Go by Example: Switch
The switch statement in Go is a powerful control structure that allows you to perform different actions based on the value of an expression. Learn its syntax, use cases, and best practices in this tutorial. Simplify complex logic and enhance code readability with the switch statement in Go.
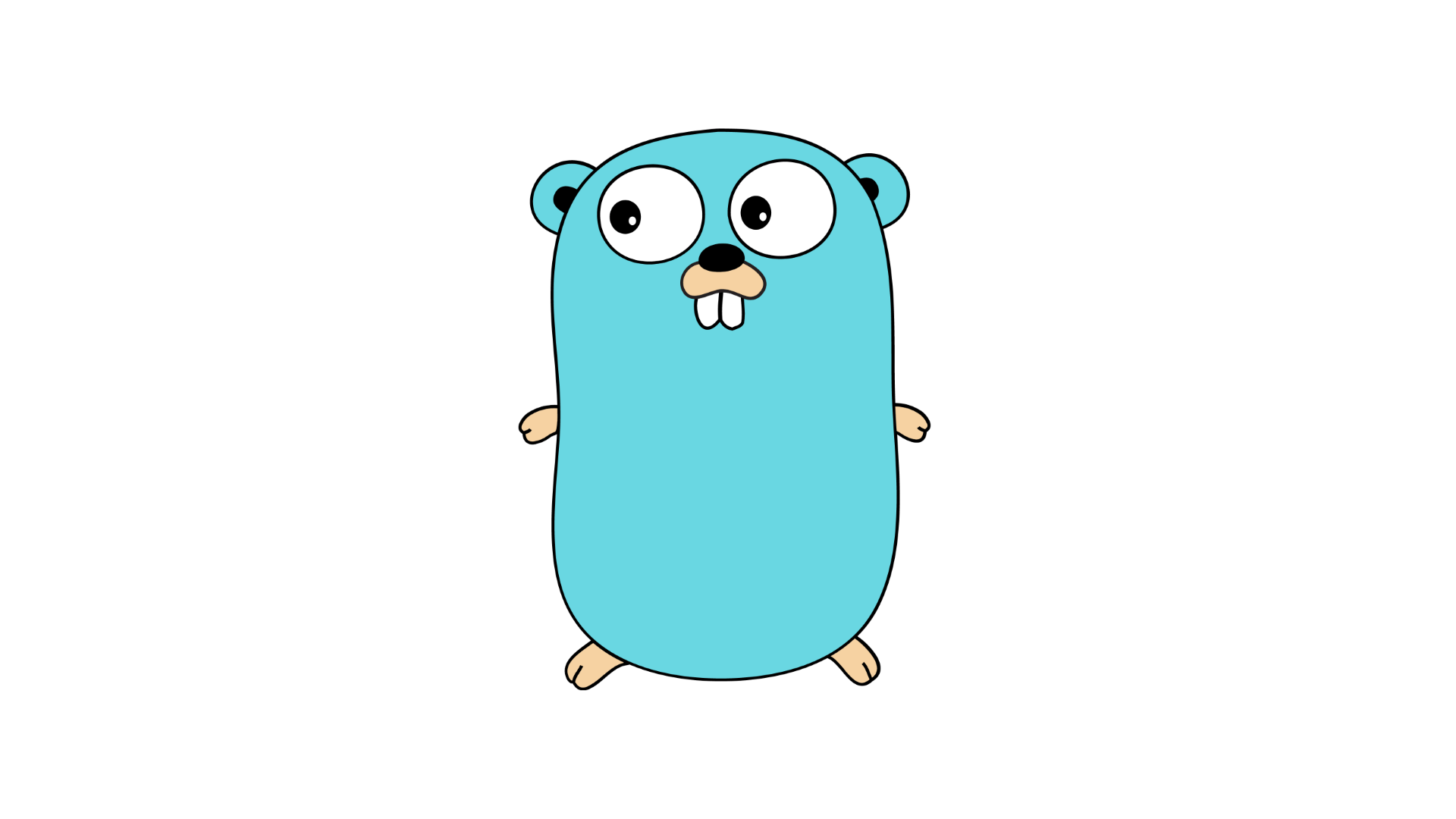
Introduction
In Go programming, the switch statement is a powerful control structure that allows you to perform different actions based on the value of an expression. The switch statement is a versatile tool that simplifies complex logic and enhances code readability. In this tutorial, we'll explore the switch statement in Go by diving into its syntax, various use cases, and best practices. Let's get started!
Switch Syntax
The basic syntax of the switch statement in Go is as follows:
```go switch expression { case value1: // code to be executed if expression equals value1 case value2: // code to be executed if expression equals value2 ... default: // code to be executed if expression doesn't match any value } ```
The expression inside the switch statement is evaluated, and Go checks each case to determine if it matches the value of the expression. If a case matches, the corresponding code block is executed. If none of the cases match, the code inside the default block is executed.
Switch with Single Expression
A switch statement in Go can be used with a single expression, which can be of any type, including strings, numbers, or even custom data types. Let's take a look at some examples:
Example 1: Using Switch with Integers
```go package main import "fmt" func main() { number := 2 switch number { case 0: fmt.Println("Number is 0") case 1: fmt.Println("Number is 1") case 2: fmt.Println("Number is 2") default: fmt.Println("Number is not 0, 1, or 2") } } ```
In this example, we have a variable `number` with a value of 2. The switch statement checks the value of `number` and executes the corresponding code block. Since `number` is 2, the output of this program will be:
``` Number is 2 ```
Example 2: Using Switch with Strings
```go package main import "fmt" func main() { fruit := "apple" switch fruit { case "apple": fmt.Println("It's an apple") case "banana": fmt.Println("It's a banana") default: fmt.Println("It's a fruit") } } ```
In this example, we have a variable `fruit` with a value of "apple". The switch statement checks the value of `fruit` and executes the appropriate code block based on the matching case. Since `fruit` is "apple", the output of this program will be:
``` It's an apple ```
Switch with Multiple Expressions
Go allows you to use multiple expressions in a switch statement. This can be helpful when you want to perform different actions based on the combination of multiple values. Let's see an example:
```go package main import "fmt" func main() { day := "Wednesday" isWeekend := false switch day { case "Saturday", "Sunday": isWeekend = true default: isWeekend = false } if isWeekend { fmt.Println("It's the weekend!") } else { fmt.Println("It's a weekday!") } } ```
In this example, the switch statement checks the value of `day` and sets the value of `isWeekend` based on whether it's a weekend day (Saturday or Sunday) or a weekday. The code then prints a message based on the value of `isWeekend`. Since `day` is "Wednesday", the output of this program will be:
``` It's a weekday! ```
Fallthrough Statement
In Go, the fallthrough statement is used to transfer control to the next case after the current case executes. By default, Go's switch statement exits after executing the code block of the matching case. However, if you want to execute code from multiple cases, you can use the fallthrough statement. Let's see an example:
```go package main import "fmt" func main() { number := 3 switch number { case 1: fmt.Println("Number is 1") fallthrough case 2: fmt.Println("Number is 2") fallthrough case 3: fmt.Println("Number is 3") default: fmt.Println("Number is not 1, 2, or 3") } } ```
In this example, the switch statement checks the value of `number` and executes the code blocks for cases 1, 2, and 3. The fallthrough statement transfers control to the next case, even if it doesn't match the value of `number`. The output of this program will be:
``` Number is 3 ```
Best Practices
To make the most of the switch statement in Go, consider the following best practices:
- Always include a
default
case to handle unexpected or unmatched values. - A case doesn't require a constant value. You can use variables or even functions as case values.
- Don't overuse the fallthrough statement. It can make the code harder to understand and maintain.
- Group similar cases together to improve code readability. For example, if you have multiple cases with similar actions, you can combine them using commas.
Conclusion
Congratulations! You now have a good understanding of the switch statement in Go. You've learned its syntax, how to use it with single and multiple expressions, and some best practices. The switch statement is a valuable tool for controlling flow and simplifying complex logic in your Go programs. Start using it in your own projects and experiment with different scenarios and expressions. Happy coding!