Go by Example: Multiple Return Values
Learn how to use multiple return values in Go. Explore their syntax, use cases, and benefits, including error handling. Improve your code's readability and modularity.
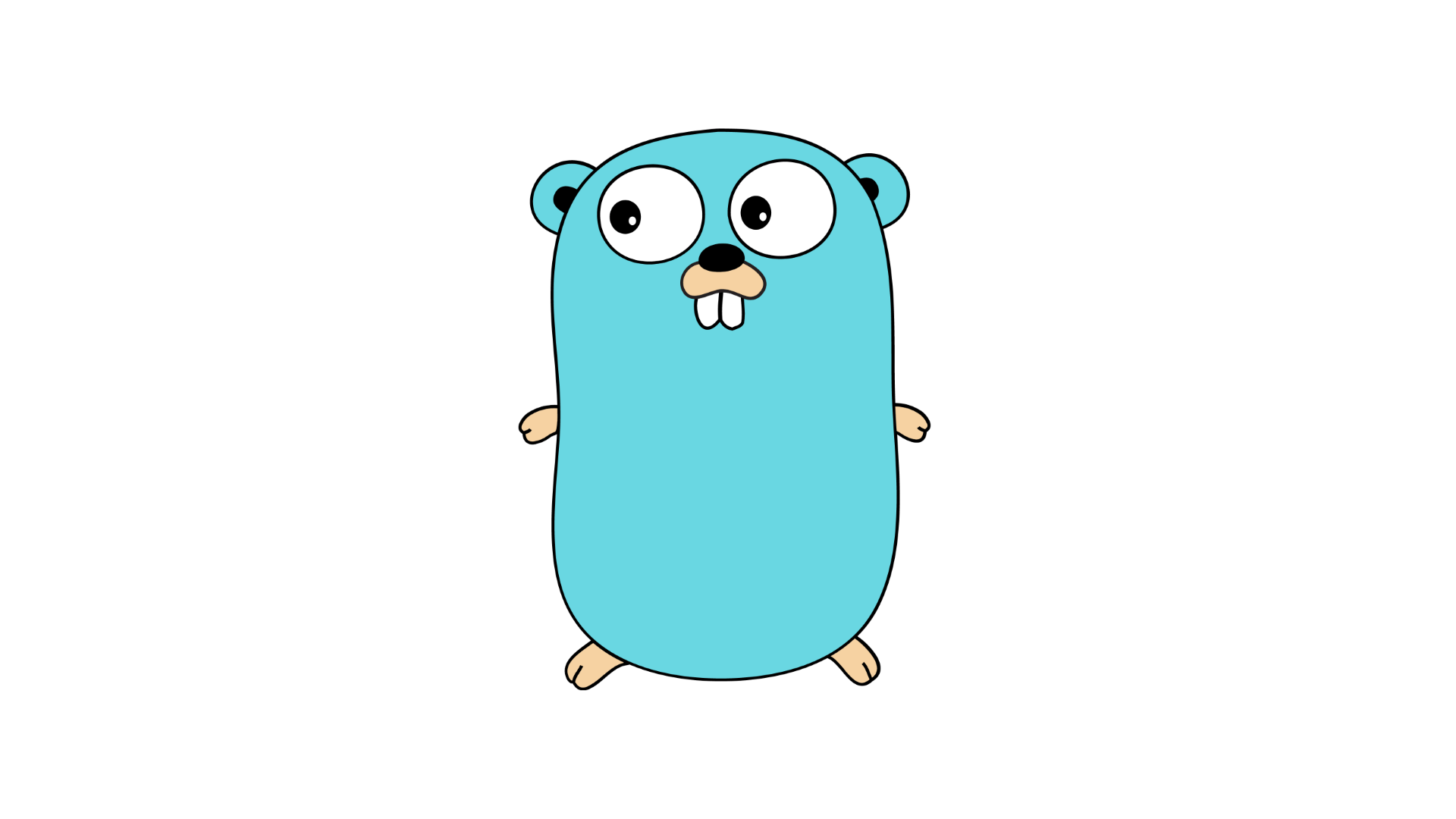
Introduction
In Go, it is common to have functions return multiple values. This powerful feature allows you to efficiently handle multiple pieces of related data in a single function call. In this tutorial, we will explore how to use multiple return values in Go and understand the benefits they provide. Let's dive in!
The Syntax for Multiple Return Values
In Go, returning multiple values from a function is as simple as specifying the return types as a comma-separated list in the function signature. Here's the basic syntax:
func functionName() (returnType1, returnType2, ...) {
// function body
return value1, value2, ...
}
Let's look at an example to see how this works in practice.
Example: Using Multiple Return Values
Suppose we have a function called divide
that takes two integers as input and returns the quotient and remainder. Here's how we can define the divide
function:
func divide(dividend, divisor int) (int, int) {
quotient := dividend / divisor
remainder := dividend % divisor
return quotient, remainder
}
To call this function and retrieve the multiple return values, we can do the following:
quot, rem := divide(10, 3)
fmt.Println("Quotient:", quot)
fmt.Println("Remainder:", rem)
The above code will output:
Quotient: 3
Remainder: 1
As you can see, we assigned the return values quot
and rem
to the results of the divide
function call. We could also use the blank identifier (_
) if we're not interested in one or more of the return values.
Returning Errors as Multiple Values
In Go, it is a common practice to return an error
as one of the multiple return values. This allows functions to indicate whether an error occurred during their execution.
Let's consider an example function called sqrt
that calculates the square root of a given number. If the number is negative, we want to return an error message. Here's how we can define the sqrt
function:
import (
"errors"
"math"
)
func sqrt(n float64) (float64, error) {
if n < 0 {
return 0, errors.New("math: square root of negative number")
}
return math.Sqrt(n), nil
}
We can use this function as follows:
result, err := sqrt(-10)
if err != nil {
fmt.Println(err)
} else {
fmt.Println("Square root:", result)
}
If the input value is negative, it will return an error message. Otherwise, it will return the square root of the number. You can customize the error message to suit your needs.
Using Blank Identifiers
As mentioned earlier, you can use the blank identifier (_
) if you are not interested in one or more of the return values. This is particularly useful when you only need to ignore certain values and focus on others.
For example, let's modify our sqrt
function to return both the square root and its inverse:
func sqrtInverse(n float64) (float64, float64, error) {
if n < 0 {
return 0, 0, errors.New("math: square root of negative number")
}
return math.Sqrt(n), 1 / math.Sqrt(n), nil
}
If we just want to get the inverse value and ignore the square root, we can use the blank identifier (_
):
_, inv, _ := sqrtInverse(16)
fmt.Println("Inverse:", inv)
In this case, we are using the blank identifier twice to ignore the square root value and the error. We can still handle the error in a separate block of code if needed, but here we are simply ignoring it.
Conclusion
By utilizing multiple return values in Go, you can enhance your code's readability, simplify error handling, and create more flexible and modular functions. Eagerly experiment with multiple return values in your own projects to experience the benefits firsthand.
Stay tuned for more articles in the "Go by Example" series, where we will continue exploring the essential features and concepts of the Go programming language. Happy coding!