Go by Example: Strings and Runes
In this tutorial, we'll explore strings and runes in Go, covering the basics as well as some advanced techniques. Learn how to manipulate strings, concatenate, find length, slice, and work with runes. Master these fundamental concepts to enhance your Go programming skills.
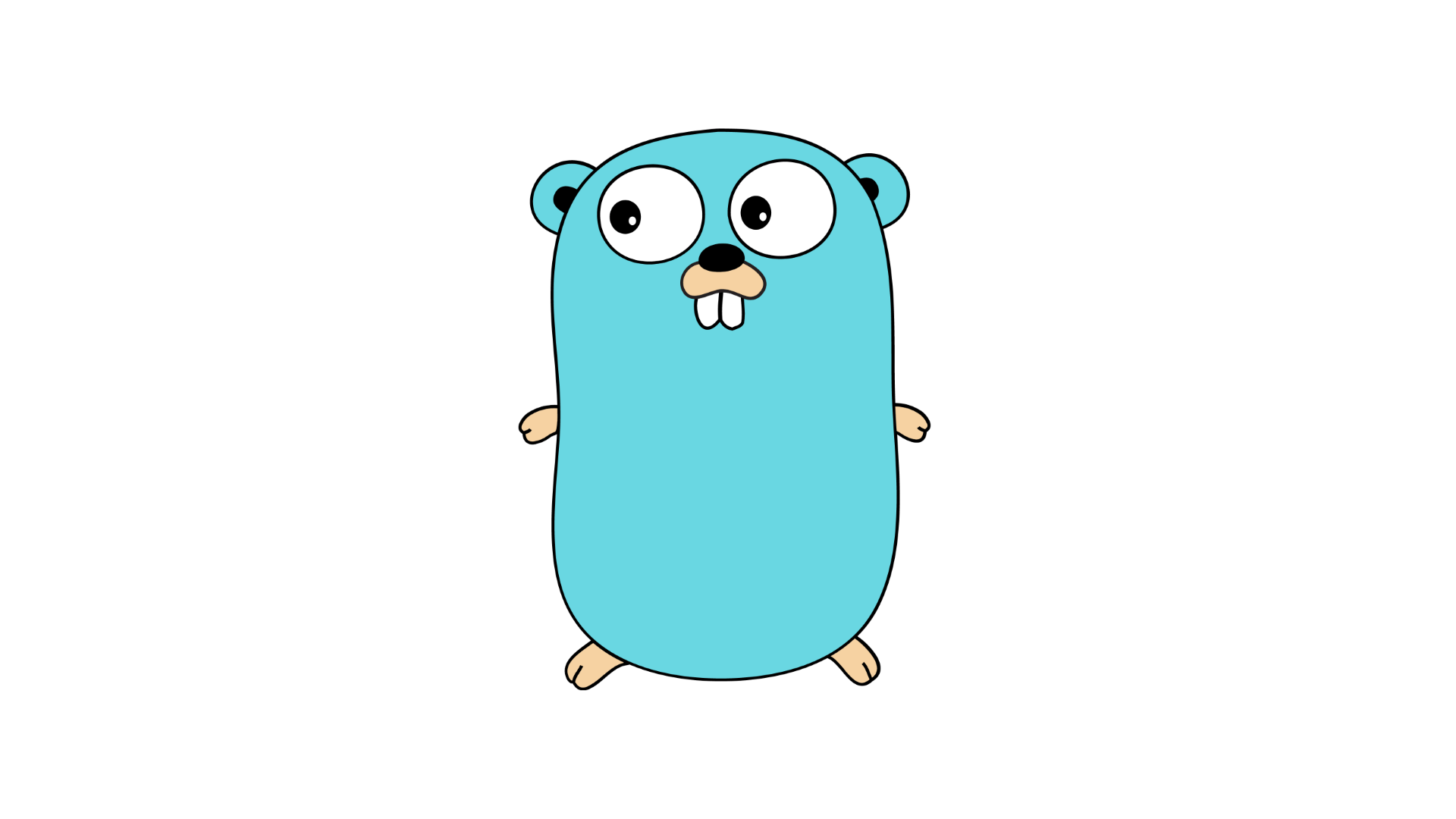
Go by Example: Strings and Runes
Go by Example: Strings and Runes
Welcome to another installment of our Go by Example series! In this tutorial, we'll explore strings and runes in Go, covering the basics as well as some advanced techniques. So let's dive in!
String Basics
In Go, a string is a sequence of one or more characters. It is represented using double quotes (e.g., "Hello, World!").
Here's an example of declaring and initializing a string variable:
package main
import "fmt"
func main() {
message := "Hello, World!"
fmt.Println(message)
}
Output:
Hello, World!
As you can see, we declare a variable called "message" and initialize it with the string "Hello, World!". Then we print the value of the "message" variable using the "fmt.Println" function.
String Operations
Go provides several operations for manipulating strings. Let's explore some of the most commonly used ones:
Concatenation
To concatenate two strings, you can use the "+" operator:
package main
import "fmt"
func main() {
firstName := "John"
lastName := "Doe"
fullName := firstName + " " + lastName
fmt.Println(fullName)
}
Output:
John Doe
String Length
To find the length of a string, you can use the "len" function:
package main
import (
"fmt"
"unicode/utf8"
)
func main() {
message := "Hello, World!"
fmt.Println(len(message))
fmt.Println(utf8.RuneCountInString(message))
}
Output:
13
13
The "len" function returns the number of bytes in the string, while "utf8.RuneCountInString" returns the number of characters or runes in the string.
String Slicing
To extract a portion of a string, you can use slicing:
package main
import "fmt"
func main() {
message := "Hello, World!"
fmt.Println(message[:5]) // Output: Hello
fmt.Println(message[7:]) // Output: World!
fmt.Println(message[7:12]) // Output: World
}
Working with Runes
In Go, a rune represents a Unicode code point. It can represent any character, including emojis and special symbols. To declare a rune, you can use single quotes (e.g., 'a', '♥').
Here's an example that prints the Unicode value of each character in a string:
package main
import "fmt"
func main() {
message := "Hello, 你好, नमस्ते"
for _, char := range message {
fmt.Printf("Character: %c, Unicode: %U\n", char, char)
}
}
Output:
Character: H, Unicode: U+0048
Character: e, Unicode: U+0065
Character: l, Unicode: U+006C
Character: l, Unicode: U+006C
Character: o, Unicode: U+006F
Character: ,, Unicode: U+002C
Character: 你, Unicode: U+4F60
Character: 好, Unicode: U+597D
Character: ,, Unicode: U+002C
Character: न, Unicode: U+0928
Character: म, Unicode: U+092E
Character: स, Unicode: U+0938
Character: ्, Unicode: U+094D
Character: त, Unicode: U+0924
Character: े, Unicode: U+0947
As you can see, the "range" keyword is used to iterate over each character in the string. The "%c" format specifier is used to print the character, and "%U" is used to print the Unicode value of the character.
Conclusion
Congratulations on learning about strings and runes in Go! You now have a solid foundation for working with strings and manipulating them. Feel free to explore more string and rune operations in the Go documentation to enhance your skills.
Stay tuned for the next part of our Go by Example series, where we'll cover more exciting topics!
Happy coding!