Golang Data Structures and Algorithms: Implementations and Performance
Learn about implementing and optimizing data structures and algorithms in Go (Golang). Explore the performance characteristics and practical use cases. Improve your coding skills with these essential techniques.
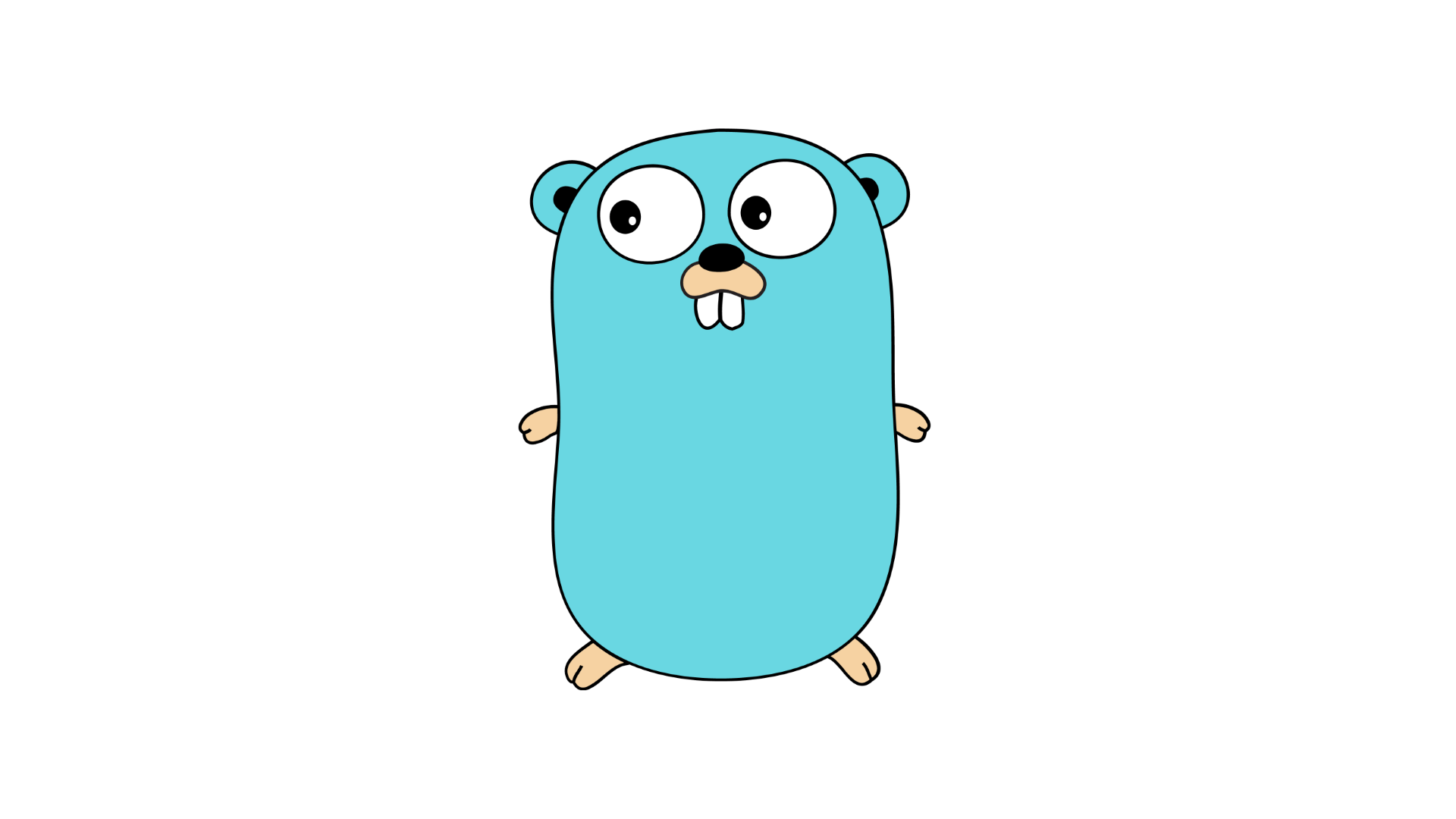
Golang Data Structures and Algorithms: Implementations and Performance
When it comes to developing efficient and scalable software applications, understanding data structures and algorithms is crucial. In the world of Go (Golang), a powerful programming language known for its simplicity and performance, mastering data structures and algorithms can take your skills to the next level. In this article, we will explore different implementations of data structures and algorithms in Go and analyze their performance characteristics.
Why Data Structures and Algorithms Matter
Data structures and algorithms are the building blocks of software development. They provide efficient ways to store and manipulate data, enabling us to solve complex problems with speed and accuracy. Whether you are developing a web application, a mobile app, or a system-level software, knowledge of data structures and algorithms can make a significant difference in the performance and reliability of your code.
Common Data Structures in Go
Go provides a rich set of built-in data structures that you can leverage in your applications. Some of the commonly used data structures in Go include:
1. Arrays
Arrays are fixed-size collections of elements of the same type. They provide efficient random access to elements but have a fixed length that cannot be changed once defined.
2. Slices
Slices are dynamic arrays that can grow or shrink as needed. They are a more flexible alternative to arrays and are widely used in Go for their versatility and convenience.
3. Linked Lists
Linked lists are data structures that consist of nodes, where each node contains a value and a reference to the next node in the list. Linked lists are particularly useful when you need efficient insertion or deletion operations.
4. Stacks
Stacks are data structures that follow the LIFO (Last In, First Out) principle. They are often used to implement function call stacks, undo/redo functionality, and parsing algorithms.
5. Queues
Queues are data structures that follow the FIFO (First In, First Out) principle. They are commonly used in scenarios where you need to process elements in the order they arrive, such as task scheduling and message passing systems.
6. Trees
Trees are hierarchical data structures that consist of nodes connected by edges. They are used in a wide range of applications, including search algorithms, file system organization, and hierarchical data representation.
7. Graphs
Graphs are a collection of nodes (vertices) and edges that connect them. They are used to represent complex relationships between entities and are often employed in social networks, route planning, and network analysis.
Implementations of Data Structures in Go
Go's standard library provides efficient implementations of common data structures. Let's take a look at some examples:
1. Array Implementation
Go's built-in arrays provide a simple and efficient way to store a fixed number of elements of the same type. Here's an example of creating and accessing elements in an array:
// Declare an array of integers with a fixed length of 5
var numbers [5]int
// Assign values to the array elements
numbers[0] = 1
numbers[1] = 2
numbers[2] = 3
numbers[3] = 4
numbers[4] = 5
// Access array elements
fmt.Println(numbers[0]) // Output: 1
fmt.Println(numbers[2]) // Output: 3
2. Slice Implementation
Slices provide a more flexible way to work with arrays. They are dynamic and can grow or shrink as needed. Here's an example of using slices in Go:
// Declare a slice of integers
numbers := []int{1, 2, 3, 4, 5}
// Add an element to the slice
numbers = append(numbers, 6)
// Access slice elements
fmt.Println(numbers[0]) // Output: 1
fmt.Println(numbers[2]) // Output: 3
3. Linked List Implementation
In Go, you can implement linked lists using structures and pointers. Here's an example of a singly linked list:
type Node struct {
value int
next *Node
}
type LinkedList struct {
head *Node
tail *Node
}
func (ll *LinkedList) Add(value int) {
newNode := &Node{value: value}
if ll.head == nil {
ll.head = newNode
} else {
ll.tail.next = newNode
}
ll.tail = newNode
}
// Usage
list := LinkedList{}
list.Add(1)
list.Add(2)
list.Add(3)
Performance of Data Structures in Go
The performance of data structures in Go depends on various factors, including the specific implementation, the size of the input, and the type of operations performed. Let's compare the performance of some common data structures in Go:
1. Arrays vs Slices
Arrays and slices have similar performance characteristics for most operations. However, slices provide more flexibility and are generally preferred in Go due to their dynamic nature.
2. Linked Lists vs Arrays or Slices
Linked lists excel in insertions and deletions, especially in the middle of the list, as they require updating only a few pointers. However, they have higher memory overhead and slower random access compared to arrays or slices.
3. Stacks and Queues
Stacks and queues have constant-time complexity for push and pop operations, making them highly efficient for their intended use cases.
4. Trees and Graphs
The performance of tree and graph operations depends on the specific algorithms used, such as depth-first search (DFS) or breadth-first search (BFS). The choice of data structure and algorithm can significantly impact the performance of these operations.
Conclusion
Understanding the implementations and performance characteristics of data structures and algorithms in Go is essential for efficient software development. By leveraging the built-in data structures and selecting appropriate algorithms, you can build high-performance applications. Remember to consider the specific requirements of your application and choose data structures and algorithms accordingly.
In the next part of our series, we will delve deeper into specific data structures and algorithms in Go, providing detailed examples and performance analysis. Stay tuned for an exciting journey into the world of Go!