Zero Allocation and Memory Efficiency: Golang's Unique Approach
Go's memory efficiency sets it apart from other languages. With stack allocation, escape analysis, and string interning, Go minimizes allocations and improves performance. Learn how it works!
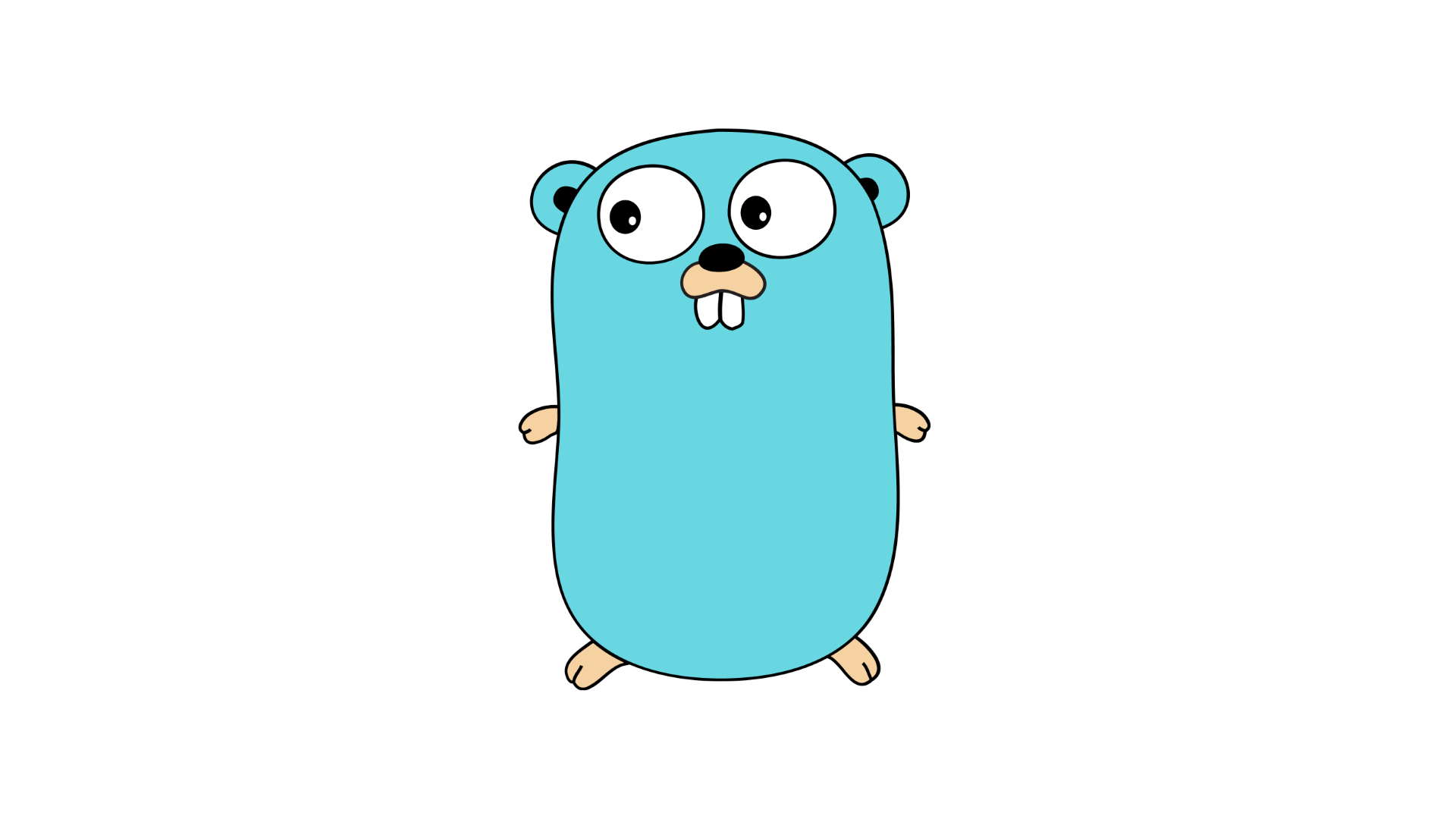
Introduction
When it comes to memory efficiency and performance optimization, Go (Golang) sets itself apart from other programming languages. Go's unique approach to memory allocation ensures efficient memory usage and reduces unnecessary garbage collection.
In this blog post, we'll explore the concept of zero allocation and how Go achieves memory efficiency through stack allocation, escape analysis, and other memory management techniques.
What is Zero Allocation?
Zero allocation refers to the practice of avoiding unnecessary memory allocations during program execution. In many programming languages, objects are frequently allocated on the heap, which requires additional memory and increases the workload on the garbage collector.
Go, on the other hand, aims to minimize memory allocations by utilizing stack allocation whenever possible. The idea is to allocate memory on the stack, which is faster and requires less overhead compared to heap allocation.
Stack Allocation in Go
Go uses stack allocation for small objects, typically those that fit within the size of a machine word. Stack allocation is fast because it only involves the adjustment of the stack pointer, without the need for complex memory management.
When a function is called, Go allocates memory for local variables and function arguments on the stack. Once the function returns, the memory is automatically reclaimed, making it a simple and efficient process.
Here's an example that demonstrates stack allocation in Go:
package main
import "fmt"
func main() {
x := 10
y := 20
z := add(x, y)
fmt.Println(z)
}
func add(a, b int) int {
return a + b
}
In this example, the variables x
, y
, and z
are allocated on the stack within the main
function. These variables are automatically deallocated when the function returns.
Escape Analysis
To determine whether an object can be allocated on the stack or must be allocated on the heap, Go uses escape analysis. Escape analysis analyzes the lifetime of objects and determines whether they can safely be allocated on the stack without causing issues.
If an object is determined to have a longer lifetime or if it escapes by returning from a function, Go allocates it on the heap instead. This ensures that the object persists even after the function has finished executing.
Here's an example that demonstrates escape analysis in Go:
package main
import "fmt"
func main() {
fmt.Println(createPerson("John", "Doe"))
}
func createPerson(firstName, lastName string) *Person {
p := Person{
firstName: firstName,
lastName: lastName,
}
return &p
}
type Person struct {
firstName string
lastName string
}
In this example, the createPerson
function creates a new Person
struct and assigns values to its fields. Since the Person
struct is returned from the function, Go performs escape analysis and determines that it should be allocated on the heap.
String Interning
Go implements a string interning technique to optimize memory usage for string values. String interning refers to the process of reusing memory for identical string values instead of allocating separate memory for each occurrence.
When you assign the same string value to multiple variables, Go internally stores the string value in a shared memory pool. It then references this shared memory pool for all the variables that contain the same string value.
This can significantly reduce memory usage, especially when dealing with large strings or when the same string value is repeated multiple times within an application.
Here's an example that demonstrates string interning in Go:
package main
import "fmt"
func main() {
s1 := "Hello, World!"
s2 := "Hello, World!"
fmt.Println(s1 == s2) // true
fmt.Println(&s1 == &s2) // true
}
In this example, both s1
and s2
contain the same string value. Go internally stores this value in a shared memory pool and references it for both variables. This is why s1 == s2
and &s1 == &s2
both return true
.
Why Memory Efficiency Matters
Memory efficiency is crucial for optimizing application performance, especially in scenarios where resources are limited or when dealing with large-scale systems.
By minimizing memory allocations and reducing unnecessary garbage collection, Go improves application responsiveness and reduces memory overhead. It allows developers to build efficient, high-performance systems and services that can handle a large number of concurrent requests.
Conclusion
Go's unique approach to memory efficiency sets it apart from other programming languages. By leveraging techniques such as stack allocation, escape analysis, and string interning, Go minimizes memory allocations and reduces unnecessary garbage collection.
Developers can take advantage of Go's memory efficiency to build high-performance applications that consume fewer resources and deliver improved responsiveness.
Understanding and applying these memory management techniques in Go can greatly benefit your code and unlock the true potential of the language.
Keep experimenting and exploring Go's memory efficiency features to build robust and efficient applications!