Angular Testing: Ensuring Code Quality and Reliability
Testing is critical in Angular development. It catches bugs early, maintains code reliability, and ensures refactoring safety. Learn how to get started with Angular testing and follow best practices for robust applications.
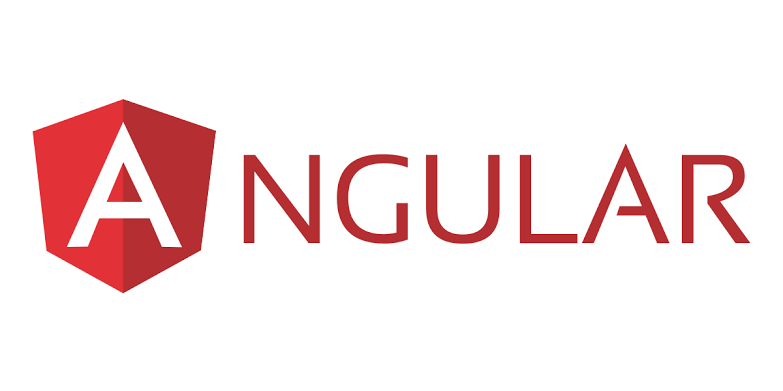
Introduction
Testing is a critical aspect of software development that ensures code quality and reliability. In the world of web development, Angular testing plays a crucial role in maintaining the stability and performance of Angular applications. By thoroughly testing your Angular code, you can catch bugs early, identify potential issues, and deliver a robust and highly functional application to your users.
Why Angular Testing?
Angular testing allows you to verify that your code works as expected and meets the requirements defined in your application. Here are some key reasons why Angular testing is essential:
- Early Bug Detection: Writing tests while developing your Angular application helps identify bugs and issues before they become challenging to fix.
- Code Reliability: Well-tested code ensures that all components, services, and directives work together correctly without unexpected side effects.
- Refactoring Safety: Tests provide confidence when refactoring your codebase, as you can quickly verify that existing functionality is still working as intended.
- Continuous Integration: Automated tests facilitate continuous integration pipelines, allowing you to deploy code with confidence.
- Improved Code Documentation: Tests serve as a living documentation for your code, describing the expected behavior and usage of your components.
Types of Angular Tests
Angular provides a wide variety of testing options to suit different scenarios and requirements. Let's explore some of the most common types of Angular tests:
1. Unit Tests
Unit tests focus on testing individual units of code in isolation. In Angular, unit tests typically validate the behavior of individual components, services, or directives. By mocking dependencies and isolating units of code, you can thoroughly test the logic and functionality of your components without relying on external resources.
2. Integration Tests
Integration tests verify the interaction and integration between different components and services within your Angular application. These tests ensure that different parts of your application work correctly together and that data flows as expected. Integration tests are valuable for catching issues that may arise from the integration of different modules and services.
3. End-to-End Tests
End-to-end (E2E) tests simulate real user scenarios by testing the complete flow of your Angular application. These tests allow you to validate the application's behavior from the user's perspective, including interactions with the user interface and backend services. Angular provides tools like Protractor for writing E2E tests that interact with the application in a browser-like environment.
Testing Frameworks for Angular
Angular supports multiple testing frameworks to help you write tests efficiently. The two main frameworks are:
1. Jasmine
Jasmine is a popular behavior-driven development (BDD) testing framework that provides an easy-to-understand syntax for writing tests. It offers a comprehensive set of built-in assertions, mocks, and spies that make it suitable for writing unit, integration, and even E2E tests in Angular applications.
2. Karma
Karma is a powerful test runner for running tests written with Jasmine, Mocha, or other testing frameworks supported by Angular. It provides an environment to execute tests on multiple browsers and devices simultaneously, making it easier to catch cross-browser compatibility issues and ensure consistent behavior across different environments.
Getting Started with Angular Testing
Now that we understand the importance of Angular testing and the different types of tests, let's dive into how to get started with Angular testing:
1. Setting Up a Testing Environment
To begin writing tests for your Angular application, you need to set up a testing environment. Angular provides a testing package called @angular/core/testing
that includes utilities for configuring the testing environment, such as mocking dependencies and creating test components. You can install it using npm or yarn:
npm install @angular/core@latest @angular/compiler@latest --save-dev
2. Creating Component Tests
Component tests are a fundamental part of Angular testing. You can create a component test using the Angular Testing APIs. These APIs allow you to create a test bed, configure dependencies, and simulate user interactions. Here's an example of a simple component test:
import { ComponentFixture, TestBed } from '@angular/core/testing';
import { MyComponent } from './my.component';
describe('MyComponent', () => {
let component: MyComponent;
let fixture: ComponentFixture<MyComponent>;
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [MyComponent]
}).compileComponents();
});
beforeEach(() => {
fixture = TestBed.createComponent(MyComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
it('should render something', () => {
const compiled = fixture.nativeElement;
expect(compiled.querySelector('h1').textContent).toContain('Hello, World!');
});
});
3. Running Tests
Angular provides the ng test
command to run your tests using Karma. This command launches the test runner and executes all the test files in your Angular project. By default, Karma watches for changes to your test files and re-runs the tests on file save:
ng test
Best Practices for Angular Testing
Effective Angular testing involves following best practices to ensure reliable and maintainable tests. Here are some best practices to consider:
- Write focused tests: Each test should focus on a specific behavior or feature.
- Use test doubles: Mock or stub external dependencies to isolate units of code.
- Test with realistic data: Write tests with realistic data to simulate real-world scenarios.
- Test edge cases: Include tests that cover edge cases to ensure robustness.
- Keep tests independent: Avoid test dependencies to maintain test isolation and prevent cascading test failures.
- Refactor tests when refactoring code: As you refactor your code, make sure to update and refactor your tests accordingly.
Conclusion
Angular testing is a crucial aspect of building high-quality applications. By writing and running tests regularly, you can ensure that your Angular application functions as expected, catches bugs early, and delivers a reliable user experience. Get started with Angular testing today, and strengthen the quality and reliability of your Angular applications!
Thank you for reading! Stay tuned for more articles on Angular development, testing, and best practices.