Go by Example: Methods
Learn how to define and use methods in Go. Methods allow you to encapsulate behavior within objects, making your code more organized and reusable. Explore pointer receivers, difference between methods and functions, and more. Take your Go programming skills to the next level!
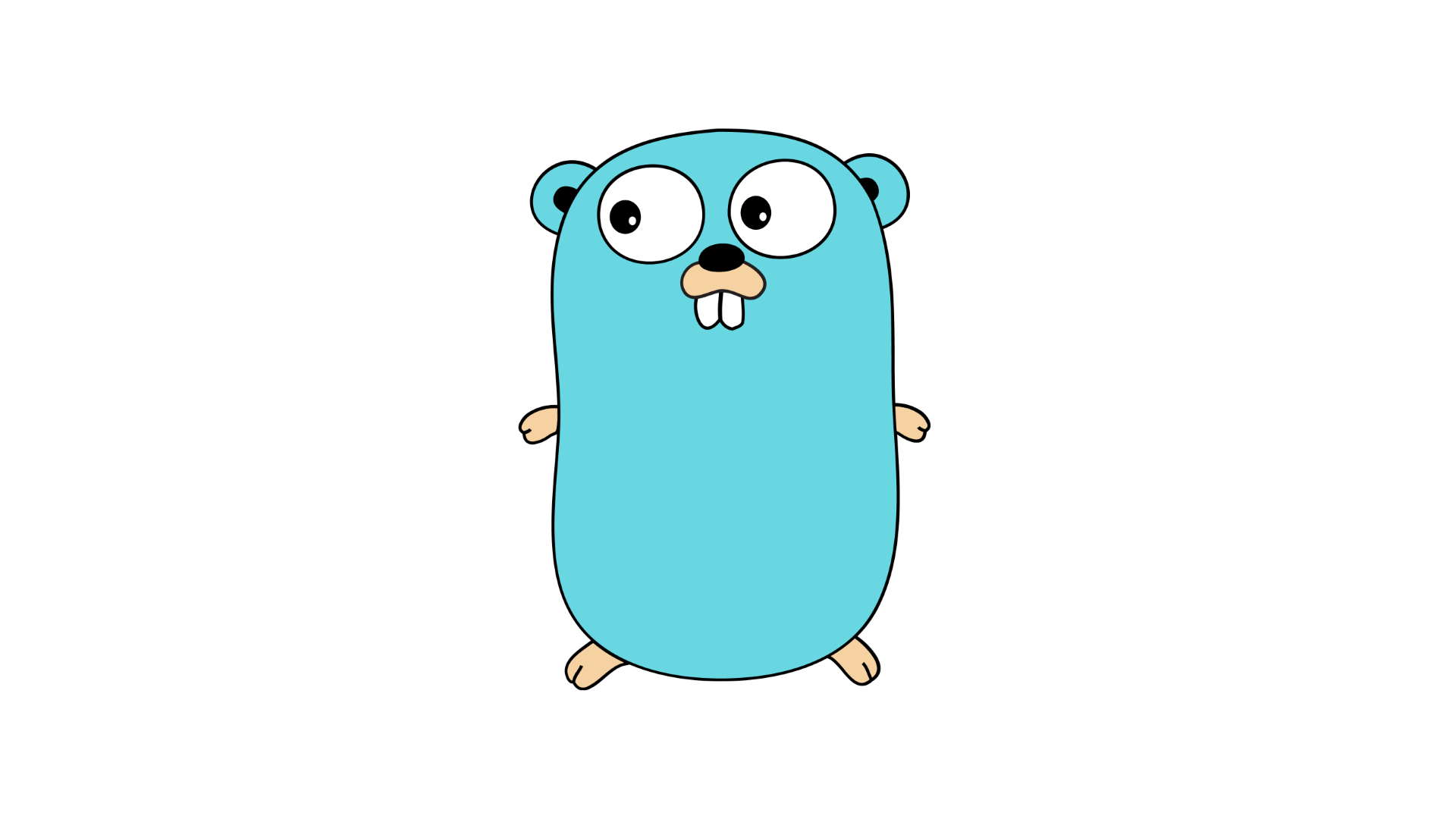
Go by Example: Methods
Welcome to another installment of our "Go by Example" series! Today, we will be diving into the wonderful world of methods in Go. Methods are an important aspect of any object-oriented programming language, and Go is no exception. By understanding how to define and use methods in Go, you can take your programming skills to the next level.
Introduction
In Go, a method is a function that operates on a specific type. It allows you to define behavior that is associated with a given type, enabling you to perform operations and manipulations on objects of that type. Methods provide a way to encapsulate functionality within the objects they belong to, making your code more organized and reusable.
Defining Methods
Defining a method in Go is straightforward. You start with the keyword func
followed by the receiver and the method name. The receiver is a parameter that specifies the type on which the method is defined. Let's take a look at an example:
type Rectangle struct {
width float64
height float64
}
// Method with a receiver of type Rectangle
func (r Rectangle) Area() float64 {
return r.width * r.height
}
In the above code snippet, we define a type called Rectangle
that has two properties: width
and height
. We then define a method called Area
that calculates and returns the area of the rectangle. The receiver r
of type Rectangle
allows us to access the properties of the object within the method.
Using Methods
Once we have defined a method, we can use it on objects of the corresponding type. Let's continue with our Rectangle
example:
rect := Rectangle{width: 10, height: 5}
area := rect.Area()
fmt.Println("Area of the rectangle:", area)
In the above code snippet, we create a new object of type Rectangle
called rect
with a width of 10 and a height of 5. We then call the Area
method on the rect
object to calculate its area and assign it to the variable area
. Finally, we print out the calculated area using the fmt.Println
function.
Pointer Receivers
In Go, we also have the option to define methods with pointer receivers. When a method has a pointer receiver, it can modify the object it operates on. Let's modify our Rectangle
example:
type Rectangle struct {
width float64
height float64
}
// Method with a pointer receiver
func (r *Rectangle) Scale(factor float64) {
r.width = r.width * factor
r.height = r.height * factor
}
In the above code snippet, we redefine the Rectangle
type and add a method called Scale
that takes a factor
parameter. By using a pointer receiver *Rectangle
, the Scale
method can modify the properties of the Rectangle
object it operates on.
Let's see how we can use this new method:
rect := Rectangle{width: 10, height: 5}
rect.Scale(2)
fmt.Println("Scaled Rectangle:", rect)
In the above code snippet, we create a Rectangle
object called rect
and call the Scale
method on it with a factor of 2. This modifies the width
and height
properties of the rect
object. Finally, we print out the modified rect
object using the fmt.Println
function.
Methods vs Functions
You might be wondering, what's the difference between methods and functions in Go? While both methods and functions allow you to define reusable blocks of code, methods are associated with specific types and can be called on objects of those types. Functions, on the other hand, can be called independently and are not tied to any specific type.
Here's a quick example to illustrate the difference:
type Circle struct {
radius float64
}
// Method with a receiver of type Circle
func (c Circle) Area() float64 {
return math.Pi * c.radius * c.radius
}
// Function that calculates the area of a circle
func CalculateArea(c Circle) float64 {
return math.Pi * c.radius * c.radius
}
In the above code snippet, we define a Circle
type with a method called Area
and a function called CalculateArea
. The method Area
can be called directly on Circle
objects, while the function CalculateArea
must be called and passed a Circle
object as an argument.
Wrapping Up
Congratulations! You now have a solid understanding of methods in Go. By using methods, you can encapsulate behavior within the objects they belong to, making your code more modular and reusable. Make sure to practice defining and using methods in your own Go programs to solidify your understanding.
Stay tuned for the next part of our "Go by Example" series, where we'll explore another interesting aspect of Go programming. Happy coding!