Implementing Redis in Python: A Step-by-Step Tutorial
Learn how to implement Redis in Python. This step-by-step tutorial covers connecting to Redis, setting/getting values, working with lists/sets/hashes, and using extra features. Boost your applications with Redis!
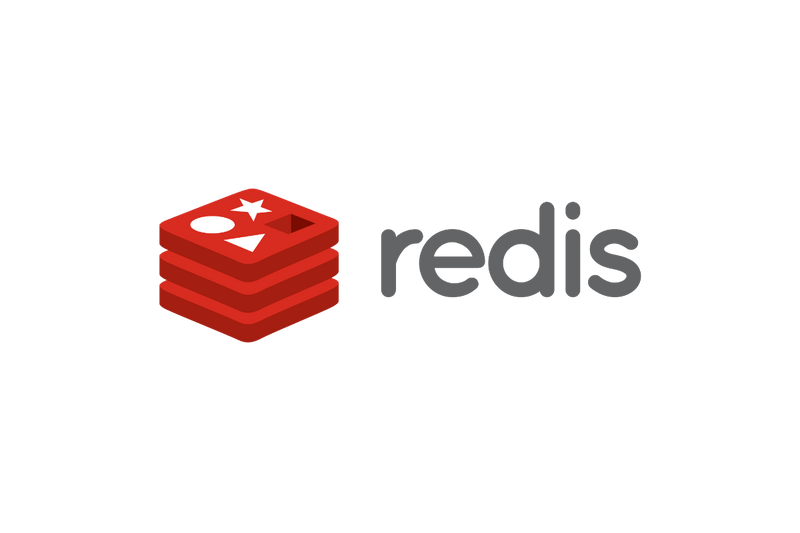
Introduction
Welcome to our step-by-step tutorial on implementing Redis in Python! Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It's known for its speed, scalability, and versatility, making it a popular choice among developers.
In this tutorial, we will walk you through the process of setting up Redis, installing the Redis package for Python, and implementing Redis features in your Python applications. So, let's get started!
Prerequisites
Before we begin, make sure you have the following prerequisites:
- Python installed on your system
- PIP package manager
- Redis server installed and running
If you haven't installed Redis yet, you can download it from the official Redis website and follow the installation instructions for your operating system.
Installing the Redis Package
The first step is to install the Redis package for Python. Open your terminal or command prompt and run the following command:
pip install redis
This command will download and install the Redis package from the Python Package Index (PyPI).
Connecting to the Redis Server
Now that we have the Redis package installed, let's establish a connection to the Redis server from our Python program. Create a new Python script file and import the Redis module:
import redis
Next, create a new Redis client object and connect to the Redis server:
redis_client = redis.Redis(host='localhost', port=6379)
Make sure to replace localhost
with the hostname or IP address of your Redis server if it's running on a different machine. Also, update the port
number if you have configured Redis to use a different port.
Setting and Getting Values
With the Redis client connected to the server, you can start setting and getting values from Redis. Redis uses a key-value pair structure to store data.
Let's see how to set a value in Redis using the set
command:
redis_client.set('key', 'value')
In the above example, we set the value value
for the key key
.
To retrieve a value from Redis, we can use the get
command:
value = redis_client.get('key')
print(value)
The above code will print the value associated with the key key
in Redis. If the key doesn't exist, it will return None
.
Working with Multiple Values
Redis also allows you to work with multiple values using data structures such as lists, sets, and hashes.
Lists
To work with lists in Redis, you can use the lpush
and rpush
commands to add elements to the left and right ends of the list, respectively. The lrange
command allows you to retrieve a range of elements from a list. Here's an example:
# Add elements to the list
redis_client.lpush('mylist', 'element1', 'element2')
redis_client.rpush('mylist', 'element3')
# Retrieve elements from the list
elements = redis_client.lrange('mylist', 0, -1)
print(elements)
The above code adds three elements to the list mylist
and then retrieves all the elements using lrange
. The output will be a list of all the elements in the order they were inserted.
Sets
Sets in Redis are unordered collections of unique elements. You can add elements to a set using the sadd
command, remove elements using the srem
command, and retrieve all elements in a set using the smembers
command. Here's an example:
# Add elements to the set
redis_client.sadd('myset', 'element1', 'element2', 'element3')
# Remove an element from the set
redis_client.srem('myset', 'element2')
# Retrieve all elements from the set
elements = redis_client.smembers('myset')
print(elements)
In the above code, we add three elements to the set myset
, remove element2
from the set, and retrieve all the elements using smembers
.
Hashes
Hashes in Redis are maps between string fields and string values. You can add fields and values to a hash using the hset
command, retrieve values using the hget
command, and retrieve all fields and values using the hgetall
command. Here's an example:
# Add fields and values to the hash
redis_client.hset('myhash', 'field1', 'value1')
redis_client.hset('myhash', 'field2', 'value2')
# Retrieve a value from the hash
value = redis_client.hget('myhash', 'field1')
print(value)
# Retrieve all fields and values from the hash
all_values = redis_client.hgetall('myhash')
print(all_values)
The above code adds two fields with their corresponding values to the hash myhash
, retrieves the value of field1
, and retrieves all fields and values from the hash using hgetall
.
Extra Features
Expiring Keys
Redis allows you to set an expiration time for keys using the expire
command. This is useful when you want certain keys to expire after a specific period of time. Here's an example:
redis_client.set('key', 'value')
redis_client.expire('key', 60) # Expire after 60 seconds
In the above code, we set the key key with the value value and set the expiration time to 60 seconds. After 60 seconds, the key will automatically be deleted from Redis.
Publish and Subscribe
Redis supports a publish-subscribe messaging pattern. You can publish messages to channels and subscribe to channels to receive those messages. Here's an example:
For the publisher:
redis_client.publish('channel', 'message')
For the subscriber:
pubsub = redis_client.pubsub()
pubsub.subscribe('channel')
for message in pubsub.listen():
print(message)
In the above code, the publisher publishes a message to the channel channel. The subscriber subscribes to the same channel and listens for messages. When a message is received, it will be printed.
Conclusion
Congratulations! You have successfully implemented Redis in Python. We have covered the basics of connecting to the Redis server, setting and getting values, working with multiple values, and using some extra features like expiring keys and publish-subscribe messaging.
Redis has a lot more to offer, including advanced data structures, transactions, and Lua scripting. I encourage you to explore the Redis documentation to learn more about its features and capabilities.
I hope you found this tutorial helpful. Happy coding!