Go by Example: Arrays
Arrays in Go are fixed-size collections of elements of the same type. They provide efficient storage and access, making them ideal for storing related items. Learn how to declare, access, and iterate over arrays in Go.
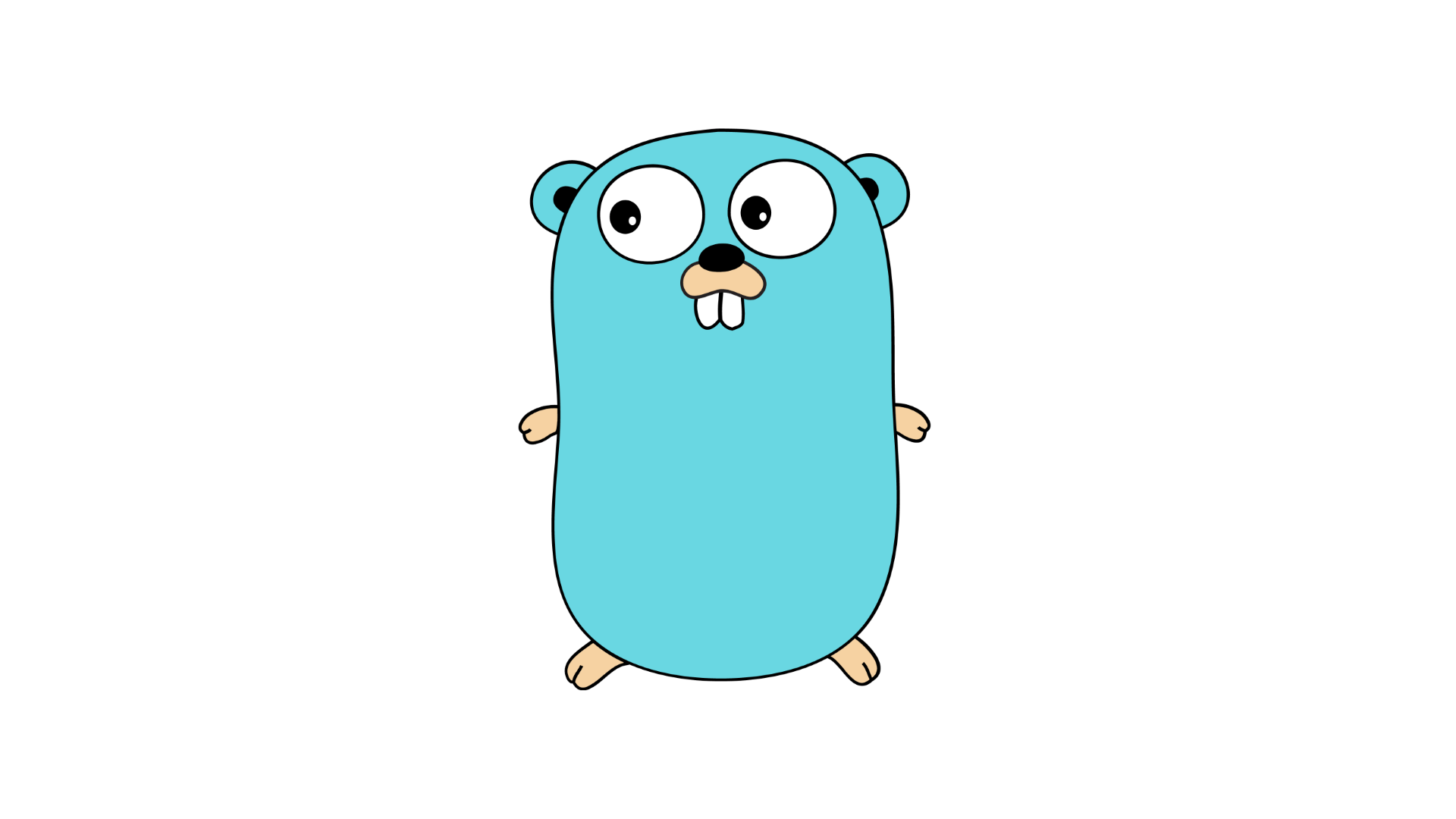
Introduction
Welcome to another installment of our Go by Example series! In this edition, we'll be diving into the topic of arrays in Go. Arrays are fundamental data structures that allow you to store and manipulate collections of elements of the same type. Whether you're a beginner or an experienced Go programmer, understanding arrays is essential for building robust and efficient applications. So, let's get started and explore arrays in Go!
What Are Arrays?
An array is a fixed-size collection of elements of the same type. Each element in an array is identified by its index, starting from zero. This means that if you have an array with a length of n
, the valid indices for accessing its elements range from 0
to n-1
. Arrays are useful when you need to store a fixed number of items that are related and need to be accessed individually.
Declaring Arrays
In Go, you declare an array by specifying the type of its elements and its length. The syntax to declare an array is as follows:
```go var arrayName [length]elementType ```
For example, let's say we want to declare an array of 5 integers:
```go var numbers [5]int ```
This code declares an array named numbers
that can hold 5 integers. By default, all the elements in the array are set to their respective zero
values.
Accessing Array Elements
To access individual elements of an array, you use the array's name followed by the index in square brackets. Here's an example:
```go numbers[0] = 10 fmt.Println(numbers[0]) ```
In this code snippet, we assign the value 10
to the first element of the numbers
array, which is at index 0
. We then print the value of that element using fmt.Println
, which outputs 10
to the console.
Array Literals
Go provides a convenient syntax for initializing arrays called array literals. With array literals, you can declare and initialize an array in a single line. Here's an example:
```go fruits := [3]string{"apple", "banana", "orange"} ```
In this code, we declare an array named fruits
with a length of 3
and initialize it with three string literals: "apple"
, "banana"
, and "orange"
. Note that the length of the array is determined by the number of elements in the literal.
Array Length
The length of an array is a fixed value that is determined at compile-time. You can obtain the length of an array using the built-in len
function. Here's an example:
```go fruits := [3]string{"apple", "banana", "orange"} fmt.Println(len(fruits)) ```
In this code, we declare an array named fruits
with a length of 3
. We then use the len
function to obtain the length of the fruits
array, which outputs 3
to the console.
Iterating Over Arrays
Go provides a convenient way to iterate over the elements of an array using the range
keyword. The range
keyword returns both the index and the value of each element. Here's an example:
```go fruits := [3]string{"apple", "banana", "orange"} for index, value := range fruits { fmt.Println(index, value) } ```
This code iterates over the fruits
array and prints the index and value of each element to the console. The output will be:
``` 0 apple 1 banana 2 orange ```
Multidimensional Arrays
In Go, you can create multidimensional arrays by nesting arrays within arrays. Here's an example of a 2-dimensional array:
```go var matrix [3][3]int ```
This code declares a 2-dimensional array called matrix
with a size of 3x3
. Each element in the array is of type int
. You can access and modify individual elements of the matrix
array using multiple indices. For example:
```go matrix[0][0] = 1 ```
This code assigns the value 1
to the first element in the matrix
array.
When to Use Arrays
Arrays are useful when you have a fixed number of items that need to be accessed individually. For example, you might use an array to store a list of student grades, a collection of coordinates in a grid, or a set of configuration values. Arrays are also useful when you need predictable and constant-time access to elements.
Limitations of Arrays
Arrays in Go have a fixed size that is determined at compile-time. This means that once you declare an array with a specific length, you cannot change its size. If you need to dynamically resize your collection, consider using slices instead, which are more flexible.
Conclusion
Congratulations! You've learned the basics of arrays in Go. You now have the knowledge to declare arrays, access their elements, initialize them using literals, iterate over them, and create multidimensional arrays. Arrays are an essential building block in any programming language, and Go provides a powerful and efficient way to work with them.
Thanks for joining us on this journey through arrays in Go. Stay tuned for the next installment in our Go by Example series!