Golang for Microservices: Designing and Architecting Distributed Systems
Learn how to design and architect distributed systems using Golang for microservices. Discover best practices for scalability, efficiency, communication, error handling, and security. Explore the power of Golang in building resilient and scalable distributed systems.
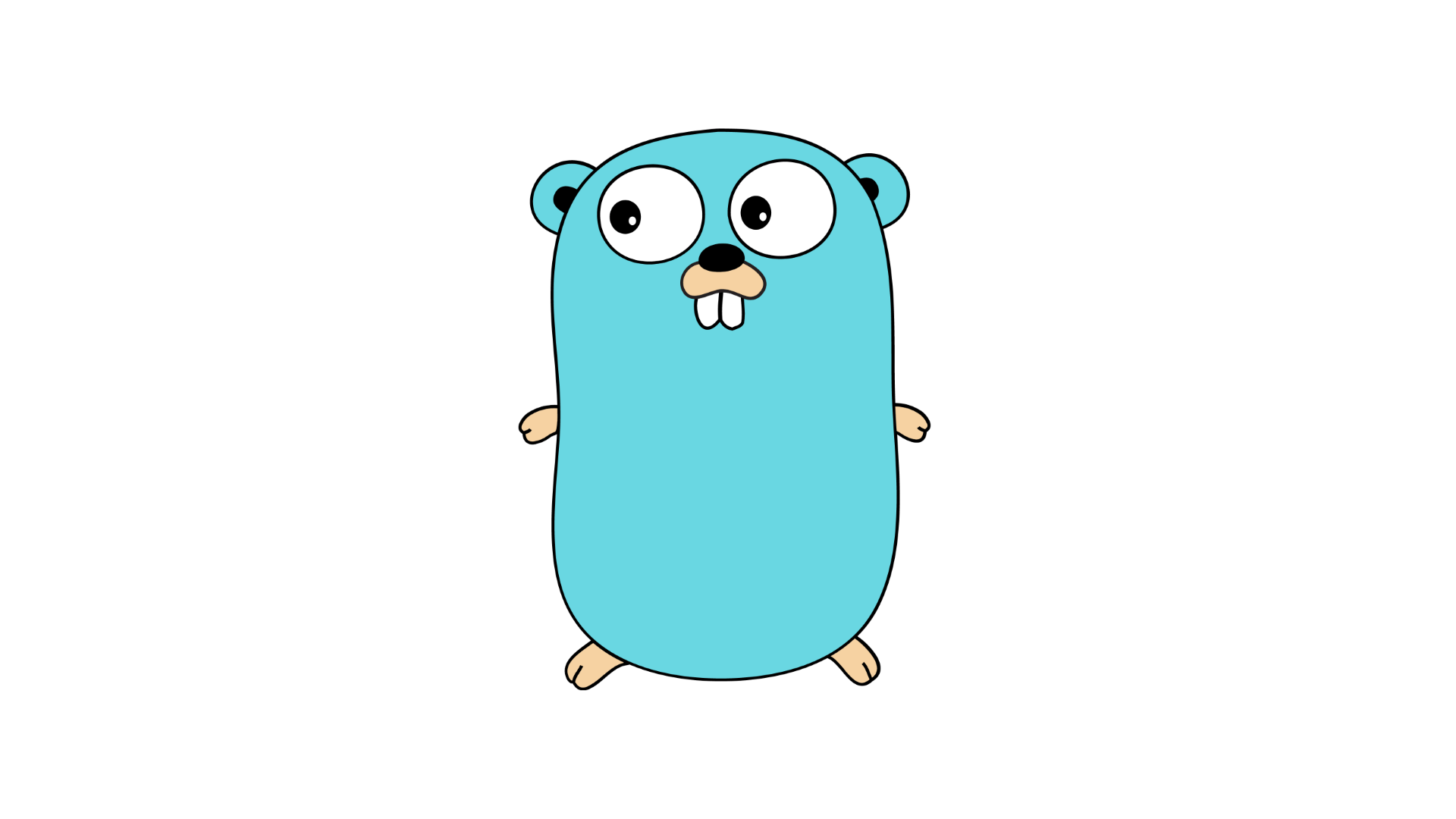
Golang for Microservices: Designing and Architecting Distributed Systems
Welcome to our comprehensive guide on using Golang for designing and architecting distributed systems with microservices. In this article, we will explore the key concepts and best practices for building scalable and efficient distributed systems using the Go programming language.
What are Microservices?
Microservices are a software architecture pattern where applications are broken down into small, loosely-coupled services that can be developed, deployed, and scaled independently. Each microservice focuses on a specific business capability and communicates with other microservices through lightweight protocols like HTTP or messaging systems like RabbitMQ or Apache Kafka.
By adopting a microservices architecture, organizations can achieve greater flexibility, resilience, and scalability compared to monolithic architectures. Golang, with its built-in concurrency primitives and efficient execution, is an ideal programming language for developing microservices.
Designing Microservices with Golang
Designing microservices requires careful consideration of various aspects, including service boundaries, communication mechanisms, data storage, and error handling. Let's explore some key principles and best practices for designing microservices in Golang.
1. Designing Service Boundaries
When defining microservices, it is important to establish clear service boundaries that reflect independent business capabilities. Each microservice should have a single responsibility and expose well-defined APIs for communication with other microservices.
In Golang, you can define each microservice as a separate package or module, encapsulating its functionality within the package boundaries. This promotes better code organization and decoupling between microservices.
2. Communicating between Microservices
Microservices communicate with each other through various protocols like HTTP, gRPC, or messaging systems. In Golang, you can use the built-in `net/http` package to create HTTP-based APIs for inter-service communication.
Golang's concurrency primitives like goroutines and channels make it easy to implement asynchronous communication between microservices, enabling parallel processing and improved system performance.
3. Data Storage for Microservices
Microservices often require independent data storage to maintain their own state. Golang provides various libraries and frameworks for interacting with different data storage systems like SQL databases (e.g., MySQL, PostgreSQL), NoSQL databases (e.g., MongoDB, Cassandra), or key-value stores (e.g., Redis).
When choosing a data storage solution for microservices, consider factors like data consistency, scalability, and availability to ensure optimal performance and reliability of your distributed system.
4. Error Handling and Resilience
In distributed systems, failures are inevitable. Golang provides error handling mechanisms like panics and recoveries, along with libraries like github.com/Netflix/hystrix-go
for implementing circuit breakers and github.com/go-kit/kit
for resilience patterns like retries, timeouts, and rate limiting.
By implementing robust error handling and resilience mechanisms, you can ensure that your microservices can recover from failures gracefully and provide uninterrupted service.
Architecting Distributed Systems with Golang
Golang's simplicity, strong typing, and efficient execution make it well-suited for building distributed systems. When architecting a distributed system with Golang, consider the following key aspects:
1. Service Discovery and Routing
Service discovery and routing enable dynamic discovery of microservices and efficient request routing. Libraries like github.com/etcd-io/etcd
and github.com/go-kratos/kratos
provide service discovery features that help in building scalable distributed systems.
2. Load Balancing
To distribute incoming traffic across multiple instances of a microservice for load balancing, you can use load balancing algorithms like round-robin, weighted round-robin, or consistent hashing. Libraries like github.com/grpc/grpc-go
and github.com/uber-go/atomic
provide load balancing capabilities in Golang.
3. Monitoring and Observability
Monitoring and observability are crucial for understanding the health and performance of a distributed system. Using tools like Prometheus and Grafana, you can collect and visualize metrics, set up distributed logging, and gain insights into the behavior and efficiency of your microservices.
4. Security and Authentication
Securing microservices involves implementing authentication, authorization, and encryption mechanisms. In Golang, libraries like golang.org/x/oauth2
and github.com/dgrijalva/jwt-go
can be used for implementing OAuth2 and JWT-based authentication, while github.com/gorilla/sessions
provides session management capabilities.
Conclusion
In this article, we explored the key concepts and best practices for designing and architecting distributed systems using Golang for microservices. We covered topics like service boundaries, inter-service communication, data storage, error handling, and resilience. We also discussed aspects of architecting distributed systems, including service discovery, load balancing, monitoring, and security.
Golang's simplicity, concurrency model, and performance characteristics make it an excellent choice for developing microservices and building scalable distributed systems. By following the principles and best practices outlined in this article, you can ensure the efficient and effective implementation of your distributed system.
Stay tuned for the next part of our series, where we will dive deeper into specific aspects of Golang for microservices and explore advanced techniques for building resilient and scalable distributed systems.