Go by Example: Maps
Maps in Go are a powerful data structure that allow you to associate values with keys. This tutorial covers how to define, initialize, manipulate, and iterate over maps in Go. Learn how to make the most of this versatile tool for key-based lookup operations.
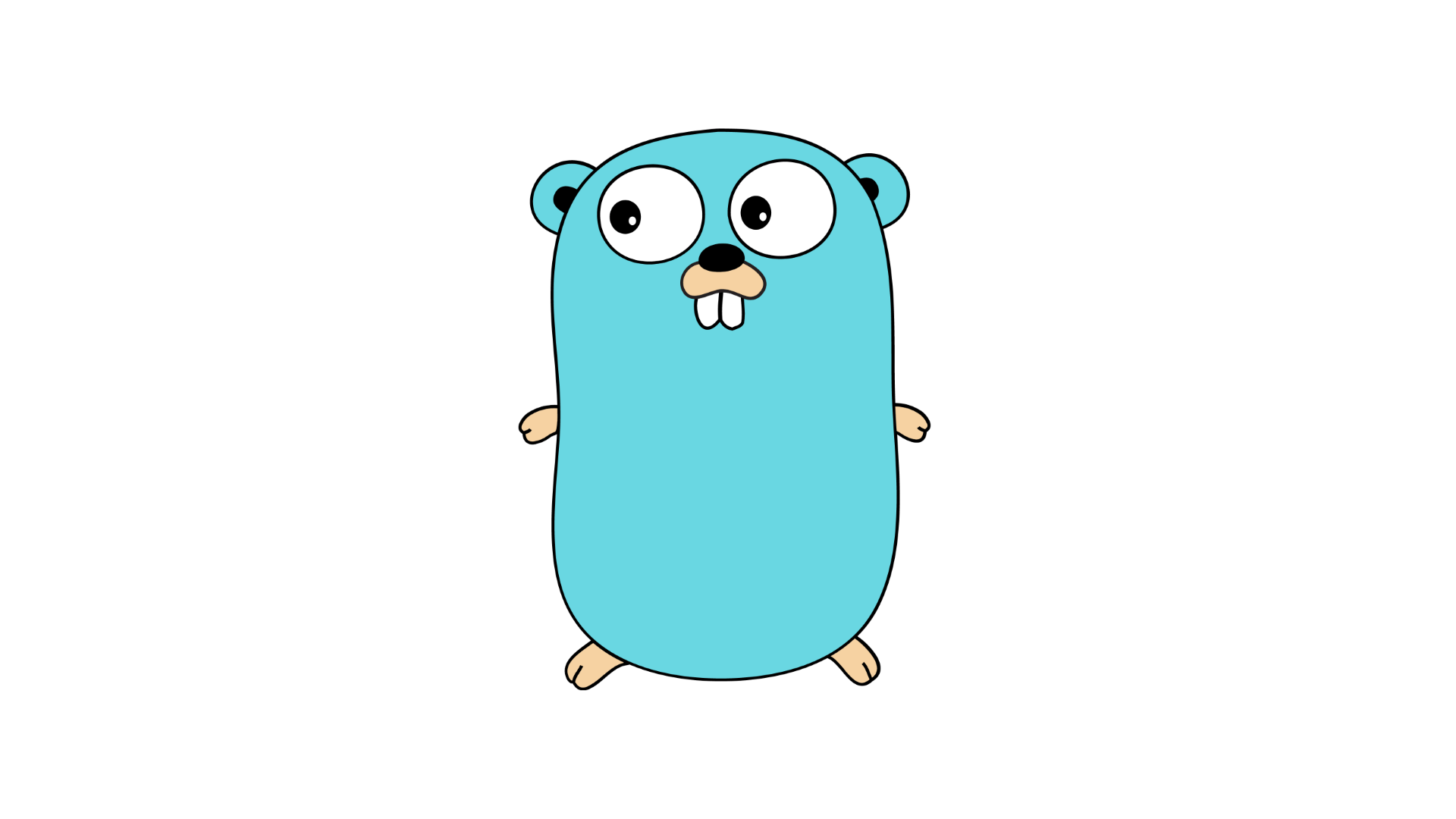
Introduction
Welcome to another installment of our Go by Example series! In this article, we'll be exploring the use of maps in Go. Maps are a powerful data structure that allow you to associate values with keys, making them a fundamental part of many programming tasks. By the end of this article, you'll have a solid understanding of how to define, manipulate, and iterate over maps in Go.
What are Maps?
A map in Go is an unordered collection of key-value pairs. The map provides a way to associate a value with a unique key, which can be of any comparable type, such as strings, numbers, or even custom data types. Maps are efficient for looking up values based on their keys, making them ideal for tasks that require quick access to stored data.
Defining a Map
In Go, you can define a map using the following syntax:
map[keyType]valueType
Here, keyType
is the data type of the key, and valueType
is the data type of the corresponding value. For example, to define a map that associates strings with integers, you would use the syntax map[string]int
. Let's take a look at an example:
var employeeSalary map[string]int
In the above example, we're declaring a map named employeeSalary
that associates strings (employee names) with integers (their respective salaries). Note that when a map is declared but not explicitly initialized, it is assigned the zero value of the map type, which is nil
. To initialize the map, we can use the make
function, as we'll see in the next section.
Initializing a Map
To initialize a map in Go, you can use the make
function. Here's the syntax:
make(map[keyType]valueType)
Let's see an example:
employeeSalary := make(map[string]int)
In the above example, we're using the make
function to initialize the employeeSalary
map. This creates an empty map, ready to store string keys and integer values. Alternatively, you can initialize a map using a map literal, which we'll discuss in a later section.
Adding Elements to a Map
To add elements to a map in Go, you can use the following syntax:
mapName[key] = value
Here, mapName
is the name of the map, key
is the key you want to associate with the value, and value
is the value you want to store. Let's consider the following example:
employeeSalary["John"] = 5000
employeeSalary["Jane"] = 6000
In the above example, we're adding two elements to the employeeSalary
map. The key-value pairs "John": 5000
and "Jane": 6000
will be stored in the map. Note that if a key already exists in the map, assigning a new value will update the existing value.
Accessing Elements in a Map
To access elements in a map, you can use the following syntax:
value = mapName[key]
Here, mapName
is the name of the map, and key
is the key of the element you want to access. Let's see an example:
johnSalary := employeeSalary["John"]
In the above example, we're accessing the value associated with the key "John"
in the employeeSalary
map. The value 5000
will be assigned to the variable johnSalary
. Note that if the key doesn't exist in the map, the zero value of the value type will be returned.
Deleting Elements from a Map
To delete elements from a map in Go, you can use the built-in delete
function. Here's the syntax:
delete(mapName, key)
Let's consider the following example:
delete(employeeSalary, "John")
In the above example, we're deleting the key-value pair associated with the key "John"
from the employeeSalary
map. After executing this statement, the map will no longer contain the key "John"
and its corresponding value.
Iterating Over a Map
Go provides a built-in range
keyword that allows you to iterate over the keys and values of a map. Here's how you can use it:
for key, value := range mapName {
// Do something with key and value
}
Let's see an example:
for employee, salary := range employeeSalary {
fmt.Println(employee, "earns", salary)
}
In the above example, we're using the range
keyword to iterate over the elements of the employeeSalary
map. On each iteration, the employee
variable will hold the key, and the salary
variable will hold the corresponding value. We're then printing the employee name and their salary.
Checking if a Key Exists in a Map
To check if a key exists in a map, Go provides a two-value assignment form of a map lookup. Here's the syntax:
value, ok = mapName[key]
The variable value
will hold the value associated with the key, and the variable ok
will indicate if the key exists in the map. If the key exists, ok
will be set to true
; otherwise, it will be set to false
. Let's see an example:
salary, exists := employeeSalary["John"]
if exists {
fmt.Println("John's salary is", salary)
} else {
fmt.Println("John's salary not found")
}
In the above example, we're checking if the key "John"
exists in the employeeSalary
map. If it exists, we're printing the salary of John; otherwise, we're printing a message stating that John's salary was not found.
Map Literals
In addition to using the make
function to initialize a map, you can also use map literals to create and initialize a map in one line. Here's the syntax:
mapName := map[keyType]valueType{
key1: value1,
key2: value2,
// More key-value pairs
}
Let's consider the following example:
employeeSalary := map[string]int{
"John": 5000,
"Jane": 6000,
}
In the above example, we're using a map literal to create and initialize the employeeSalary
map. The key-value pairs "John": 5000
and "Jane": 6000
will be stored in the map.
Map Size
To get the number of key-value pairs in a map, you can use the built-in len
function. Here's an example:
size := len(employeeSalary)
In the above example, we're using the len
function to determine the number of elements in the employeeSalary
map. The size of the map will be stored in the size
variable.
Nil Maps
In Go, a map can be nil
. If a map is nil
, attempting to access, insert, or delete elements from it will result in a runtime panic. To work with a map, it must be initialized using the make
function or a map literal.
Conclusion
Congratulations! You've reached the end of this Go by Example tutorial on maps. By now, you should have a solid understanding of how to define, initialize, manipulate, and iterate over maps in Go. Maps are a versatile tool for associative mapping, and they provide efficient key-based lookup operations. If you have any questions or want to further explore the topic, feel free to refer to the official Go documentation. Happy coding!