Go by Example: Struct Embedding
Struct embedding is a powerful feature allowing you to combine multiple structs, promoting code reuse and modular design. It's a flexible alternative to inheritance, enabling access to embedded struct fields and methods. With anonymous struct embedding, you can achieve complex data structures.
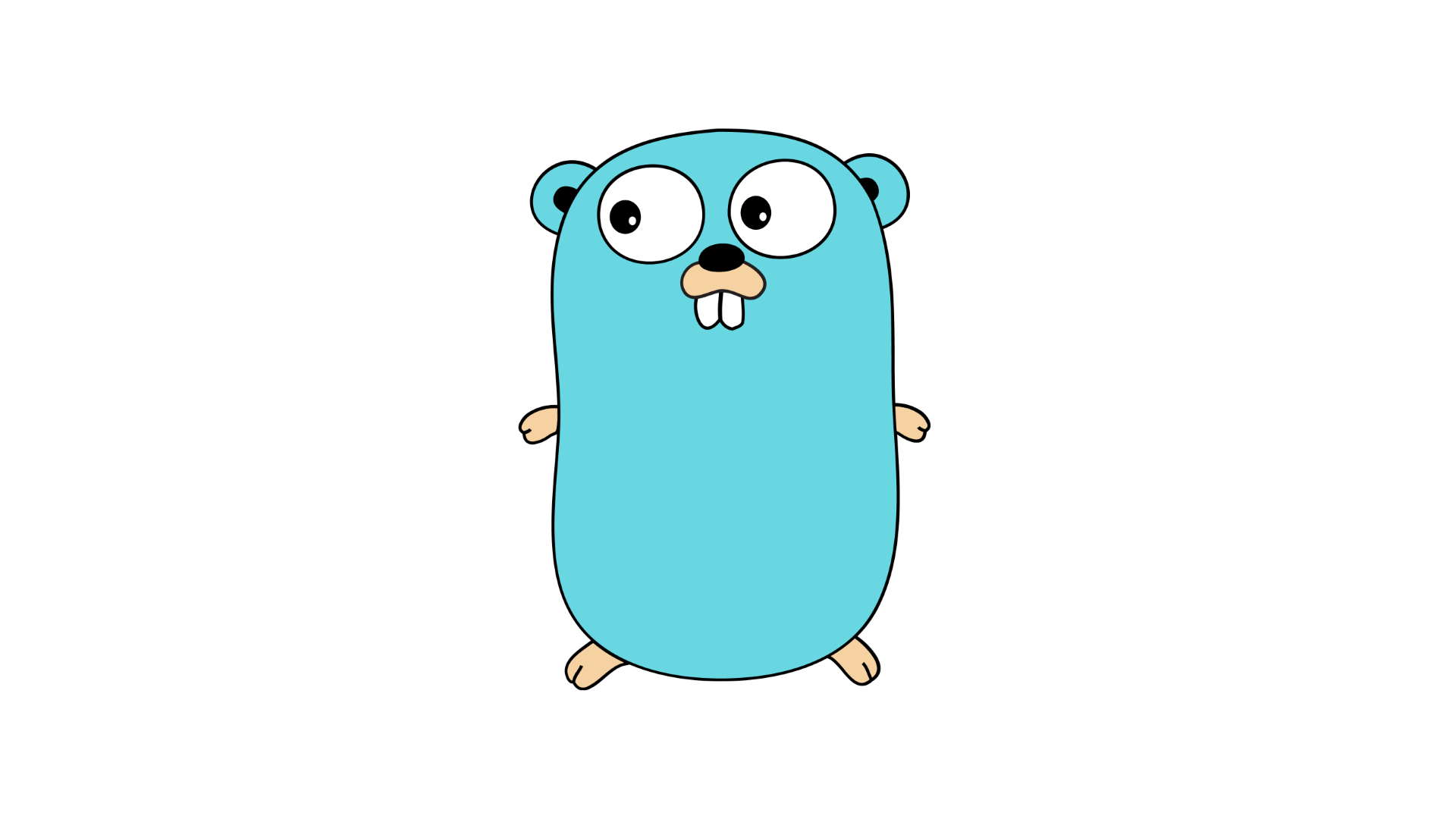
Go by Example: Struct Embedding
Welcome to another installment of our "Go by Example" series. In this tutorial, we'll explore the concept of struct embedding in Go. Struct embedding is a powerful feature that allows you to combine multiple structs into a single composite struct, enabling code reuse and promoting modular design. Let's dive in and learn more about struct embedding in Go!
Introduction
In Go, struct embedding allows you to include one struct (the embedded struct) into another struct (the embedding struct), effectively creating a relationship between the two. The embedding struct can access the fields and methods of the embedded struct, as if they were its own. This feature is similar to inheritance in object-oriented programming (OOP), but with some unique characteristics that make it particularly useful in Go.
Embedding Syntax
Let's start by understanding the syntax used for struct embedding in Go. Here's an example:
type Animal struct {
name string
}
type Dog struct {
Animal
breed string
}
In the example above, we have two structs: `Animal` and `Dog`. The `Dog` struct embeds the `Animal` struct by simply referencing it as a field. This syntax tells Go that the `Dog` struct includes all the fields and methods of the `Animal` struct.
Accessing Embedded Fields and Methods
Once a struct is embedded, you can access its fields and methods directly from the embedding struct. Let's take a look at an example:
func main() {
dog := Dog{
Animal: Animal{
name: "Buddy",
},
breed: "Labrador",
}
fmt.Println(dog.name) // Output: Buddy
}
In the example above, we create a new instance of the `Dog` struct and assign a name to it. Even though the `name` field is defined in the `Animal` struct, we can access it directly from the `Dog` struct.
Note that if the embedding struct and the embedded struct have fields with the same name, the field in the embedding struct takes precedence. This allows you to override the fields of the embedded struct when necessary.
Method Overriding
In addition to accessing fields, you can also override methods defined in the embedded struct. Let's see an example:
type Animal struct {
sound string
}
func (a *Animal) Speak() {
fmt.Println(a.sound)
}
type Dog struct {
Animal
sound string
}
func (d *Dog) Speak() {
fmt.Println("Woof!")
}
func main() {
dog := Dog{
Animal: Animal{
sound: "Roar",
},
sound: "Bark",
}
dog.Speak() // Output: Woof!
dog.Animal.Speak() // Output: Roar
}
In the example above, we have a method called `Speak` defined in both the `Animal` and `Dog` structs. When calling the `Speak` method on the `Dog` instance, it will invoke the method defined in the `Dog` struct, overriding the method in the embedded `Animal` struct. However, you can still access the method from the embedded struct by using the `..` syntax.
Embedding Multiple Structs
In Go, struct embedding also allows you to include multiple structs into a single embedding struct. This enables you to combine the fields and methods of multiple structs together. Let's see an example:
type Animal struct {
sound string
}
type Flyer struct {
maxAltitude int
}
type Bird struct {
Animal
Flyer
species string
}
func main() {
bird := Bird{
Animal: Animal{
sound: "Tweet",
},
Flyer: Flyer{
maxAltitude: 1000,
},
species: "Sparrow",
}
fmt.Println(bird.sound) // Output: Tweet
fmt.Println(bird.maxAltitude) // Output: 1000
}
In the example above, we have three structs: `Animal`, `Flyer`, and `Bird`. The `Bird` struct embeds both the `Animal` and `Flyer` structs, effectively combining their fields and methods into a single struct.
Anonymous Struct Embedding
In some cases, you may not want to give a name to the embedded struct. In such situations, you can use anonymous struct embedding. Here's an example:
type Employee struct {
name string
age int
}
type Manager struct {
Employee
teamSize int
}
func main() {
manager := Manager{
Employee{"John Smith", 35},
10,
}
fmt.Println(manager.name) // Output: John Smith
fmt.Println(manager.age) // Output: 35
}
In the example above, we have an `Employee` struct and a `Manager` struct. The `Manager` struct embeds the `Employee` struct anonymously. This means that the fields and methods of the `Employee` struct are directly accessible from the `Manager` struct.
When to Use Struct Embedding
Struct embedding is a powerful feature that promotes code reuse and modular design. Here are a few scenarios where struct embedding can be beneficial:
- Implementing inheritance-like behavior: Struct embedding allows you to achieve similar functionality to inheritance in OOP, without the complexities and limitations associated with traditional inheritance.
- Managing complex data structures: Embedding multiple structs into one makes it easier to manage and manipulate complex sets of data.
- Implementing interfaces: Embedding can help you implement multiple interfaces by combining the required methods into a single struct.
However, it's important to use struct embedding judiciously and avoid unnecessary complexity. Overusing embedding can make the code more difficult to understand and maintain.
Conclusion
Struct embedding is a powerful feature in Go, enabling code reuse and modular design. By including one or more structs within another, you can combine their fields and methods, creating a composite struct that benefits from the embedded structs' functionality. Struct embedding provides a flexible alternative to traditional inheritance and promotes cleaner, more modular code.
We hope this tutorial has given you a comprehensive understanding of struct embedding in Go. Stay tuned for more informative and practical tutorials in our "Go by Example" series. Happy coding!