine and channel features can greatly improve the performance of your applications.
Identify sections of your code that can be executed concurrently and leverage goroutines to parallelize the workload. However, be careful with excessive allocation of goroutines, as it can cause overhead and potentially degrade performance.
3. Data Structures and Algorithms
Choosing the right data structures and algorithms is critical for good performance. Evaluate your code to ensure that you are using the most efficient data structures and algorithms for the task at hand.
For example, if you are frequently performing lookups on large data sets, consider using a hash table (map) instead of linear search algorithms. Regularly reviewing and optimizing data structures and algorithms can have a significant impact on the overall performance of your Go programs.
4. Compiler Optimizations
Go provides various compiler optimization flags that can be used to improve the performance of your code. These flags enable additional optimizations during the compilation process.
For example, the -m
flag can be used to print optimization decisions made by the compiler, allowing you to identify opportunities for further optimization. The -l
flag can be used to disable inlining, which can help reduce the size of your executable.
Experiment with different compiler optimization flags to find the best configuration for your specific application.
Testing and Benchmarking
When optimizing your Go applications, it's important to have a solid testing and benchmarking strategy in place. This allows you to measure the impact of various optimizations and ensure that they do not introduce new bugs or regressions.
Go provides a built-in testing framework that includes support for benchmarking. By writing comprehensive tests and benchmarks, you can accurately measure the impact of your optimizations and make data-driven decisions.
Wrapping Up
Optimizing the performance of your Go applications is a continuous process. By analyzing performance bottlenecks, implementing optimization techniques, and regularly testing and benchmarking your code, you can ensure that your Go programs run efficiently and deliver the best possible performance.
Remember, optimization is not about premature optimization or sacrificing readability, but about making informed decisions based on performance analysis. Strive for code that is both performant and maintainable.
Stay tuned for more articles on Go programming, where we will explore advanced optimization strategies, profiling tools, and real-world performance scenarios. Happy optimizing!