Exploring the Role of gRPC in Cloud-Native Architectures
Discover the power of gRPC in cloud-native architectures. With efficient communication, scalability, and ease of use, gRPC simplifies development, improves performance, and enhances the user experience. Unleash the potential of cloud-native systems with gRPC!
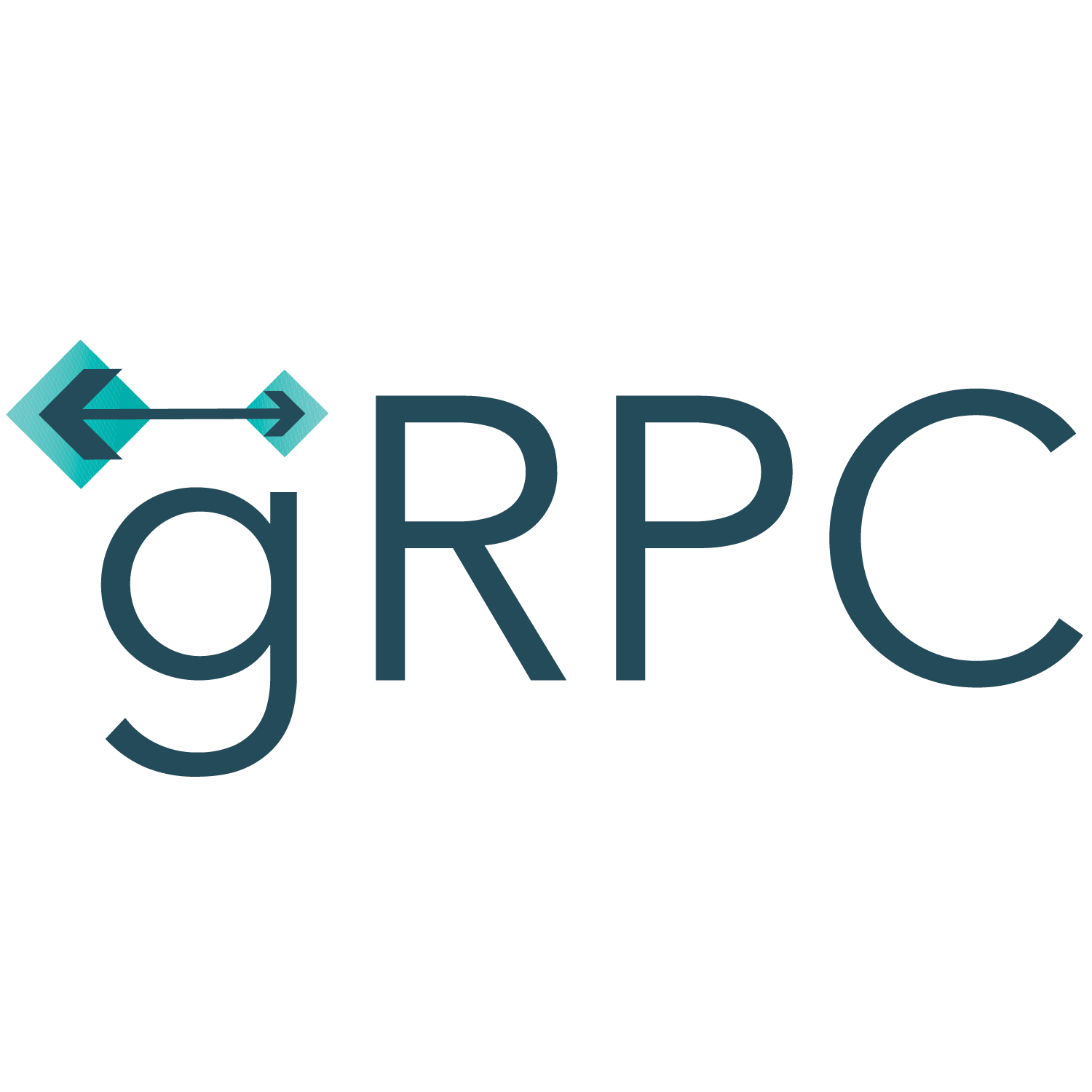
Exploring the Role of gRPC in Cloud-Native Architectures
Exploring the Role of gRPC in Cloud-Native Architectures
Welcome to our blog post on the role of gRPC in cloud-native architectures. In this post, we will dive into the world of gRPC and understand how it can empower your cloud-native applications. By the end of this article, you will have a clear understanding of the benefits of using gRPC and how it can enhance the performance and scalability of your cloud-native systems.
What is gRPC?
gRPC is an open-source, high-performance remote procedure call (RPC) framework developed by Google. It enables efficient communication between distributed systems using a simple and extensible interface definition language (IDL) called Protocol Buffers.
gRPC offers a wide range of features that make it an ideal choice for building cloud-native architectures, such as:
- Efficiency: gRPC uses Protocol Buffers, a language-agnostic binary serialization format that offers smaller message sizes and faster serialization and deserialization compared to other formats like JSON or XML. This efficiency is crucial for cloud-native applications that need to handle large amounts of data.
- Strong Typing: With Protocol Buffers, you can define the structure of your messages using a schema, which allows for strong typing. This ensures that both the client and server agree on the structure of the data, reducing the risk of errors and making it easier to evolve your APIs over time.
- Bidirectional Streaming: gRPC supports bidirectional streaming, allowing both the client and server to send and receive streams of messages. This feature is useful for real-time applications, such as chat applications or IoT systems, where both sides need to send data asynchronously.
- Code Generation: gRPC generates client and server code from your service definition, reducing the amount of boilerplate code you need to write. This not only saves development time but also ensures that both the client and server implementations are consistent and up-to-date.
- Load Balancing and Service Discovery: gRPC integrates seamlessly with popular service discovery and load balancing solutions, such as Kubernetes and Istio. This makes it easy to deploy your gRPC services in a cloud-native environment and distribute traffic efficiently across multiple instances.
How Does gRPC Fit into Cloud-Native Architectures?
In cloud-native architectures, applications are typically composed of multiple microservices that communicate with each other over the network. gRPC provides a fast and efficient way to connect these microservices, enabling them to exchange data and collaborate seamlessly.
Here's how gRPC fits into different layers of a typical cloud-native architecture:
1. Client-Side Libraries
On the client side, gRPC provides client libraries for various programming languages, including Go, Python, Java, and C#. These client libraries enable developers to consume gRPC services easily. They abstract away the complexity of networking and serialization, allowing you to focus on your application logic.
2. Service Definition
With gRPC, you define your service interface using Protocol Buffers. This service definition acts as a contract between the client and server, specifying the available RPC methods and their parameters. gRPC uses this definition to generate client and server stubs, which you can use to implement your service logic.
3. Server Implementation
On the server side, gRPC provides server libraries that handle the incoming requests and delegate them to the appropriate service implementation. These server libraries take care of the network communication, message serialization, and other low-level details, allowing you to focus on your business logic.
4. Scalable Deployment
gRPC plays well with cloud-native deployment options like Docker and Kubernetes. You can package your gRPC services into containers, deploy them to a container orchestration platform, and easily scale them horizontally to handle increased traffic. gRPC also supports advanced load balancing techniques, such as weighted round-robin, to distribute traffic evenly across multiple instances.
Getting Started with gRPC
Ready to get started with gRPC? Here are the steps to set up a basic gRPC project:
1. Define your service interface
Create a Protocol Buffers (.proto) file and define your service interface and message structures. Specify the RPC methods, their parameters, and return types. This service definition will act as the contract between the client and server.
syntax = "proto3";
package example;
service UserService {
rpc GetUser(GetUserRequest) returns (UserResponse) {}
rpc CreateUser(CreateUserRequest) returns (UserResponse) {}
}
message GetUserRequest {
string user_id = 1;
}
message CreateUserRequest {
string name = 1;
string email = 2;
}
message UserResponse {
string id = 1;
string name = 2;
string email = 3;
}
2. Generate client and server code
Use the protoc compiler to generate client and server code from your .proto file. The generated code will include client and server stubs that you can use as a starting point for your implementation.
$ protoc --go_out=. --go-grpc_out=. example.proto
3. Implement your service logic
Write the server-side implementation of your gRPC service. Use the generated server stub and implement the RPC methods. You can define custom logic, access databases or external services, and return appropriate responses.
type Server struct{}
func (s *Server) GetUser(ctx context.Context, req *example.GetUserRequest) (*example.UserResponse, error) {
// Retrieve user from the database
user := getUserFromDatabase(req.UserId)
// Create a response
response := &example.UserResponse{
Id: user.Id,
Name: user.Name,
Email: user.Email,
}
return response, nil
}
func (s *Server) CreateUser(ctx context.Context, req *example.CreateUserRequest) (*example.UserResponse, error) {
// Create a new user in the database
newUser := createUserInDatabase(req.Name, req.Email)
// Create a response
response := &example.UserResponse{
Id: newUser.Id,
Name: newUser.Name,
Email: newUser.Email,
}
return response, nil
}
4. Create a client
Use the generated client code to create a gRPC client and connect to your service. You can then use the client to invoke the RPC methods defined in your service interface.
func main() {
conn, err := grpc.Dial("localhost:50051", grpc.WithInsecure())
if err != nil {
log.Fatalf("Failed to connect: %v", err)
}
defer conn.Close()
client := example.NewUserServiceClient(conn)
// Invoke RPC methods
// ...
log.Println("Client connected to gRPC server")
}
Conclusion
gRPC is a powerful framework for building cloud-native architectures. Its efficient communication protocol, scalability, and ease of use make it a perfect choice for modern distributed systems. By embracing gRPC, you can simplify the development and deployment of your microservices, improve performance, and provide a seamless experience to your users.
So, what are you waiting for? Start exploring gRPC and unlock the full potential of cloud-native architectures!