Go by Example: Range
Learn how to use the range keyword in Go to iterate over arrays, maps, strings, and channels. Simplify your code and access elements with ease.
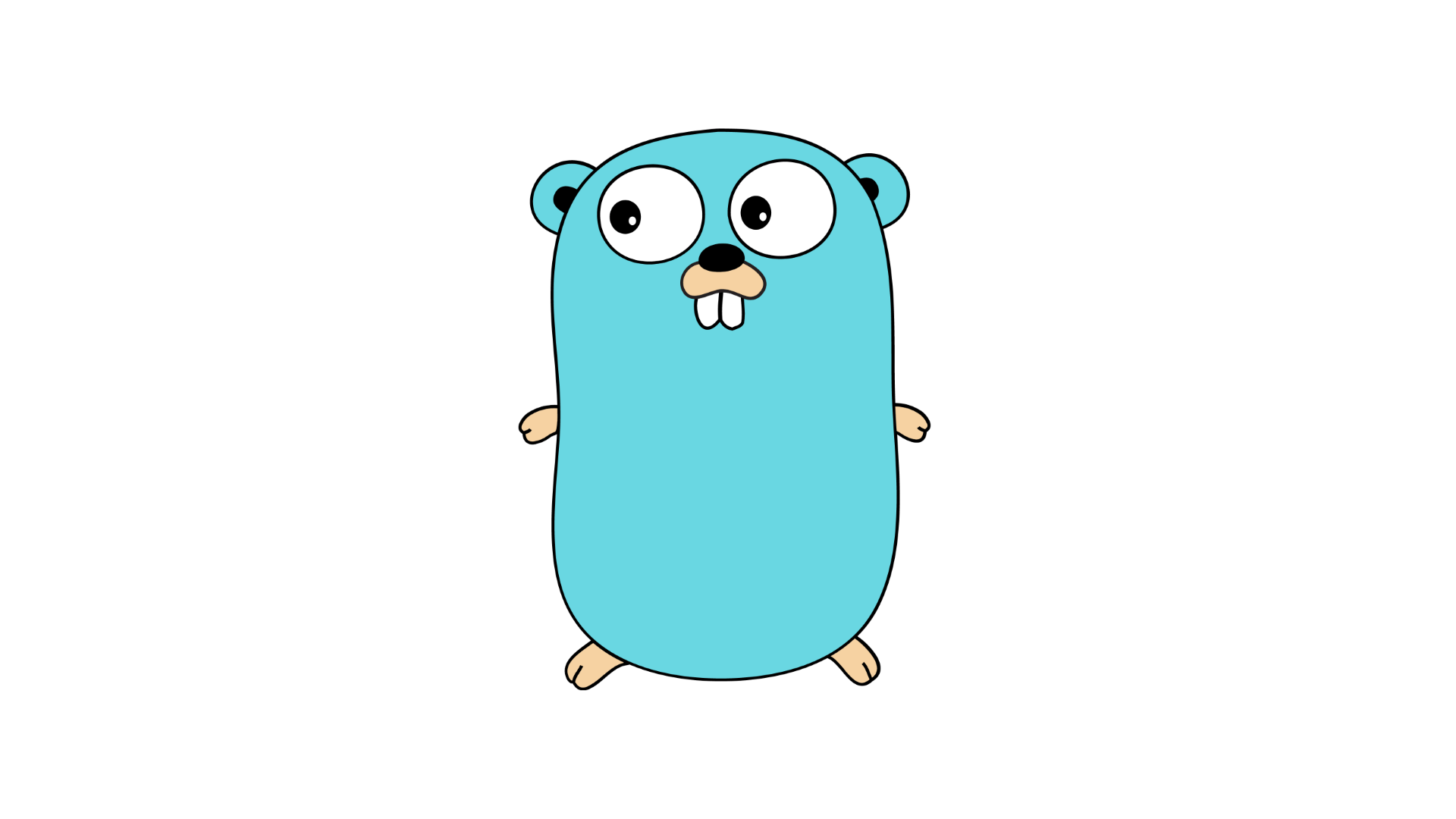
Introduction
Welcome to another installment of our Go by Example series! In this tutorial, we'll be exploring the range
keyword in Go. The range
keyword is a powerful construct that allows you to iterate over elements in a variety of data structures, such as arrays, slices, maps, channels, and more. It provides a clean and concise syntax for looping and accessing elements. Let's dive in and see how the range
keyword can simplify your code!
Iterating over Arrays and Slices
The range
keyword is commonly used to iterate over arrays and slices. It provides access to both the index and value of each element in the array or slice during the iteration process. Here's an example:
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5}
for index, value := range numbers {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
}
In the above example, we have an integer slice called numbers
containing five elements. We use the range
keyword within a for
loop to iterate over each element. Inside the loop, we print the index and value of each element using fmt.Printf
.
The output of this program will be:
Index: 0, Value: 1
Index: 1, Value: 2
Index: 2, Value: 3
Index: 3, Value: 4
Index: 4, Value: 5
As you can see, the range
keyword allows us to access both the index and value of each element, making it easy to perform operations on the elements during iteration.
Ignoring the Index or Value
Sometimes, you might only be interested in either the index or the value, and not both. In such cases, you can use the underscore (_
) to discard the unused variable. Here's an example:
package main
import "fmt"
func main() {
numbers := []int{1, 2, 3, 4, 5}
for _, value := range numbers {
fmt.Println(value)
}
}
In this example, we use the underscore (_
) to ignore the index variable. We only print the value of each element. The output will be:
1
2
3
4
5
By using the underscore (_
), we indicate to the Go compiler that we are not interested in storing or using the index variable. This can help improve the readability of our code and make it clear that the index is not needed for our specific use case.
Iterating over Maps
In addition to arrays and slices, you can also use the range
keyword to iterate over maps. When iterating over a map, the range
keyword returns the key and value of each key-value pair. Here's an example:
package main
import "fmt"
func main() {
ages := map[string]int{
"Alice": 25,
"Bob": 30,
"Charlie":35,
}
for name, age := range ages {
fmt.Printf("%s is %d years old\n", name, age)
}
}
In this example, we have a map called ages
that maps names to ages. We use the range
keyword to iterate over each key-value pair in the map. Inside the loop, we print the name and age of each person.
The output of this program will be:
Alice is 25 years old
Bob is 30 years old
Charlie is 35 years old
As you can see, the range
keyword makes it easy to iterate over the key-value pairs in a map and access both the key and value.
Iterating over Strings
The range
keyword can also be used to iterate over strings. When iterating over a string, the range
keyword returns the index and Unicode code point value of each character. Here's an example:
package main
import "fmt"
func main() {
message := "Hello, World!"
for index, runeValue := range message {
fmt.Printf("Index: %d, Rune Value: %U\n", index, runeValue)
}
}
In this example, we have a string called message
containing the text "Hello, World!". We use the range
keyword to iterate over each character in the string. Inside the loop, we print the index and Unicode code point value of each character using fmt.Printf
.
The output of this program will be:
Index: 0, Rune Value: U+0048
Index: 1, Rune Value: U+0065
Index: 2, Rune Value: U+006C
Index: 3, Rune Value: U+006C
Index: 4, Rune Value: U+006F
Index: 5, Rune Value: U+002C
Index: 6, Rune Value: U+0020
Index: 7, Rune Value: U+0057
Index: 8, Rune Value: U+006F
Index: 9, Rune Value: U+0072
Index: 10, Rune Value: U+006C
Index: 11, Rune Value: U+0064
Index: 12, Rune Value: U+0021
As you can see, the range
keyword provides access to both the index and Unicode code point value of each character in a string. This can be useful when working with Unicode strings and performing operations on individual characters.
Iterating over Channels
The range
keyword can also be used to iterate over channels in Go. When iterating over a channel, the range
keyword waits for values to be sent to the channel and returns them. Here's an example:
package main
import "fmt"
func generateNumbers(ch chan int) {
for i := 1; i <= 5; i++ {
ch <- i
}
close(ch)
}
func main() {
ch := make(chan int)
go generateNumbers(ch)
for value := range ch {
fmt.Println(value)
}
}
In this example, we have a function called generateNumbers
that sends the numbers 1 to 5 to a channel called ch
. We then use the range
keyword in the main
function to iterate over the values received from the channel and print them.
The output of this program will be:
1
2
3
4
5
As you can see, the range
keyword allows us to easily iterate over values sent to a channel, making it straightforward to process data as it becomes available.
Conclusion
Congratulations! You've learned about the power of the range
keyword in Go. We explored how to use it to iterate over arrays, slices, maps, strings, and channels, and saw how it simplifies the process of accessing and working with elements within these data structures.
The range
keyword is an essential tool in your Go toolkit, enabling you to write cleaner, more concise, and more efficient code. By leveraging the range
keyword, you can make your Go programs more expressive, readable, and effective in handling data.
I hope this tutorial has been helpful in understanding how to use the range
keyword in Go. Stay tuned for more Go by Example tutorials, where we'll continue exploring the language and its various features. Happy coding!