Golang for DevOps: Automating Infrastructure and Deployment
Learn how to automate infrastructure and deployment with Golang. Discover the powerful features of Golang workspace structure, build web servers, and deploy them to production. Boost your DevOps skills now!
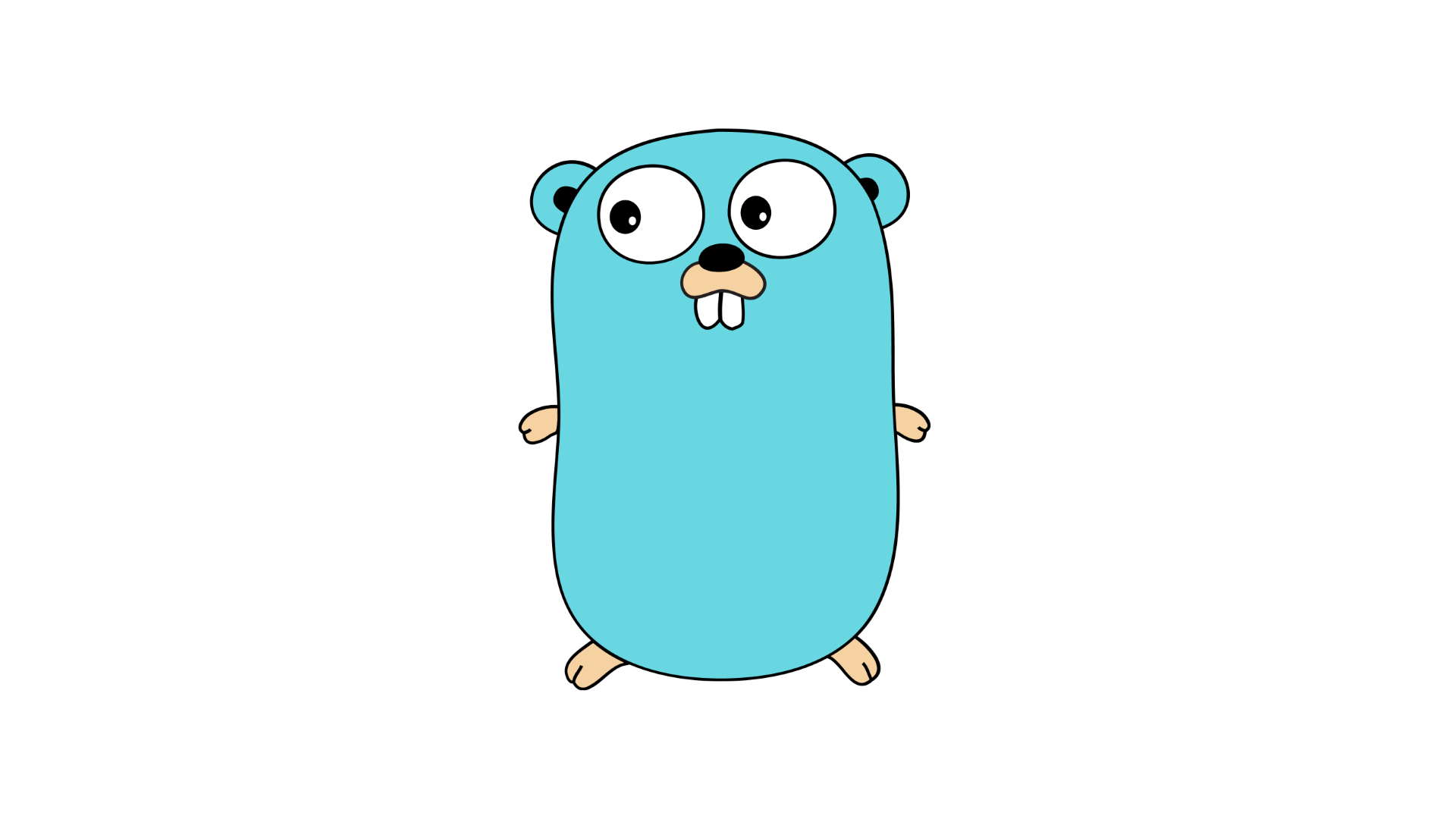
Introduction
As a beginner in the field of DevOps, understanding how to automate infrastructure and deployment can be challenging. However, with the help of Go (Golang), you can simplify and streamline these processes. Go is a powerful and efficient programming language that is gaining popularity in the DevOps community due to its simplicity, performance, and concurrency. In this guide, we will explore how Go can be used to automate infrastructure and deployment, empowering you to become a more efficient and effective DevOps engineer.
The Benefits of Automating Infrastructure and Deployment
Before diving into the details of using Go for automating infrastructure and deployment, let's first understand why it is important to do so. Here are some key benefits:
- Saves time and effort: By automating infrastructure and deployment, repetitive tasks can be eliminated, saving valuable time and effort that can be redirected towards more critical tasks.
- Improves consistency: Manual configuration and deployment processes can lead to inconsistencies and errors. Automation ensures that every infrastructure and deployment step is performed consistently, reducing the risk of issues arising due to human error.
- Enhances scalability: With the help of automation, resources can be provisioned and deployed quickly and efficiently, allowing for seamless scalability as demand increases.
- Increases reliability: Automated infrastructure and deployment processes can be tested and validated, ensuring that they work reliably every time. This reduces the risk of downtime and enhances the overall reliability of the system.
Getting Started with Golang for DevOps
To begin using Go for automating infrastructure and deployment, you'll need to have Go installed on your machine. You can download and install Go from the official website (https://golang.org/). Once installed, you can check if Go is properly set up by running the following command in your terminal:
$ go version
If you see the version number of Go printed, it means that Go is successfully installed and configured on your machine.
Creating a New Go Project
Now that Go is set up, let's create a new Go project. Go follows a specific workspace structure, which consists of three main directories: src
, pkg
, and bin
. The src
directory is used to store your Go source code, the pkg
directory contains compiled package objects, and the bin
directory holds the executable binaries.
1. src
Directory
The src
directory acts as the repository for your Go source code. It houses all your Go projects, each residing in its own subdirectory. This organization promotes project management and keeps the codebase organized and maintainable.
2. pkg
Directory
The pkg
directory stores compiled package objects generated during the build process. These compiled packages are reusable and can be imported into different projects within your workspace. The pkg
directory facilitates code reuse and eliminates the need for redundant recompilation.
3. bin
Directory
The bin
directory contains the executable binaries resulting from the build process. When you compile a Go program, the resulting binary is stored in the bin
directory. These binaries can be executed directly from the command line, allowing you to test and run your applications effortlessly.
Configuring and Customizing the Workspace
Although Go recommends a specific workspace structure, you have the flexibility to customize it to suit your needs. For instance, you can further organize your projects into subdirectories based on their purpose, such as src/github.com/your-username/project-name
. This approach proves especially useful when collaborating with version control systems like Git.
Furthermore, you can customize your workspace by setting the GOPATH
environment variable. By default, Go assumes the GOPATH
to be the workspace directory, but you can modify it to a different location if desired.
Building a Web Server
Now that we have our project set up, let's start building a simple web server using Go. In this example, we will use the standard Go library's net/http
package to create a basic HTTP server that listens on a specified port and responds to incoming requests.
Here is an example code snippet to get you started:
package main
import (
"fmt"
"net/http"
)
func main() {
// Define the handler function
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, World!")
})
// Start the server and listen on port 8080
http.ListenAndServe(":8080", nil)
}
Save the above code in a file named main.go
within your project's src
directory. To build and run the web server, navigate to your project's root directory in the terminal and run the following command:
$ go run main.go
You should see an output indicating that the server is running. Open a web browser and visit http://localhost:8080
. You should see the message "Hello, World!" displayed in the browser.
Deploying the Web Server
Now that we have a working web server, let's explore how we can deploy it to a production environment. There are various methods you can use to deploy a Go application, depending on your requirements and infrastructure. Here are a few common options:
- Deploying on a Virtual Machine: You can deploy your Go application on a virtual machine by creating a VM instance, installing Go, and running your application on the VM.
- Containerization with Docker: Docker allows you to package your application along with its dependencies into a container. This container can then be deployed on any server that has Docker installed.
- Using a Cloud Platform: Cloud platforms like AWS, Google Cloud, and Azure provide managed services for deploying Go applications. You can leverage these platforms to easily deploy and scale your application.
Choose the deployment method that best suits your needs and follow the appropriate documentation to deploy your web server to a production environment.
Conclusion
In this guide, we explored how Go can be used to automate infrastructure and deployment in the DevOps workflow. By leveraging the power and simplicity of Go, you can streamline and optimize your development process, saving time and effort. We covered the basics of the Go workspace structure, configuring and customizing it to suit your needs, building a web server, and deploying it to a production environment. Armed with this knowledge, you are now well-equipped to start automating infrastructure and deployment using Go.
Remember to continuously learn and explore new techniques and tools in the DevOps landscape to stay ahead and enhance your skills as a DevOps engineer. Happy coding!