Go by Example: Interfaces
Interfaces in Go define a set of method signatures, enabling polymorphism and enhancing code reusability. Learn how interfaces work and how to implement and use them effectively in Go.
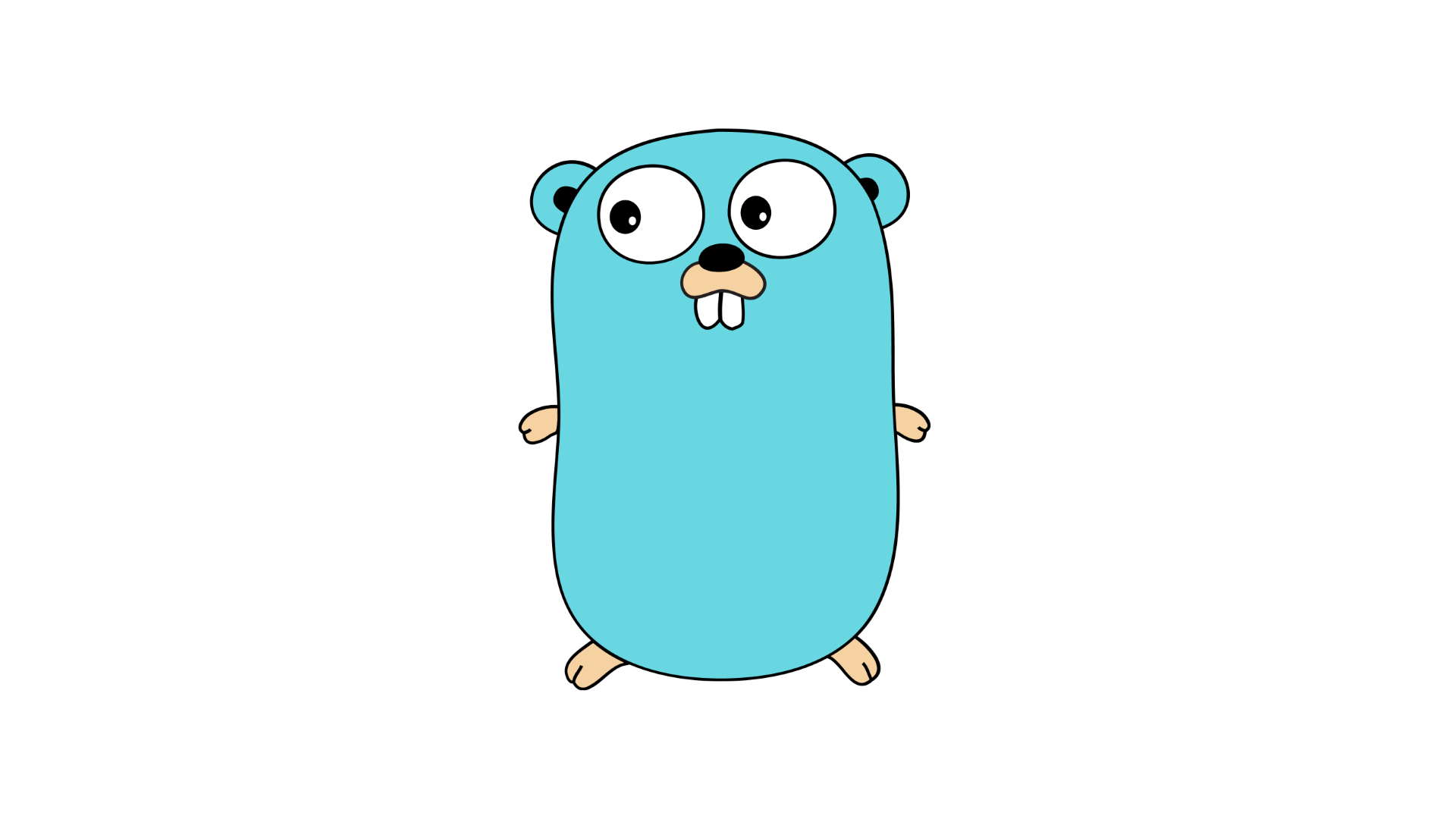
Introduction
Welcome to the next installment of our "Go by Example" series! In this tutorial, we will explore the concept of interfaces in Go. Interfaces play a crucial role in Go's type system, enabling polymorphism and enhancing code reusability. Whether you are a beginner or an experienced Go developer, understanding interfaces will help you write cleaner and more modular code. Let's dive in!
What Are Interfaces?
In simple terms, an interface in Go defines a set of method signatures. It specifies what a type can do but does not provide any implementation details. Any type that implements all the methods of an interface implicitly satisfies that interface.
Let's understand this with an example. Consider a scenario where we have different shapes: a square, a circle, and a rectangle. Each shape needs to have a method that calculates its area. Instead of defining separate methods for each shape, we can define an interface called "Shape" with a method signature for calculating area:
type Shape interface {
Area() float64
}
Now, any type that implements the "Area()" method will implicitly satisfy the "Shape" interface. This enables us to write functions that work with any shape type that satisfies the "Shape" interface, as we'll see later in this tutorial.
Implementing the Interface
To implement an interface, a type must provide the necessary method implementations. Let's continue with our shape example and implement the "Shape" interface for a square type:
type Square struct {
side float64
}
func (s Square) Area() float64 {
return s.side * s.side
}
In the above code, we define a struct type called "Square" with a single field called "side." We also define the "Area()" method for the "Square" type, which calculates the area of the square. Since the "Square" type implements the "Area()" method, it implicitly satisfies the "Shape" interface.
Similarly, we can implement the "Shape" interface for other shape types like circle and rectangle. Each type can provide its own implementation of the "Area()" method:
type Circle struct {
radius float64
}
func (c Circle) Area() float64 {
return math.Pi * c.radius * c.radius
}
type Rectangle struct {
length, width float64
}
func (r Rectangle) Area() float64 {
return r.length * r.width
}
Using Interfaces
Now that we have implemented the "Shape" interface for different shape types, let's see how we can use interfaces to write more versatile code.
Consider a function called "PrintArea()" that takes a shape as an argument and prints its area:
func PrintArea(s Shape) {
fmt.Println("Area:", s.Area())
}
Since the "PrintArea()" function expects a parameter of type "Shape", we can pass any shape type that satisfies the "Shape" interface:
square := Square{side: 4}
circle := Circle{radius: 3}
rectangle := Rectangle{length: 5, width: 3}
PrintArea(square) // Prints "Area: 16"
PrintArea(circle) // Prints "Area: 28.274333882308138"
PrintArea(rectangle) // Prints "Area: 15"
As you can see, the "PrintArea()" function can work with any shape type that implements the "Shape" interface. This polymorphic behavior allows us to write generic functions that can operate on different types as long as they satisfy a common interface.
Empty Interfaces
In addition to regular interfaces, Go also has a special type called the empty interface. The empty interface is denoted by the type "interface{}". It can represent any type because it has zero methods:
var x interface{}
x = 42 // x can hold any value
x = "Go by Example" // x can hold any value
x = []int{1, 2, 3} // x can hold any value
Empty interfaces are commonly used when we want to work with values of different types without specifying the exact type. For instance, the "fmt.Println()" function takes empty interfaces as arguments, allowing us to print values of any type:
fmt.Println(42) // Prints "42"
fmt.Println("Go by Example") // Prints "Go by Example"
fmt.Println([]int{1, 2, 3}) // Prints "[1 2 3]"
While empty interfaces provide flexibility, excessive use of empty interfaces can lead to less readable and maintainable code. Therefore, it's important to use them judiciously and with clear intent.
Interface Composition
Go allows us to compose interfaces by embedding one or more interfaces within another. This feature enables us to create complex interfaces by combining smaller reusable interfaces.
Let's extend our shape example to include another interface called "Resizable" that defines a method for resizing a shape:
type Resizable interface {
Resize(factor float64)
}
We can then compose the "Shape" interface and the "Resizable" interface to create a new interface called "ResizableShape":
type ResizableShape interface {
Shape
Resizable
}
The "ResizableShape" interface now requires any type that satisfies it to provide the methods of both the "Shape" interface and the "Resizable" interface. This allows us to write functions that work with shapes that are both resizable and have an area:
func PrintResizableShapeInfo(rs ResizableShape) {
fmt.Println("Area:", rs.Area())
rs.Resize(2)
}
By using interface composition, we enforce a contract that any type satisfying the "ResizableShape" interface must provide implementations for both the "Shape" methods and the "Resizable" methods.
Interface Nil Assertions
In Go, you can assert whether an interface value is nil or non-nil using the syntax: "value, ok := interfaceValue.(Type)".
Let's consider a scenario where we want to calculate the area of a shape if it is resizable, but ignore it if it isn't. We can achieve this using interface nil assertions:
func CalculateArea(shape Shape) float64 {
if resizable, ok := shape.(Resizable); ok {
resizable.Resize(2)
}
return shape.Area()
}
In the above code, we attempt to assert whether the "shape" value satisfies the "Resizable" interface by assigning it to "resizable" and a boolean value "ok". If the assertion is successful (i.e., "ok" is true), we resize the shape by calling the "Resize()" method. Otherwise, we simply calculate the shape's area using the "Area()" method.
This technique allows us to gracefully handle cases where an interface value may or may not satisfy an additional interface, making our code more robust.
Wrapping Up
Congratulations! You now have a solid understanding of interfaces in Go. Interfaces provide a powerful mechanism for achieving polymorphism and code reusability. By defining interfaces and implementing them for different types, you can write more modular and flexible code.
Interfaces are an integral part of Go's type system, allowing you to leverage the benefits of static typing while enjoying the flexibility of dynamic languages.
We hope this tutorial has been informative and helpful. Stay tuned for more exciting topics in our ongoing "Go by Example" series!