Golang for Game Development: Creating 2D and 3D Games
Learn how to create 2D and 3D games with Golang! Explore libraries like Ebiten and Azul3D, and start building your dream game today. Happy coding!
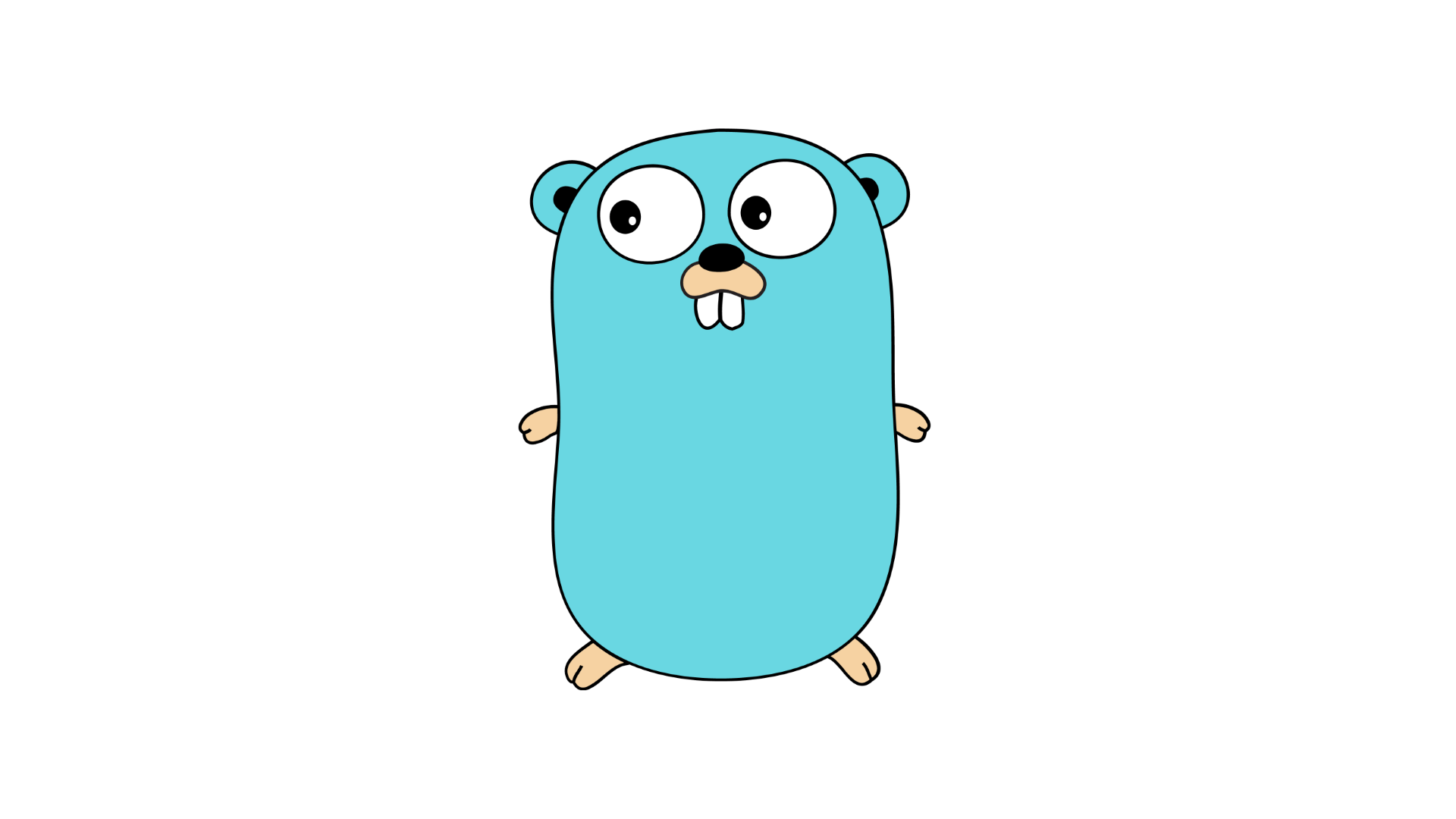
Introduction
Are you a game development enthusiast looking to explore new programming languages? Look no further! In this blog post, we will dive into the world of game development with Golang. Often referred to as Go, Golang is a powerful and efficient programming language known for its simplicity and ease of use.
In this tutorial, we will focus on creating 2D and 3D games using Golang. We will explore some of the popular libraries and tools available in the Golang ecosystem that will help us bring our game ideas to life. So, let's get started!
Getting Started with Golang Game Development
Before we jump into game development, let's make sure we have all the necessary tools set up on our machine. Here's a step-by-step guide to get started with Golang game development:
Step 1: Install Golang
The first step is to install Golang on your machine. Visit the official Golang website (https://golang.org) and follow the installation instructions for your operating system. Once the installation is complete, open your terminal or command prompt to verify that Golang is installed correctly by running the following command:
go version
If the command returns the version of Go installed on your machine, you are good to go!
Step 2: Install Additional Tools and Libraries
Golang has a rich ecosystem of tools and libraries that make game development easier. Here are a few popular ones:
1. Ebiten
Ebiten is a 2D game library for Golang that provides a simple and intuitive API for creating games. It is built on top of OpenGL and provides features like input handling, audio playback, and sprite rendering. To install Ebiten, run the following command:
go get github.com/hajimehoshi/ebiten/v2
2. Engo Engine
Engo Engine is a 2D game engine for Golang that enables developers to create complex games with ease. It provides a powerful entity-component system and supports features like physics simulation, audio, and animation. To install Engo Engine, run the following command:
go get github.com/EngoEngine/engo/v2
3. Azul3D
Azul3D is a 3D game engine for Golang that offers a range of features for creating immersive 3D games. It provides a clean API for rendering 3D graphics, handling input, and implementing physics simulations. To install Azul3D, run the following command:
go get azul3d.org/engine
Feel free to explore other tools and libraries available in the Golang ecosystem based on your game development requirements.
Creating a 2D Game
Now that we have our development environment set up, let's dive into creating our first 2D game using Golang and Ebiten.
Step 1: Set Up the Project
Create a new directory for your project and navigate to it using your terminal or command prompt. Once inside the project directory, run the following command to initialize a new Go module:
go mod init your-module-name
This command will create a new go.mod
file, which will keep track of your project's dependencies.
Step 2: Import the Ebiten Library
In your project directory, create a new file called main.go
. Open the file in your favorite text editor and import the Ebiten library by adding the following line at the top:
import (
"github.com/hajimehoshi/ebiten/v2"
)
Step 3: Create the Game Structure
In the main.go
file, define a new structure to represent our game. This structure should implement the ebiten.Game
interface, which requires implementing three methods: Update()
to update the game state, Draw()
to draw the game, and Layout()
to define the screen size. Here's an example:
type Game struct {}
func (g *Game) Update() error {
// Update game logic here
return nil
}
func (g *Game) Draw(screen *ebiten.Image) {
// Draw game graphics here
}
func (g *Game) Layout(outsideWidth, outsideHeight int) (int, int) {
// Define screen size here
return screenWidth, screenHeight
}
Step 4: Initialize and Run the Game
In the main()
function, initialize a new instance of our game structure and pass it to the ebiten.Run()
function to start the game loop. Here's an example:
func main() {
game := &Game{}
ebiten.Run(game.Update, game.ScreenWidth, game.ScreenHeight, 1, "My 2D Game")
}
Replace game.ScreenWidth
and game.ScreenHeight
with the actual values for your game window size.
Step 5: Build and Run the Game
To build and run your 2D game, navigate to your project directory in the terminal or command prompt and run the following command:
go run main.go
If everything is set up correctly, you should see your game window open and your game loop running!
Creating a 3D Game
Now that we've explored 2D game development, let's take it a step further and learn how to create a 3D game using Golang and Azul3D.
Step 1: Set Up the Project
Similar to the 2D game, create a new directory for your project and navigate to it using your terminal or command prompt. Run the following command to initialize a new Go module:
go mod init your-module-name
Step 2: Import the Azul3D Library
In your project directory, create a new file called main.go
. Open the file in your favorite text editor and import the Azul3D library by adding the following line at the top:
import (
"azul3d.org/engine/audio"
"azul3d.org/engine/gfx"
"azul3d.org/engine/keyboard"
"azul3d.org/engine/lmath"
"azul3d.org/engine/window"
)
Step 3: Create the Game Structure
In the main.go
file, define a new structure to represent our game. This structure should implement the required methods for the azul3d.org/engine/engine.Engine
interface. Here's an example:
type Game struct {}
func (g *Game) Init(w window.Window) {}
func (g *Game) Render(r gfx.Renderer, deltaTime float64) {
// Render 3D graphics here
}
func (g *Game) Update(w window.Window, deltaTime float64) {
// Update game logic here
}
func (g *Game) Close() {}
func (g *Game) Run() {
// Run game loop here
}
Step 4: Initialize and Run the Game
In the main()
function, initialize a new instance of our game structure and call the Run()
method to start the game loop. Here's an example:
func main() {
game := &Game{}
window.Run(game.Run, &window.Config{
Title: "My 3D Game",
Width: 800,
Height: 600,
})
}
Replace Width
and Height
with the desired dimensions for your game window.
Step 5: Build and Run the Game
To build and run your 3D game, navigate to your project directory in the terminal or command prompt and run the following command:
go run main.go
If everything is set up correctly, you should see your 3D game window open and your game loop running!
Conclusion
Congratulations! You've learned the basics of game development with Golang. By exploring popular libraries like Ebiten for 2D game development and Azul3D for 3D game development, you have the tools necessary to create amazing games in Golang. Now, let your creativity flow and start building your dream game!
Stay tuned for more advanced tutorials on Golang game development, including topics like physics simulation, AI, and multiplayer networking. Happy coding!