Go by Example: Functions
Learn about functions in Go! Functions are the building blocks of any program, encapsulating code that can be reused. Understand declaration, calling, and usage of functions.
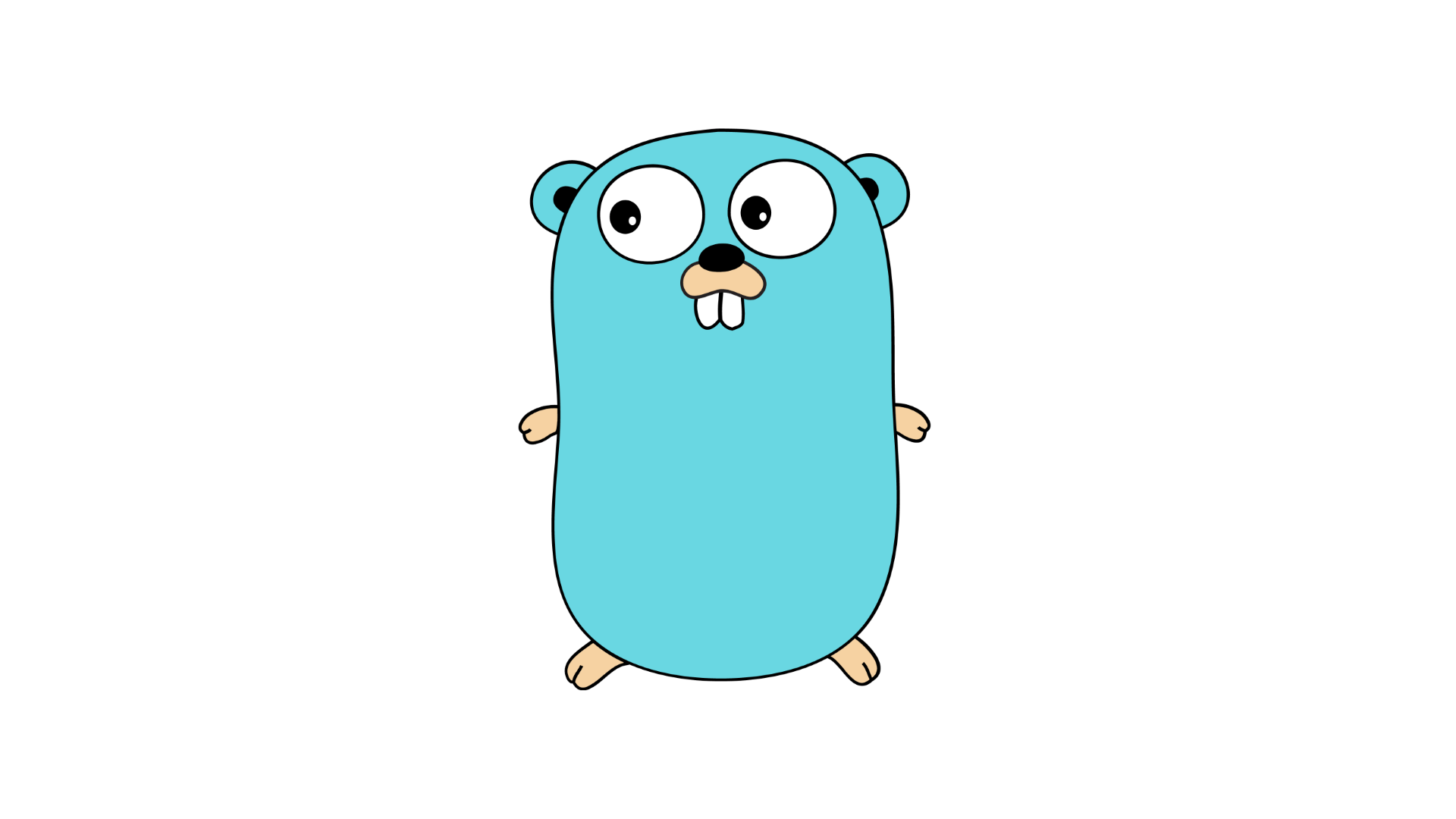
Introduction
Welcome to the world of Go programming! In this Go by Example tutorial, we will dive into the fascinating concept of functions in Go. Functions are the building blocks of any programming language, and Go is no exception. By mastering functions, you will gain the ability to write reusable code and create modular programs. So, let's get started and explore functions in Go!
What are Functions?
In Go, a function is a group of statements that perform a specific task. It takes input values, performs operations on them, and returns a result. Functions encapsulate a piece of code that can be reused throughout your program, making your code more organized and maintainable.
Let's dive deeper into the syntax and usage of functions in Go.
Function Declaration
In Go, a function is declared using the func
keyword followed by the function name and a list of parameters enclosed in parentheses. If the function returns a value, you need to specify the return type after the parameter list. Here's the general syntax:
func functionName(parameter1 type1, parameter2 type2) returnType {
// function body
// perform operations
return value
}
Let's break down the syntax:
func
: The keyword that marks the beginning of a function declaration.functionName
: The name you choose for your function. It should be descriptive and follow naming conventions.parameter1, parameter2, ...
: The arguments or input values that the function expects. Each parameter should have a type specified.returnType
: The type of value that the function returns. If the function doesn't return any value, you can specifyvoid
or simply omit the return type.function body
: The code enclosed in curly braces that defines the tasks to be performed by the function.return value
: If the function has a return type specified, you must use thereturn
keyword followed by the value to be returned.
Function Call
Once you have defined a function, you can call it from anywhere in your program by using its name and passing the required arguments. Here's the syntax:
functionName(argument1, argument2, ...)
Let's break down the syntax:
functionName
: The name of the function you want to call.argument1, argument2, ...
: The values you want to pass to the function. The number and types of arguments should match the defined parameters.
Example: Functions in Go
Now that you have a deep understanding of function declaration and function calls, let's look at some examples to solidify your knowledge. We'll start with a simple function that calculates the sum of two numbers:
func sum(a int, b int) int {
return a + b
}
func main() {
result := sum(5, 7)
fmt.Println("Sum:", result)
}
In the example above, we define a function called sum
that takes two int
parameters and returns an int
value. Inside the main
function, we call the sum
function with the values 5
and 7
. The returned sum is stored in the variable result
and printed to the console using the fmt.Println
function.
Let's look at another example. This time, we'll create a function that checks if a number is even or odd:
func isEven(number int) bool {
if number % 2 == 0 {
return true
}
return false
}
func main() {
result := isEven(7)
if result {
fmt.Println("Number is even")
} else {
fmt.Println("Number is odd")
}
}
In this example, we define a function called isEven
that takes an int
parameter and returns a bool
value. Inside the function, we use an if
statement to check if the number is divisible by 2 without a remainder. If it is, we return true
, indicating that the number is even. Otherwise, we return false
. In the main
function, we call the isEven
function with the value 7
and print the result to the console.
Conclusion
Congratulations! You've successfully learned about functions in Go. You now have the knowledge to declare and call functions, pass arguments, and return values. Functions are one of the fundamental building blocks of Go programming, and understanding how to use them effectively will greatly enhance your programming skills.
Stay tuned for the next part of our Go by Example tutorial series, where we'll explore more advanced concepts and techniques. Happy coding!