Go by Example: For
In this article, we explore the versatile for loop in Go. We cover the basics, creating infinite loops, using the range keyword for iteration, nested loops, and controlling flow with break and continue statements. Mastering the for loop is essential for any Go programmer.
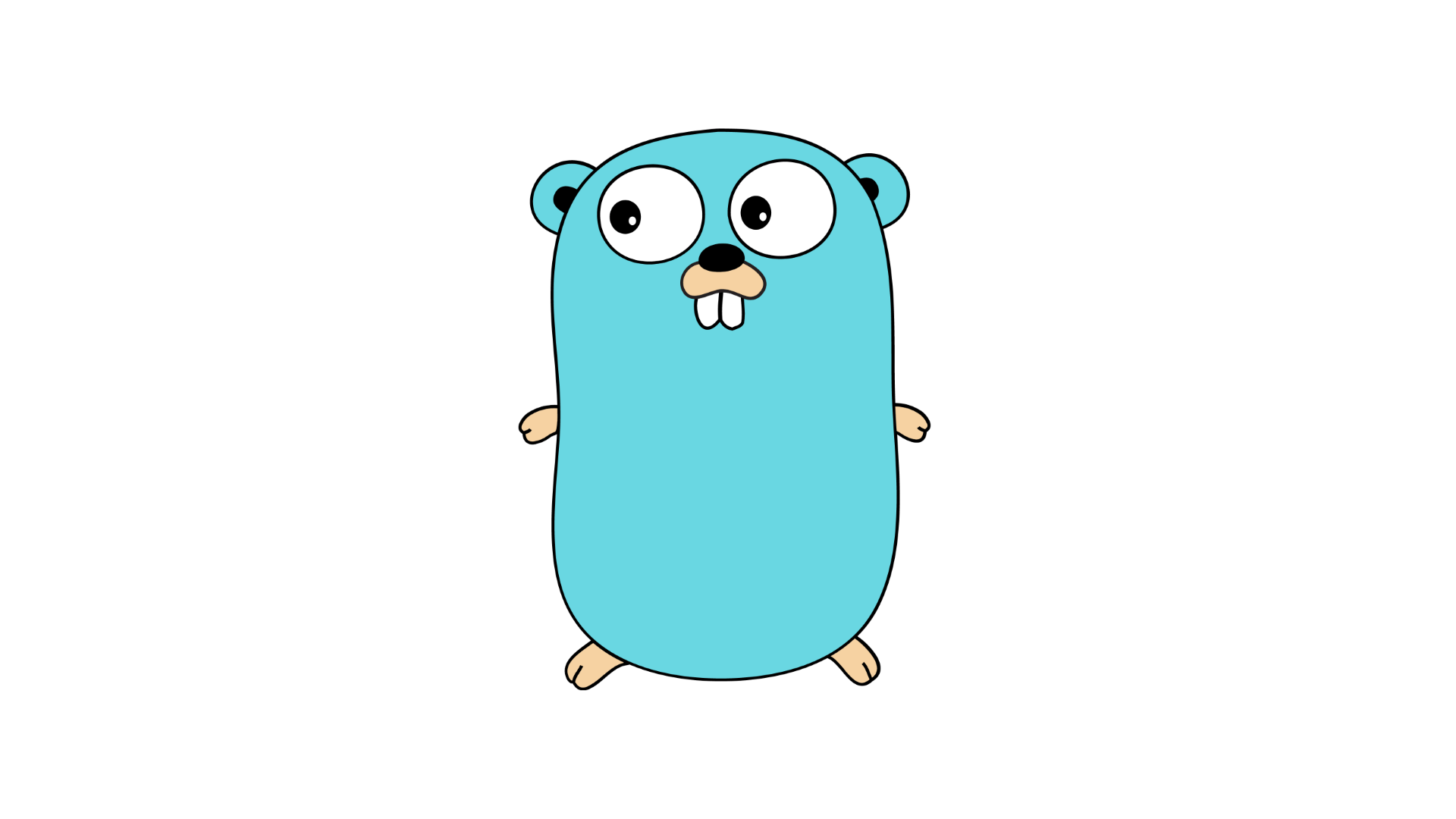
Go by Example: For
Welcome to another installment of our Go by Example series! In this article, we'll be diving into the usage and syntax of the for
loop in Go. The for
loop is one of the fundamental control flow constructs in any programming language, and Go provides a versatile and concise way to use it. Let's get started!
The Basics
The basic syntax for a for
loop in Go looks like this:
for initialisation; condition; post {
// Code to be executed
}
Let's break down each component of the for
loop:
- Initialisation: This is an optional statement that is executed before the loop starts. It typically initializes the loop counter or any other variables needed for the loop.
- Condition: The loop continues to execute as long as this condition is true. If the condition evaluates to false, the loop exits.
- Post: This statement is executed at the end of each iteration of the loop. It typically increments the loop counter or updates any other variables as needed.
Let's look at a simple example:
for i := 0; i < 5; i++ {
fmt.Println(i)
}
In this example, the loop will print the values 0 to 4. Let's break down the code:
i := 0
is the initialization statement. It declares a variablei
and initializes it to 0.i < 5
is the condition. As long asi
is less than 5, the loop will continue to execute.i++
is the post statement. It incrementsi
by 1 at the end of each iteration.fmt.Println(i)
is the code to be executed. It prints the value ofi
at each iteration.
The output of this code will be:
0
1
2
3
4
Infinite Loops
Go also allows you to create infinite loops using the for
loop construct when you omit the condition. For example:
for {
// Code to be executed
}
In this case, the code inside the loop will continue to execute indefinitely until you explicitly break out of the loop using the break
statement.
For Each Loop
Go does not have a built-in for each
loop like some other languages. However, you can achieve similar functionality using the range
keyword. The range
keyword allows you to iterate over elements in an array, slice, string, map, or channel.
Here's an example of how to use the range
keyword:
numbers := []int{1, 2, 3, 4, 5}
for index, value := range numbers {
fmt.Println("Index:", index, "Value:", value)
}
In this code, numbers
is a slice containing the numbers 1 to 5. The range
keyword is used to iterate over each element in the slice. On each iteration, the index and value of the current element are printed.
The output of this code will be:
Index: 0 Value: 1
Index: 1 Value: 2
Index: 2 Value: 3
Index: 3 Value: 4
Index: 4 Value: 5
The range
keyword can also be used with strings to iterate over each character in the string, with maps to iterate over key-value pairs, and with channels to iterate over values received from the channel.
Nested Loops
Go supports nested loops, which means you can have a for
loop inside another for
loop. This is useful when you need to iterate over multiple dimensions or perform repetitive actions within the outer loop.
Here's an example:
for i := 1; i <= 3; i++ {
for j := 1; j <= 3; j++ {
fmt.Println(i, j)
}
}
In this code, we have two nested for
loops. The outer loop iterates from 1 to 3, and the inner loop also iterates from 1 to 3. The output of this code will be:
1 1
1 2
1 3
2 1
2 2
2 3
3 1
3 2
3 3
As you can see, the nested loops allow you to systematically iterate over all possible combinations of the loop variables.
Break and Continue Statements
The break
statement is used to exit the loop prematurely. It is often used in conjunction with an if
statement to check for a certain condition and break out of the loop when that condition is met.
Here's an example:
for i := 1; i <= 5; i++ {
if i == 3 {
break
}
fmt.Println(i)
}
In this code, the if i == 3
condition checks if the current value of i
is equal to 3. If it is, the break
statement is executed, and the loop is exited. The output of this code will be:
1
2
The continue
statement is used to skip the rest of the current iteration and move on to the next iteration of the loop.
Here's an example:
for i := 1; i <= 5; i++ {
if i == 3 {
continue
}
fmt.Println(i)
}
In this code, when the i == 3
condition is true, the continue
statement is executed, and the rest of the loop body is skipped. The output of this code will be:
1
2
4
5
Conclusion
That's a wrap on the for
loop in Go! We covered the basic syntax of the for
loop, how to create infinite loops, the range
keyword for iterating over elements, nested loops, and the break
and continue
statements for controlling loop flow.
The for
loop is a powerful tool that allows you to repeat a block of code multiple times. It's an essential construct to master as a Go programmer.
I hope you found this article helpful. Stay tuned for more articles in our Go by Example series!