Go by Example: Structs
Structs in Go are a powerful way to organize and manage data. Learn how to define, initialize, access, and modify struct values with examples in this tutorial.
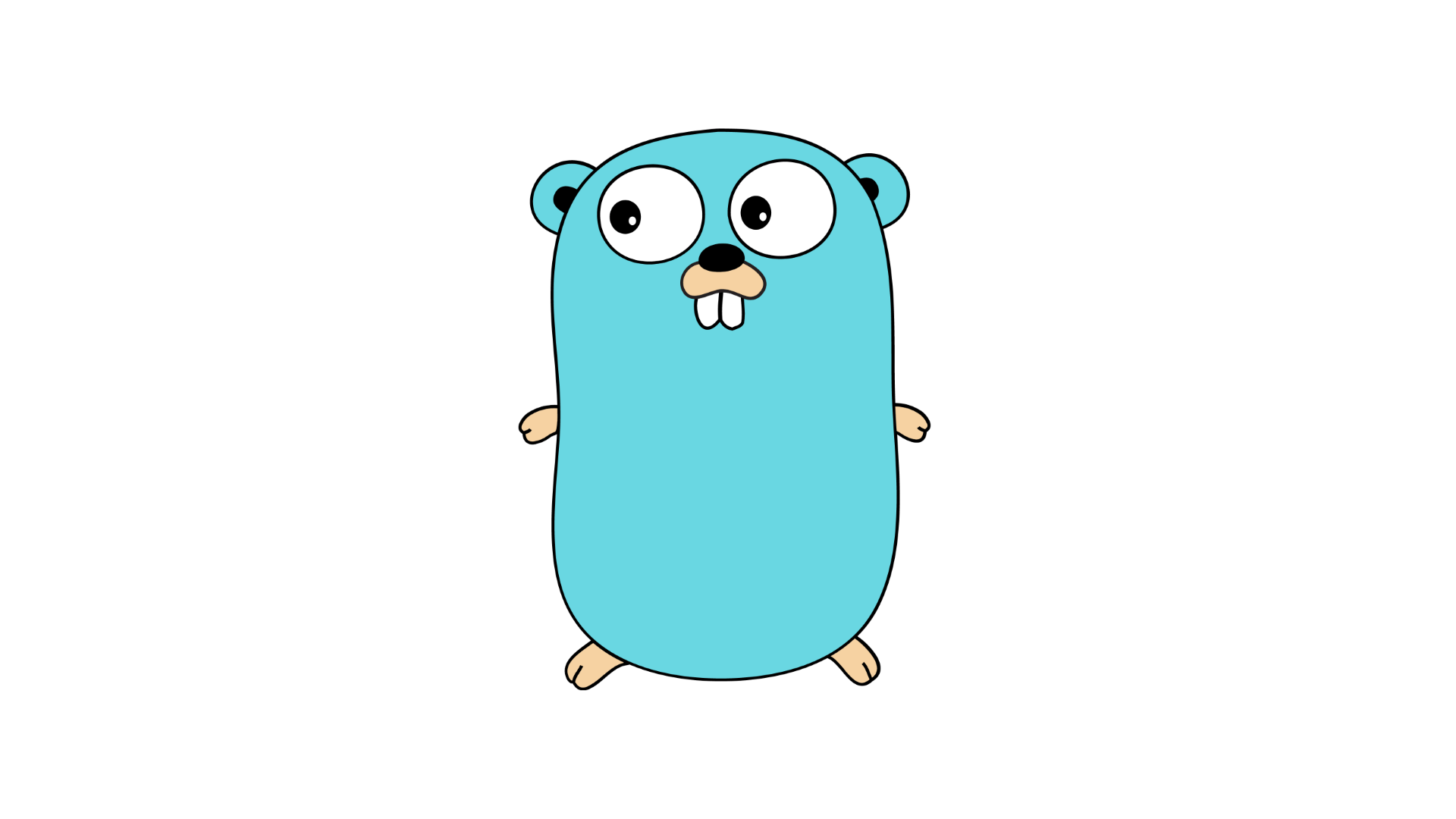
Introduction
Structs are an essential feature in Go programming language. They allow you to create custom data types that group together values of different types. This can be incredibly useful for organizing and managing data in your Go programs. In this tutorial, we will explore structs in Go by example, covering their syntax, initialization, and usage.
Defining Structs
To define a struct in Go, you use the type
keyword followed by the struct's name, and then a list of fields enclosed in curly braces.
type Person struct {
Name string
Age int
Email string
}
In the example above, we define a struct called Person
with three fields: Name
of type string
, Age
of type int
, and Email
of type string
.
Initializing Structs
There are a couple of ways to initialize a struct in Go. One way is to use the literal syntax, where you simply list the values in the order they appear in the struct definition:
person := Person{"John Doe", 30, "[email protected]"}
In the example above, we create a person
variable of type Person
and initialize it with the values "John Doe"
for Name
, 30
for Age
, and "[email protected]"
for Email
.
Another way to initialize a struct is by using named fields:
person := Person{
Name: "John Doe",
Age: 30,
Email: "[email protected]",
}
Named fields allow you to specify the values for individual fields, regardless of their order in the struct definition.
Accessing Values
To access the values of a struct, you use the dot notation followed by the field name. For example:
fmt.Println(person.Name) // Output: John Doe
fmt.Println(person.Age) // Output: 30
fmt.Println(person.Email)// Output: [email protected]
Modifying Values
Struct fields are mutable, meaning you can modify their values once a struct is initialized. Here's an example:
person.Age = 31
person.Email = "[email protected]"
fmt.Println(person.Age) // Output: 31
fmt.Println(person.Email)// Output: [email protected]
In the example above, we modify the values of the Age
and Email
fields using the dot notation and then print the updated values.
Structs as Parameters
You can pass structs as parameters to functions in Go. This allows you to encapsulate related values and perform operations on them. Here's an example:
func printPerson(person Person) {
fmt.Println("Name:", person.Name)
fmt.Println("Age:", person.Age)
fmt.Println("Email:", person.Email)
}
func main() {
person := Person{"John Doe", 30, "[email protected]"}
printPerson(person)
}
The printPerson
function takes a Person
struct as a parameter and prints its fields. In the main
function, we create a person
variable and pass it to the printPerson
function.
Structs as Return Values
Functions in Go can also return structs. This allows you to create functions that generate and return custom data types. Here's an example:
func createPerson(name string, age int, email string) Person {
return Person{
Name: name,
Age: age,
Email: email,
}
}
func main() {
person := createPerson("John Doe", 30, "[email protected]")
fmt.Println("Name:", person.Name)
fmt.Println("Age:", person.Age)
fmt.Println("Email:", person.Email)
}
In this example, the createPerson
function takes individual values for Name
, Age
, and Email
and returns a Person
struct. In the main
function, we call createPerson
and print its returned fields.
Embedding Structs
In Go, you can embed one struct into another, allowing you to create more complex data structures. This is achieved by including the embedded struct's type as a field in the outer struct.
type Contact struct {
Phone string
Address string
}
type Person struct {
Name string
Age int
Contact // embedded struct
}
func main() {
person := Person{
Name: "John Doe",
Age: 30,
Contact: Contact{
Phone: "123-456-7890",
Address: "123 Main St",
},
}
fmt.Println("Name:", person.Name)
fmt.Println("Age:", person.Age)
fmt.Println("Phone:", person.Contact.Phone)
fmt.Println("Address:", person.Contact.Address)
}
In this example, we define a Contact
struct with two fields: Phone
and Address
. We then create a Person
struct with two fields: Name
and Age
. The Contact
struct is embedded into the Person
struct, which means the Contact
fields are accessible directly from the Person
struct.
Wrapping Up
Congratulations! You've learned the basics of working with structs in Go. Structs are a fundamental part of Go programming and are essential for creating complex data structures. By using structs, you can organize and manage your data more effectively, improving the overall structure and readability of your code.
Remember to practice what you've learned by experimenting with different struct definitions and usage scenarios. This will help solidify your understanding and broaden your Go programming skills.
Stay tuned for more Go by Example tutorials where we'll continue exploring Go's powerful features!