Go by Example: If/Else
In this edition, we will explore the "if/else" statement in Go. It allows us to execute different blocks of code based on certain conditions, making it a powerful tool for decision-making in programming. Let's dive right in and see how it works in Go!
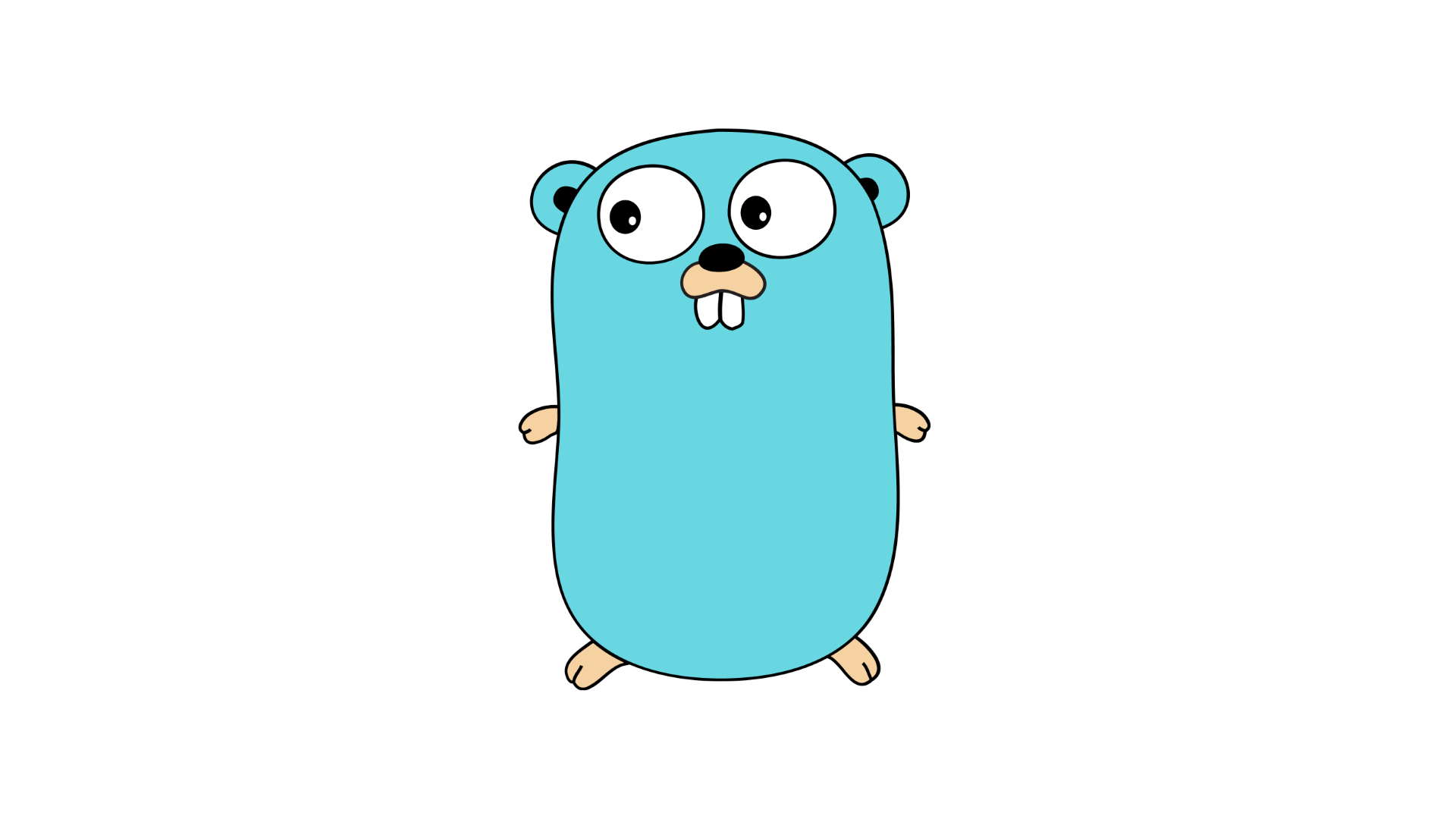
Go by Example: If/Else
Welcome to another exciting installment of our Go by Example series! In this edition, we will explore the "if/else" statement in Go.
The "if/else" statement is a fundamental control flow statement used to make decisions in programming. It allows us to execute different blocks of code based on certain conditions. Let's dive right in and see how it works in Go!
Basic Syntax
The basic syntax of the "if/else" statement in Go is as follows:
if condition {
// code toexecute if condition is true
} else {
// code to execute if condition is false
}
In this syntax, the "condition" is a boolean expression that evaluates to either true or false. If the condition is true, the code inside the first block is executed. If the condition is false, the code inside the else block is executed.
Example
Let's take a look at a simple example to better understand how the "if/else" statement works in Go:
package main
import "fmt"
func main() {
age := 25
if age >= 18 {
fmt.Println("You are an adult.")
} else {
fmt.Println("You are a minor.")
}
}
In this example, we have a variable called "age" with a value of 25. The "if" statement checks if the age is greater than or equal to 18. If it is, the message "You are an adult." is printed. Otherwise, the message "You are a minor." is printed.
When we run this program, the output will be:
You are an adult.
Since the "age" variable is 25, which is greater than 18, the condition evaluates to true, and the code inside the "if" block is executed.
Else If Construct
In addition to the "if" and "else" statements, Go also provides an "else if" construct that allows us to check multiple conditions. This construct is useful when we have more than two possible outcomes.
The syntax for the "else if" construct is as follows:
if condition1 {
// code to execute if condition1 is true
} else if condition2 {
// code to execute if condition2 is true
} else {
// code to execute if both conditions are false
}
In this syntax, the "condition1" is checked first. If it is true, the code inside the first block is executed. If it is false, the "condition2" is checked. If it is true, the code inside the second block is executed. If both conditions are false, the code inside the else block is executed.
Example
Let's modify our previous example to include an "else if" construct:
package main
import "fmt"
func main() {
age := 25
if age < 18 {
fmt.Println("You are a minor.")
} else if age >= 18 && age < 60 {
fmt.Println("You are an adult.")
} else {
fmt.Println("You are a senior citizen.")
}
}
In this example, we have added an additional condition to check if the age is above 60. If it is, the message "You are a senior citizen." will be printed. Otherwise, the message "You are an adult." will be printed.
When we run this program, the output will be:
You are an adult.
Since the "age" variable is 25, which is in the range of 18 to 60, the second condition evaluates to true, and the code inside the second block is executed.
Conclusion
The "if/else" statement is a powerful tool for making decisions in Go. It allows us to control the flow of our programs based on specific conditions. By understanding the basic syntax and how to use the "else if" construct, you can write more sophisticated and flexible programs.
Stay tuned for the next installment of our Go by Example series, where we will explore another important topic in Go programming. Happy coding!