Getting Started with gRPC: A Comprehensive Guide
"Discover the power of gRPC, an efficient communication framework for building microservices. Learn how to define services using Protocol Buffers, generate code, and implement a gRPC server. Start building your next-gen microservices with gRPC today!"
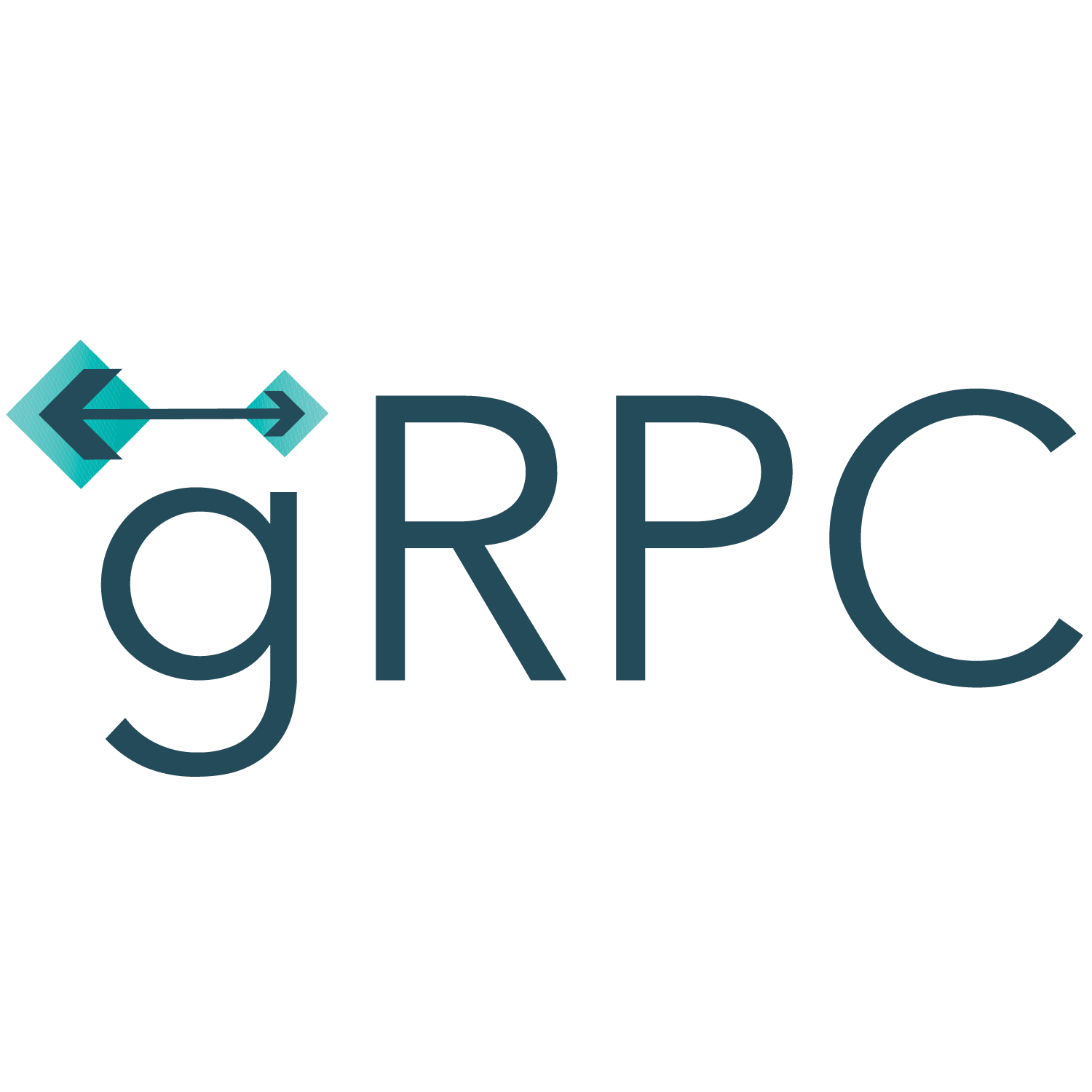
Introduction
Welcome to Getting Started with gRPC: A Comprehensive Guide. In this guide, we will explore the fundamentals of gRPC and provide you with the knowledge needed to start building efficient and scalable microservices using this modern and powerful communication framework.
What is gRPC?
gRPC is an open-source remote procedure call (RPC) framework that was developed by Google. It allows you to build distributed systems by defining services and message types using the Protocol Buffers language, and then generating efficient client and server code in various programming languages.
The core idea behind gRPC is to enable different services to communicate with each other easily and efficiently, regardless of the programming languages they are implemented in. It achieves this by allowing you to define the structure of the messages and the remote methods using a language-agnostic Interface Definition Language (IDL).
The Benefits of gRPC
gRPC offers a number of advantages over traditional HTTP-based APIs:
Efficiency
Unlike REST-based APIs, which often rely on JSON or XML for serialization, gRPC uses Protocol Buffers (protobufs) to define the messages and methods. These protobufs are binary-encoded, which makes them more compact and efficient for both data transfer and parsing.
Additionally, gRPC takes advantage of HTTP/2, a modern and efficient communication protocol, which allows for multiplexing and request/response streaming. This enables gRPC to support high-performance and low-latency communication between services, making it a great choice for building microservices architectures.
Strong Typing
With gRPC, the client and server code is generated based on the protobuf definitions. This means that the communication is strongly typed, with each message and method having a well-defined structure. This makes it easier to identify and fix errors during development, and provides better tooling support for refactoring and code generation.
Polyglot Support
One of the key benefits of gRPC is its polyglot support. gRPC can generate client and server code in multiple programming languages, including Java, C++, Go, Python, and many more. This means that you can use gRPC to build distributed systems that are not tied to a specific programming language or technology stack.
Setting Up Your Development Environment
Before we dive into building gRPC services, let's take a moment to set up our development environment. Here are the basic prerequisites:
- Install Protocol Buffers Compiler (protoc)
- Install the gRPC code generator plugin for protoc
If you're using a specific programming language, you may need to install language-specific gRPC tools and libraries. These can be easily found in the official gRPC documentation for your chosen language.
Defining a Service with Protobuf
The first step in building a gRPC service is to define the service and the message types using Protocol Buffers. Protocol Buffers is a language-agnostic binary serialization format that gRPC uses to define the structure of the messages and services.
Let's look at an example of a simple "Hello World" service:
syntax = "proto3";
package helloworld;
service Greeter {
rpc SayHello (HelloRequest) returns (HelloResponse);
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
In this example, we define a service called `Greeter` with a single remote method called `SayHello`. The `SayHello` method takes a message of type `HelloRequest` as input and returns a message of type `HelloResponse` as output.
Each message type is defined using the `message` keyword, followed by the name of the message and its fields. In our example, the `HelloRequest` message has a single field called `name` with type `string`.
Once we have defined the service and messages using Protocol Buffers, we can use the `protoc` compiler to generate the client and server code in our desired programming language. This will allow us to implement the logic for our service and interact with it programmatically.
Implementing a gRPC Service
Now that we have defined our service using Protocol Buffers, we can start implementing it in our chosen programming language.
Each language-specific gRPC library provides a set of tools and APIs that make it easy to build gRPC services and clients. These libraries use the generated code from the `protoc` compiler to provide a high-level interface for working with gRPC.
Let's take a look at an example of how to implement a gRPC service in Go:
package main
import (
"context"
"log"
"net"
"google.golang.org/grpc"
)
type greeterServer struct{}
func (s *greeterServer) SayHello(ctx context.Context, req *pb.HelloRequest) (*pb.HelloResponse, error) {
name := req.GetName()
message := "Hello, " + name
return &pb.HelloResponse{Message: message}, nil
}
func main() {
lis, err := net.Listen("tcp", ":50051")
if err != nil {
log.Fatalf("failed to listen: %v", err)
}
s := grpc.NewServer()
pb.RegisterGreeterServer(s, &greeterServer{})
log.Printf("server listening at %v", lis.Addr())
if err := s.Serve(lis); err != nil {
log.Fatalf("failed to serve: %v", err)
}
}
Here, we define a struct called `greeterServer` that implements the methods defined in the `Greeter` service. In our case, we only have one method: `SayHello`. This method takes a `HelloRequest` message as input and returns a `HelloResponse` message as output.
We then create a gRPC server using `grpc.NewServer()`, register our service with the server using `pb.RegisterGreeterServer()`, and start listening on a specific network address.
With our gRPC service implemented, we can now build and run it. Make sure you have the necessary dependencies installed and run the following command:
go run main.go
Once the service is running, you can start interacting with it using the generated gRPC client code, or by using external tools like BloomRPC or gRPCurl.
Conclusion
Congratulations! You have now been introduced to the basics of gRPC and have learned how to define a service using Protocol Buffers, generate the necessary code, and implement a gRPC server in your chosen programming language.
With its efficiency, strong typing, and polyglot support, gRPC is a powerful communication framework that can greatly simplify the development of distributed systems and microservices.
Now that you have a solid foundation, you can explore more advanced features of gRPC, such as client-side and server-side streaming, bi-directional streaming, and authentication and authorization.
So go ahead and start building your next generation of efficient and scalable microservices with gRPC. Happy coding!