Golang for Network Programming: Socket Programming and Beyond
Explore the world of network programming in Go (Golang). Master socket programming, concurrency, and advanced protocols, and build efficient, scalable network applications.
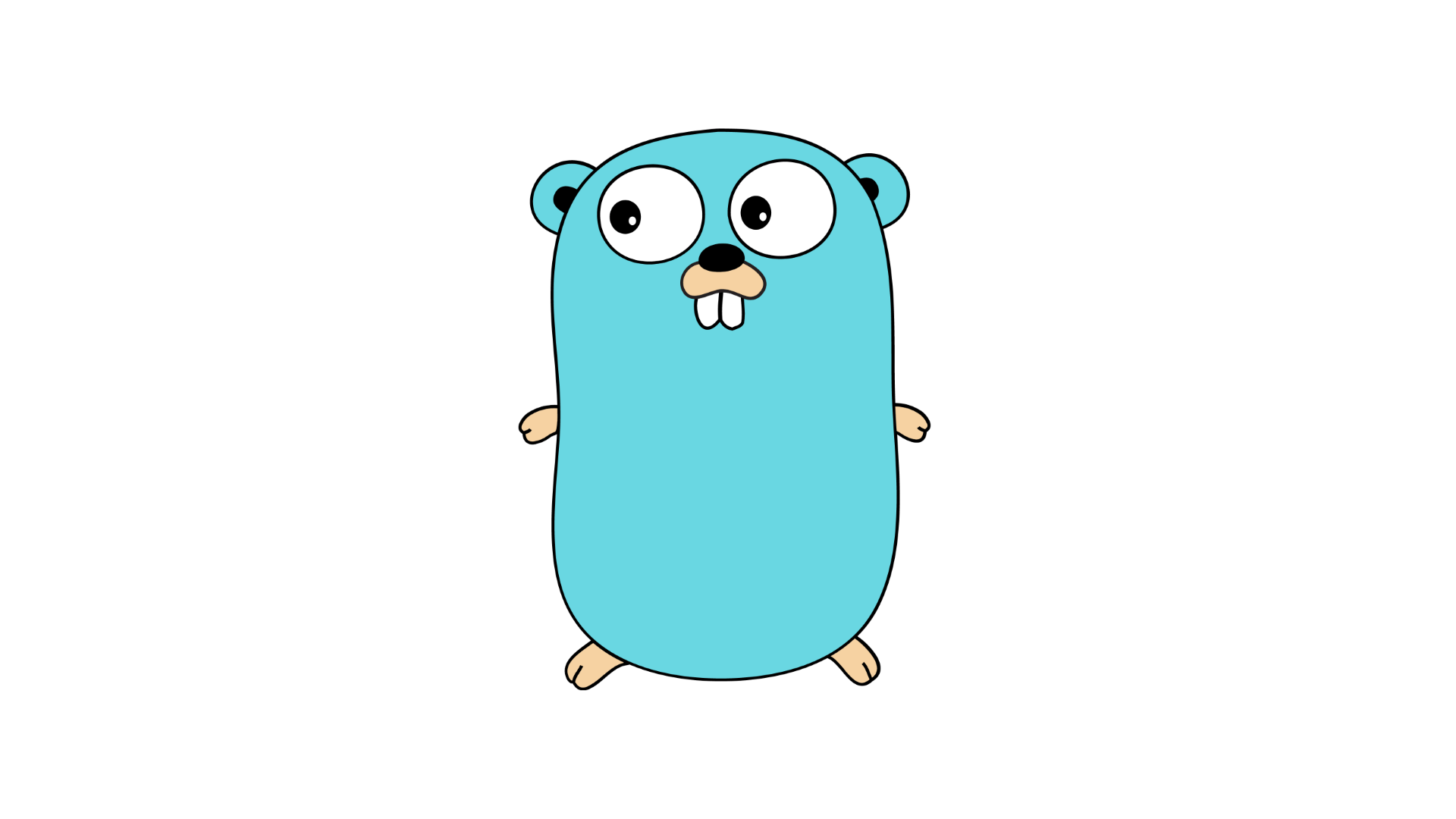
Introduction
When it comes to network programming, Go (Golang) is an excellent choice due to its simplicity, efficiency, and built-in support for concurrency. In this guide, we'll explore the world of network programming in Go, starting with the fundamentals of socket programming and going beyond to advanced techniques. Whether you're a beginner or an experienced developer, this article will equip you with the knowledge and skills to build robust network applications using Go.
What is Socket Programming?
Socket programming is a technique that allows two endpoints (usually on different machines) to communicate over a network by establishing a socket connection. In simple terms, a socket is an endpoint for sending or receiving data between two nodes on a network. It provides a communication channel through which programs can exchange data.
Sockets in Go
Go provides a powerful set of libraries and functions for socket programming. The core net
package in Go includes essential primitives for creating and interacting with sockets. By leveraging these tools, you can build a wide range of network applications, including client-server architectures, peer-to-peer communication, and more.
Getting Started with Golang Network Programming
Before diving into network programming, you need to set up a development environment for Go. Follow these steps to get started:
1. Install Go
To begin, download and install the Go programming language from the official website (https://golang.org). Follow the installation instructions specific to your operating system.
2. Set up your workspace
Go follows a specific workspace structure for organizing your projects. Create a directory to serve as your workspace and set the GOPATH
environment variable to point to this directory. This ensures that Go can find your packages and dependencies correctly.
3. Write your first Golang network program
Now that your workspace is set up, it's time to write your first Go network program. Open your favorite text editor or integrated development environment (IDE) and create a new file with a .go
extension. Let's start with a simple "Hello, World!" example:
package main
import (
"fmt"
"net"
)
func main() {
conn, err := net.Dial("tcp", "example.com:80")
if err != nil {
fmt.Println("Error connecting:", err)
return
}
_, err = conn.Write([]byte("GET / HTTP/1.0\r\n\r\n"))
if err != nil {
fmt.Println("Error sending request:", err)
return
}
buffer := make([]byte, 1024)
_, err = conn.Read(buffer)
if err != nil {
fmt.Println("Error reading response:", err)
return
}
fmt.Println("Response:", string(buffer))
conn.Close()
}
In this example, we create a TCP connection to example.com
on port 80
using the net.Dial
function. We then send an HTTP request to the server and read the response. Finally, we print the response to the console. Build and run the program using the go run
command and observe the output.
Beyond Socket Programming
Socket programming is just the beginning of the possibilities you can explore with Go for network programming. Here are some advanced topics you can dive into:
1. Concurrency in Go
Go's built-in support for concurrency makes it an excellent choice for network programming. You can leverage Goroutines and channels to handle multiple client connections concurrently, improving the performance and scalability of your network applications.
2. Protocol Implementations
Go allows you to implement various network protocols like TCP, UDP, and HTTP. You can build your own servers or clients for these protocols, giving you full control over the network communication.
3. Websockets
Websockets enable real-time, bidirectional communication between clients and servers. Go has excellent support for building websocket servers and clients, making it easy to develop applications like chatrooms, real-time collaboration tools, and more.
Conclusion
In this guide, we introduced you to the world of network programming in Go. We covered the basics of socket programming and provided an example of a simple network program. We also explored some advanced topics you can dive into to take your network programming skills to the next level. With Go's simplicity and power, you have a powerful toolkit at your disposal for building efficient, scalable, and reliable network applications.
Now that you have a solid foundation in Go network programming, it's time to start exploring the possibilities and building your own network applications. Happy coding!