Getting Started with Apache Kafka: A Comprehensive Guide
Learn how to build scalable, fault-tolerant, and real-time data streaming applications with Apache Kafka. This comprehensive guide covers key concepts, installation, integration, and more. Get started on your Kafka journey today!
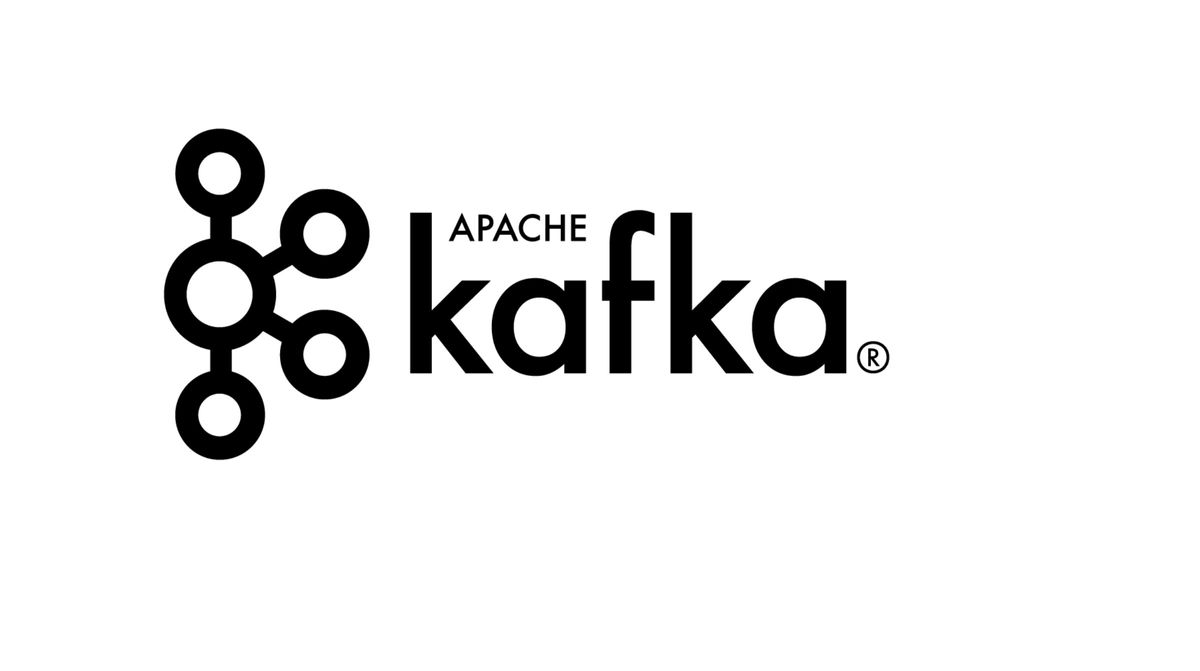
Getting Started with Apache Kafka: A Comprehensive Guide
If you're looking to build scalable, fault-tolerant, and real-time data streaming applications, Apache Kafka is the perfect tool for the job. Designed as a distributed streaming platform, Kafka enables you to publish and subscribe to streams of records in a fault-tolerant and durable manner. Whether you're processing large amounts of data, building event-driven microservices, or implementing real-time analytics, Kafka has you covered. In this comprehensive guide, we'll dive into the world of Apache Kafka and get you started on your journey to becoming a Kafka pro.
What is Apache Kafka?
Apache Kafka is an open-source distributed streaming platform that allows you to build real-time streaming data pipelines and applications. It was originally developed by LinkedIn and later donated to the Apache Software Foundation, where it became an Apache Top-Level Project. Kafka was designed to handle high-throughput, fault-tolerant, and scalable streaming data, making it a popular choice for building modern data infrastructure.
Kafka is built around the concept of a distributed commit log, which provides durability and fault tolerance by replicating data across multiple brokers or servers. Each broker in a Kafka cluster is responsible for storing and serving a portion of the data, allowing you to scale horizontally as your data and workload grow.
Key Concepts
Before we jump into the installation and usage of Kafka, let's familiarize ourselves with a few key concepts:
1. Topics
A topic is a category or feed name to which messages are published by producers. In simple terms, a topic represents a stream of records in Kafka. Think of it as a virtual bulletin board where producers can publish messages, and consumers can subscribe to those messages to process them.
2. Partitions
A partition is a log-structured unit of a topic that holds a sequence of records. Each topic is divided into one or more partitions, allowing you to parallelize the data ingestion and consumption. Partitions enable Kafka to achieve high throughput and scalability by distributing the load across multiple brokers.
3. Offsets
Every record within a partition is assigned a unique identifier called an offset. The offset represents the position of a record within a partition and can be used for both reading and writing data. Offsets are used for maintaining the order and guaranteeing the delivery of messages in Kafka.
4. Producer
A producer is a component that publishes messages to Kafka topics. Producers are typically responsible for generating data streams and feeding them into Kafka. They can be as simple as a standalone application or as complex as a distributed system generating huge volumes of data.
5. Consumer
A consumer is a component that subscribes to one or more topics and processes the published messages. Consumers read data from Kafka and process it based on the business logic defined in the consumer application. Consumers can be single-threaded or multi-threaded, and they can be part of a distributed system consuming data in parallel.
6. Consumer Groups
A consumer group is a group of related consumers that work together to consume and process messages from one or more topics. Within a consumer group, each partition is consumed by only one consumer, allowing you to horizontally scale by adding more consumers to a group. Consumer groups enable fault tolerance and high throughput by distributing the message processing across multiple consumers.
Installing and Configuring Apache Kafka
Now that we understand the key concepts of Kafka, let's move on to installing and configuring Kafka on your system. Follow the steps below to get started:
1. Download Apache Kafka
First, download the latest binary distribution of Apache Kafka from the official website (https://kafka.apache.org/downloads). Kafka is written in Scala, so make sure you have Java installed on your system.
2. Extract the Download Archive
After the download is complete, extract the contents of the archive to a directory of your choice. This directory will be referred to as the Kafka home directory in the subsequent steps.
3. Start the Kafka Server
To start the Kafka server, you need to run the following command from the Kafka home directory:
bin/kafka-server-start.sh config/server.properties
This command will start the Kafka server, and you should see the logs indicating the successful startup of the server.
4. Create a Topic
Now that the Kafka server is up and running, you can create a topic using the following command:
bin/kafka-topics.sh --create --topic my-topic --bootstrap-server localhost:9092 --replication-factor 1 --partitions 1
This command will create a topic named "my-topic" with a single partition and a replication factor of 1. Feel free to modify the topic name, partition count, and replication factor according to your requirements.
5. Send and Receive Messages
Now it's time to have some fun with Kafka! Let's send and receive messages with the Kafka command-line tools. To send messages to the "my-topic" topic, use the following command:
bin/kafka-console-producer.sh --topic my-topic --bootstrap-server localhost:9092
This command will open a console where you can start typing your messages. Every line you enter will be treated as a separate message and will be sent to the "my-topic" topic.
To receive messages from the "my-topic" topic, open a new terminal window or tab and run the following command:
bin/kafka-console-consumer.sh --topic my-topic --from-beginning --bootstrap-server localhost:9092
This command will start consuming messages from the beginning of the topic and display them on the console.
Integrating Kafka into Your Application
Now that you have Kafka up and running, it's time to integrate it into your application. Kafka provides language-specific client libraries for Java, Python, Go, and more. You can choose the client library that best suits your programming language and ecosystem.
Here's a simple example of how to use the Kafka Java client library to send and receive messages:
<dependency>
<groupId>org.apache.kafka</groupId>
<artifactId>kafka-clients</artifactId>
<version>2.8.0</version>
</dependency>
Properties properties = new Properties();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
properties.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(properties);
producer.send(new ProducerRecord<>("my-topic", "key", "value"));
producer.close();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
properties.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
Consumer<String, String> consumer = new KafkaConsumer<>(properties);
consumer.subscribe(Collections.singletonList("my-topic"));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
System.out.println("Received message: " + record.value());
}
}
Make sure to update the "bootstrap.servers" property with the correct Kafka broker addresses and modify the topic name accordingly.
Scaling and Fault Tolerance
One of the key advantages of Kafka is its ability to scale horizontally and provide fault tolerance. To scale Kafka, you can add more brokers to the cluster, distribute the partitions across multiple brokers, and increase the replication factor of the topics. Kafka takes care of the partition assignment and leader election process automatically.
Similarly, if a broker fails, Kafka ensures that the data and partition replicas are still available by electing new leaders and maintaining the desired replication factor. This fault-tolerance mechanism ensures the uninterrupted availability of your data and stream processing.
Wrapping Up
Congratulations on completing this comprehensive guide to getting started with Apache Kafka! You now have a solid foundation for building real-time streaming applications and processing large-scale data streams. Make sure to explore the Kafka documentation and experiment with the various features and configurations available.
Remember, Kafka is a powerful streaming platform with numerous use cases, from building event-driven architectures to implementing real-time analytics. Embrace the possibilities Kafka offers, and happy streaming!