Go by Example: Variables
Learn the fundamentals of variables in Go programming. Understand how to declare, initialize, assign values, and work with variables effectively.
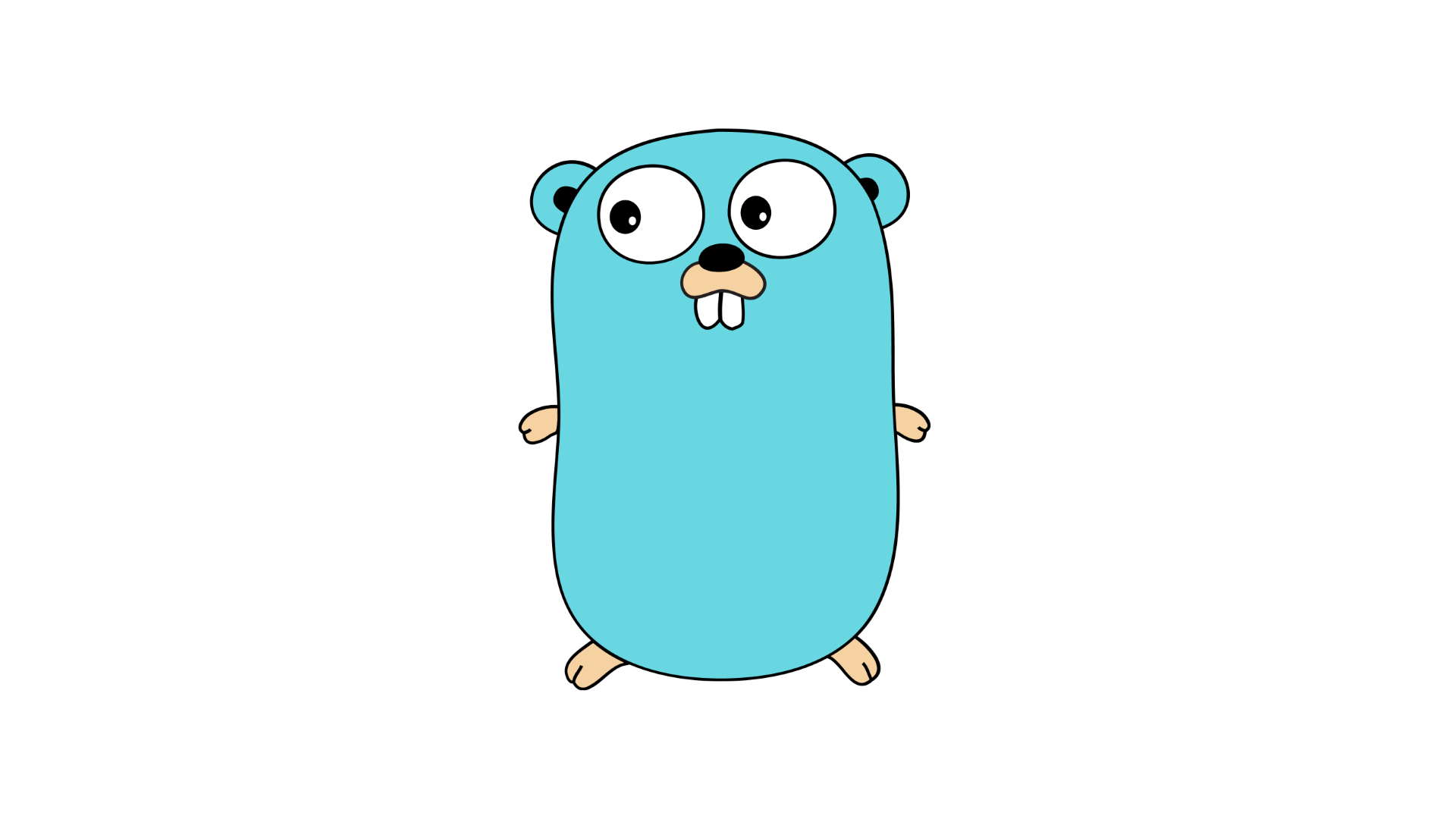
Introduction
In Go programming, variables play a fundamental role in storing and manipulating data. Understanding how to declare and use variables is essential for every Go developer. In this article, we'll explore the concept of variables in Go and learn how to declare and initialize them. By the end of this tutorial, you'll have a solid understanding of Go variables and how to work with them effectively.
Declaring Variables
Before using a variable in Go, we must declare it. A variable declaration tells the compiler the name and type of the variable we want to use. Here's the syntax for declaring variables in Go:
var variableName type
Let's declare a few variables of different types:
// Integer variable
var age int
// String variable
var name string
// Boolean variable
var isStudent bool
Initializing Variables
After declaring a variable, we can initialize it with a value. Initializing a variable assigns an initial value to it at the time of declaration. Here's the syntax for initializing variables in Go:
var variableName type = value
Let's declare and initialize some variables:
// Integer variable with initial value
var age int = 25
// String variable with initial value
var name string = "John Doe"
// Boolean variable with initial value
var isStudent bool = true
Go also supports a shorthand syntax for initialization called the "short variable declaration" or the ":=" operator. It allows us to declare and initialize a variable in a single line without explicitly specifying the type:
// Integer variable with initial value using short variable declaration
age := 25
// String variable with initial value using short variable declaration
name := "John Doe"
// Boolean variable with initial value using short variable declaration
isStudent := true
The Go compiler infers the type of the variable from the assigned value when using the ":=" operator.
Assigning Values to Variables
Once a variable is declared and initialized, we can assign new values to it using the assignment (=) operator. Here's an example:
// Declaring and initializing a variable
var age int = 25
// Assigning a new value to the age variable
age = 30
We can also perform various operations on variables during assignment:
// Perform arithmetic operations during assignment
x := 5
y := 10
sum := x + y
// Concatenate strings during assignment
firstName := "John"
lastName := "Doe"
fullName := firstName + " " + lastName
Redeclaring Variables
In Go, we can redeclare a variable within the same block, but we must use it:
var x int = 5
var x int = 10 // Error: x already declared
However, we can redeclare variables in different blocks:
var x int = 5
if true {
var x int = 10 // No error: x declared in a different block
}
Zero Values
In Go, if we declare a variable without initializing it explicitly, it is assigned a "zero value". The zero value depends on the type of the variable:
0
for numeric types (integers, floating-point numbers)false
for booleans""
(empty string) for stringsnil
for pointers, functions, interfaces, slices, channels, and maps
Here's an example of zero values:
// Declaring variables without initializing them
var age int
var name string
var isStudent bool
fmt.Println(age) // Output: 0
fmt.Println(name) // Output: ""
fmt.Println(isStudent) // Output: false
Constants
In addition to variables, Go also supports constants. Constants are immutable values whose values cannot be changed once defined. To declare a constant in Go, we use the const
keyword:
const pi = 3.14
const (
monday = "Monday"
tuesday = "Tuesday"
wednesday = "Wednesday"
thursday = "Thursday"
friday = "Friday"
)
When declaring multiple constants in a block, we can use the const
keyword only for the first constant and omit it for the rest.
Conclusion
Congratulations! You now have a solid understanding of variables in Go. We covered declaring variables, initializing them, assigning values, redeclaring variables, zero values, and constants. Variables are essential building blocks in any programming language, and Go provides a robust set of tools for working with them.
Stay tuned for more articles in the "Go by Example" series, where we will explore other essential concepts and features of the Go programming language.