Golang Design Patterns: Implementing Essential Patterns in Practice
Explore Golang design patterns and learn how to implement Singleton, Factory, Builder, and Observer patterns. Improve code quality, maintainability, and scalability. Essential for any Golang developer!
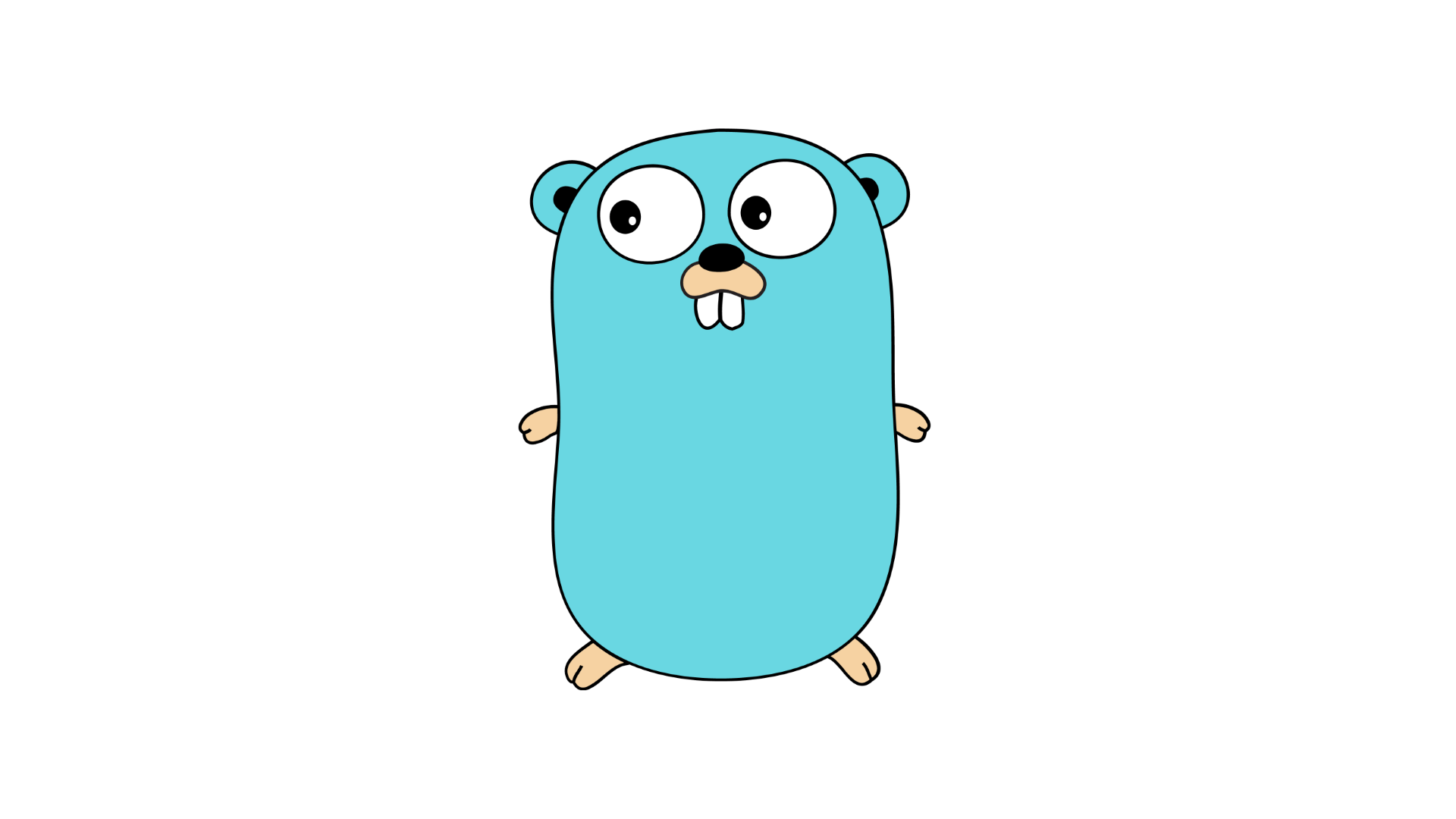
Introduction
Design patterns are an essential aspect of software development. They provide reusable solutions to common programming problems, improving code quality, maintainability, and scalability. In this blog post, we will explore Golang design patterns and demonstrate how to implement them in practice. Whether you are a beginner or an experienced developer, understanding and using design patterns can greatly enhance your Golang projects. Let's dive into the world of Golang design patterns!
Benefits of Using Design Patterns
Before we delve into the details of Golang design patterns, let's take a moment to understand why they are beneficial:
- Code reusability: Design patterns help developers reuse proven solutions to common problems, reducing the need to reinvent the wheel and saving development time.
- Maintainability and scalability: Using design patterns ensures that your code is structured, organized, and modular, making it easier to maintain and scale as your project grows.
- Communication and collaboration: Design patterns provide a common language and understanding among developers, facilitating effective communication and collaboration within a team.
- Best practices: Design patterns embody best practices and guidelines for solving specific problems, ensuring that your code follows industry standards and quality practices.
Singleton Pattern
The Singleton pattern is one of the most commonly used design patterns. It ensures that a class has only one instance and provides a global point of access to it. In Golang, we can implement the Singleton pattern using a combination of package-level variables and functions. Here's an example:
package singleton
import "sync"
var instance *singleton
var once sync.Once
type singleton struct {
// your singleton struct fields
}
// GetInstance returns the singleton instance
func GetInstance() *singleton {
once.Do(func() {
instance = &singleton{}
})
return instance
}
// add your singleton methods here
In the above code, we define a package-level variable instance
to hold the singleton instance. We also use the sync.Once
type to ensure that the creation of the singleton instance happens only once, even in concurrent scenarios. The GetInstance
function returns the singleton instance, creating it if it doesn't exist yet.
Factory Pattern
The Factory pattern is another commonly used design pattern in Golang. It provides an interface for creating objects without specifying their exact class. This pattern is useful when you want to abstract the object creation process and make it more flexible and extensible. Here's an example implementation of the Factory pattern:
package factory
type Shape interface {
Draw() string
}
type Circle struct {}
// Circle implements the Shape interface
func (c *Circle) Draw() string {
return "Drawing a circle"
}
type Rectangle struct {}
// Rectangle implements the Shape interface
func (r *Rectangle) Draw() string {
return "Drawing a rectangle"
}
func CreateShape(shapeType string) Shape {
switch shapeType {
case "circle":
return &Circle{}
case "rectangle":
return &Rectangle{}
default:
return nil
}
}
In the above code, we define an interface Shape
that declares a common method Draw
. We then create two concrete types, Circle
and Rectangle
, that implement the Shape
interface. The CreateShape
function acts as the factory, creating and returning the appropriate shape object based on the input shape type.
Builder Pattern
The Builder pattern is useful when you want to create complex objects step by step. It separates the construction of an object from its representation, allowing you to create different object representations using the same construction process. Here's an example implementation of the Builder pattern:
package builder
// Product represents the object that we want to create
type Product struct {
// Product fields
}
// Builder defines the steps to construct the product
type Builder interface {
StepOne() Builder
StepTwo() Builder
Build() *Product
}
// ConcreteBuilder implements the Builder interface
type ConcreteBuilder struct {
product *Product
}
// StepOne implements the first construction step
func (c *ConcreteBuilder) StepOne() Builder {
// Perform step one
return c
}
// StepTwo implements the second construction step
func (c *ConcreteBuilder) StepTwo() Builder {
// Perform step two
return c
}
// Build returns the final product
func (c *ConcreteBuilder) Build() *Product {
// Return the constructed product
return c.product
}
// Director directs the construction process
type Director struct {
builder Builder
}
// Construct invokes the construction steps in a specific order
func (d *Director) Construct() *Product {
d.builder.StepOne().StepTwo()
return d.builder.Build()
}
// Usage example
func main() {
builder := &ConcreteBuilder{}
director := &Director{builder: builder}
product := director.Construct()
}
In the above code, we define the Product
struct that represents the object we want to create. The Builder
interface declares the construction steps, and the ConcreteBuilder
struct implements these steps. The Director
struct directs the construction process by invoking the steps in a specific order using a builder object. Finally, in the usage example, we create a builder, instantiate a director with the builder, and invoke the Construct
method to obtain the final product.
Observer Pattern
The Observer pattern is useful when you want to establish a one-to-many relationship between objects, where the change in one object triggers a notification to all the dependent objects. This pattern allows for loose coupling between objects, as they only need to know about the subject they are observing. Here's an example implementation of the Observer pattern in Golang:
package observer
type Observer interface {
Update(message string)
}
type Subject struct {
observers []Observer
}
func (s *Subject) Attach(o Observer) {
// Add observer to the observers list
}
func (s *Subject) Detach(o Observer) {
// Remove observer from the observers list
}
func (s *Subject) Notify(message string) {
for _, observer := range s.observers {
observer.Update(message)
}
}
// Usage example
func main() {
subject := &Subject{}
observer1 := &ConcreteObserver{}
observer2 := &ConcreteObserver{}
subject.Attach(observer1)
subject.Attach(observer2)
subject.Notify("Hello!")
}
In the above code, we define the Observer
interface that declares the Update
method. The Subject
struct maintains a list of observers and provides methods to attach, detach, and notify them. In the usage example, we create a subject and two concrete observers, attach the observers to the subject, and then notify all the observers with a message.
Conclusion
In this blog post, we explored some essential Golang design patterns and demonstrated how to implement them in practice. The Singleton, Factory, Builder, and Observer patterns are just a few examples of the many design patterns available to Golang developers. By incorporating these patterns into your projects, you can improve code quality, maintainability, and scalability. Design patterns are a valuable tool in every developer's arsenal, and mastering them will greatly enhance your Golang development skills. Happy coding!