Exploring Golang's Database Connectivity: SQL and NoSQL Databases
Learn how to connect and interact with SQL and NoSQL databases in Go. Explore libraries like database/sql and GORM for SQL databases, and MongoDB and Redis for NoSQL databases. Follow best practices for efficient and secure database connectivity.
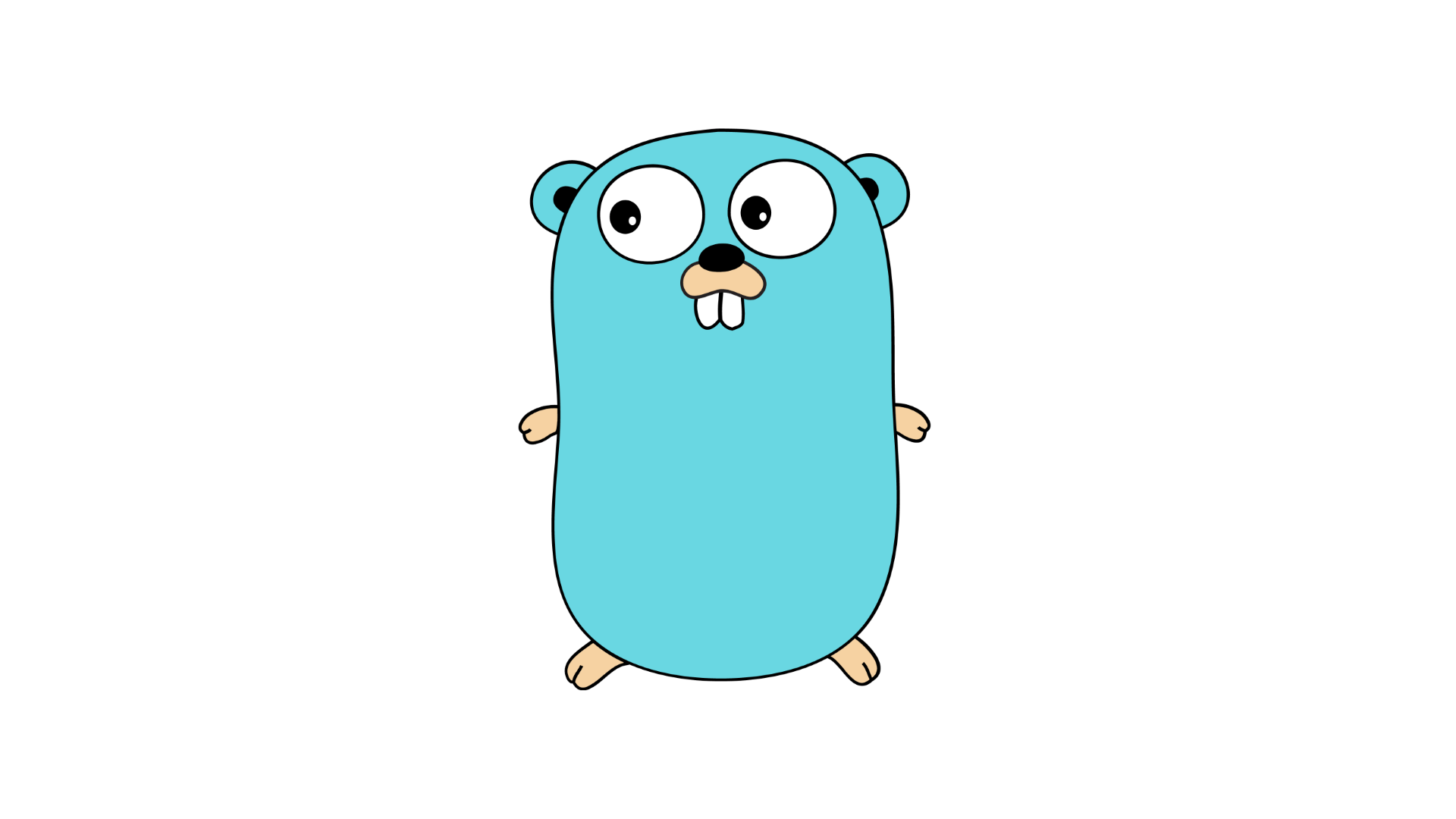
Introduction
Golang (Go) provides excellent support for working with databases, both SQL and NoSQL. Whether you're building a web application, a command-line tool, or any other type of software, understanding how to connect and interact with databases is crucial for storing and retrieving data. In this blog post, we will explore the different options available for connecting to SQL and NoSQL databases in Go, and cover some best practices for database connectivity.
SQL Databases in Go
SQL databases are a popular choice for structured data storage, and Go offers comprehensive support for them. Let's take a look at a few popular SQL database libraries in the Go ecosystem:
1. database/sql
package
The database/sql
package is part of the Go standard library and provides a generic interface for working with SQL databases. It follows the database/sql/driver interface, allowing you to use various SQL drivers such as SQLite, MySQL, PostgreSQL, and more. Using the database/sql
package, you can connect to databases, execute queries, and retrieve data.
```go import ( "database/sql" _ "github.com/go-sql-driver/mysql" ) func main() { // Open a connection to the database db, err := sql.Open("mysql", "user:password@tcp(host:port)/database_name") if err != nil { log.Fatal(err) } // Close the database connection when finished defer db.Close() // Execute a query rows, err := db.Query("SELECT * FROM users") if err != nil { log.Fatal(err) } // Retrieve data from the query result for rows.Next() { var id int var name string if err := rows.Scan(&id, &name); err != nil { log.Fatal(err) } fmt.Println(id, name) } } ```
2. GORM
GORM is an Object-Relational Mapping (ORM) library for Go that simplifies database access and provides a high-level API for working with SQL databases. It supports various databases, including SQLite, MySQL, PostgreSQL, and SQL Server. GORM allows you to define models, perform queries, and handle database relationships with ease.
```go import ( "gorm.io/gorm" "gorm.io/driver/mysql" ) type User struct { gorm.Model Name string Email string } func main() { // Open a connection to the database dsn := "user:password@tcp(host:port)/database_name" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{}) if err != nil { log.Fatal(err) } // Close the database connection when finished defer db.Close() // Auto-migrate the tables db.AutoMigrate(&User{}) // Create a new user user := User{Name: "John Doe", Email: "[email protected]"} db.Create(&user) // Retrieve all users var users []User db.Find(&users) // Print the retrieved users for _, user := range users { fmt.Println(user.ID, user.Name, user.Email) } } ```
NoSQL Databases in Go
NoSQL databases are a popular choice for unstructured or semi-structured data storage. Go provides several libraries for connecting to and interacting with NoSQL databases. Let's explore a couple of options:
1. MongoDB
MongoDB is a widely-used NoSQL database, and the official MongoDB Driver for Go provides a straightforward way to connect and work with MongoDB databases using Go.
```go import ( "go.mongodb.org/mongo-driver/mongo" "go.mongodb.org/mongo-driver/mongo/options" ) func main() { // Set up the MongoDB client clientOptions := options.Client().ApplyURI("mongodb://localhost:27017") client, err := mongo.Connect(context.Background(), clientOptions) if err != nil { log.Fatal(err) } // Close the MongoDB client when finished defer func() { if err = client.Disconnect(context.Background()); err != nil { log.Fatal(err) } }() // Access a database and collection database := client.Database("mydb") collection := database.Collection("mycollection") // Insert a document into the collection document := bson.D{{"name", "John Doe"}, {"age", 30}} _, err = collection.InsertOne(context.Background(), document) if err != nil { log.Fatal(err) } // Find documents in the collection cursor, err := collection.Find(context.Background(), bson.M{}) if err != nil { log.Fatal(err) } // Iterate over the cursor and print documents defer cursor.Close(context.Background()) for cursor.Next(context.Background()) { var result bson.M if err := cursor.Decode(&result); err != nil { log.Fatal(err) } fmt.Println(result) } } ```
2. Redis
Redis is an in-memory data structure store that supports various data types. For using Redis with Go, you can use the popular Redis client library called "go-redis". It provides a simple and idiomatic way to work with Redis databases in Go.
```go import ( "github.com/go-redis/redis/v8" ) func main() { // Create a new Redis client client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", DB: 0, }) // Close the Redis client when finished defer client.Close() // Set a key-value pair err := client.Set(context.Background(), "key", "value", 0).Err() if err != nil { log.Fatal(err) } // Get the value of a key value, err := client.Get(context.Background(), "key").Result() if err != nil { log.Fatal(err) } fmt.Println(value) } ```
Best Practices for Database Connectivity
When working with databases in Go, it's essential to keep some best practices in mind:
1. Use Connection Pooling
For performance and scalability reasons, it's advisable to use connection pooling when connecting to databases. Connection pooling allows you to reuse existing database connections instead of creating a new one for each request, reducing the overhead of connection establishment.
2. Parameterize Queries
Always use parameterized queries or prepared statements to prevent SQL injection attacks and ensure the security and integrity of your data.
3. Error Handling
Proper error handling is crucial when working with databases. Always check and handle errors returned by database operations to avoid unexpected behavior and ensure data consistency.
4. Maintain Consistent Naming and Data Types
Use consistent naming conventions for tables, columns, and indexes to improve code readability and maintainability. Additionally, ensure that your application and database handle data types consistently to prevent issues related to data conversions.
Conclusion
Go provides robust support for connecting and working with both SQL and NoSQL databases. By leveraging libraries like database/sql
and GORM, you can easily connect to SQL databases and perform queries. Similarly, libraries like the MongoDB Driver for Go and "go-redis" allow you to work with NoSQL databases seamlessly.
Remember to follow best practices, such as using connection pooling, parameterizing queries, and handling errors properly, to ensure efficient and secure database connectivity in your Go applications.
Now that you are familiar with the options available for connecting to SQL and NoSQL databases in Go, you can start building powerful and data-driven applications. Happy coding!