Exploring Golang's Standard Library: Essential Packages and Modules
Discover the essential packages and modules in Golang's Standard Library, covering input/output operations, networking, concurrency, data structures, cryptography, and more. Explore the power of Golang with these optimized tools for seamless development.
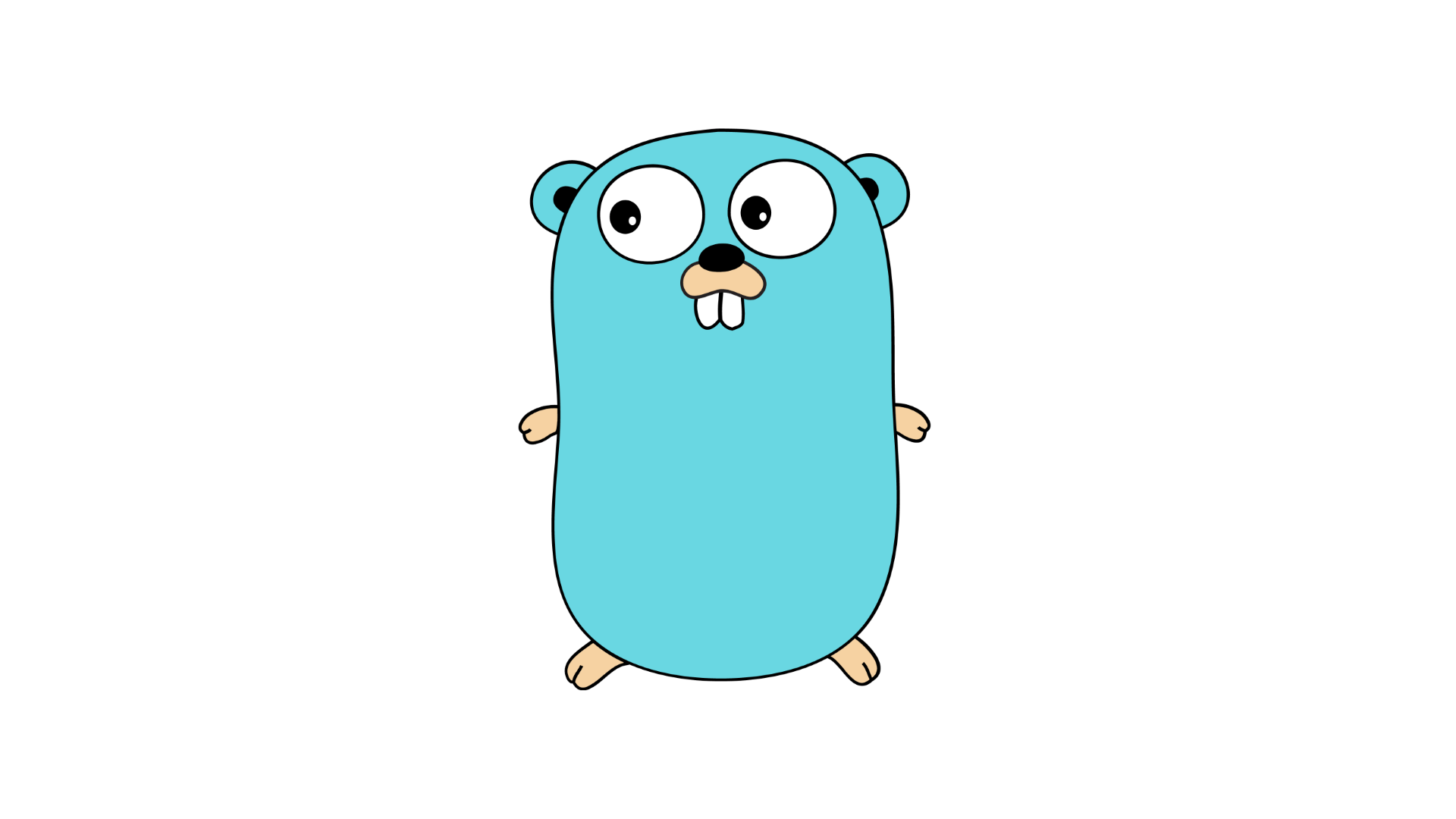
Introduction
Welcome to the world of Go (Golang)! In this blog post, we will explore the essential packages and modules offered by Go's standard library. Understanding these packages is crucial for every beginner as they provide the building blocks for developing efficient and robust applications. So, let's dive in and explore Golang's standard library!
Overview of Golang's Standard Library
Before we delve into the essential packages and modules, let's take a moment to understand the importance of Go's standard library. The standard library is a collection of pre-built packages that encompass a wide range of functionalities, from input/output operations to networking, concurrency, data structures, and cryptography.
Importance of Essential Packages and Modules
Why are these essential packages and modules important, you may ask? Well, they serve as the foundation for a smooth and efficient development process. By leveraging these packages, you can save time and effort by avoiding reinventing the wheel. Let's explore some of the essential packages and modules that will help you kickstart your journey with Go.
Basic Input/Output Operations
1. fmt package
The fmt
package is a powerful tool for handling input and output operations in Go. It provides various functions for formatting output using verbs and scanning input using functions like Scan, Scanf, and Scanln. Let's see some code samples to understand this better:
``` package main import "fmt" func main() { fmt.Println("Hello, World!") // Prints Hello, World! var name string fmt.Print("Enter your name: ") fmt.Scan(&name) fmt.Printf("Nice to meet you, %s!\n", name) } ```
2. bufio package
The bufio
package is useful for buffered input/output operations, making file reading and writing more efficient. It provides functions like NewScanner, NewWriter, and NewReader to work with files and streams. Here's an example:
``` package main import ( "bufio" "fmt" "os" ) func main() { file, err := os.Open("input.txt") if err != nil { fmt.Println("Error opening file:", err) return } defer file.Close() scanner := bufio.NewScanner(file) for scanner.Scan() { line := scanner.Text() fmt.Println(line) } if err := scanner.Err(); err != nil { fmt.Println("Error reading file:", err) } } ```
3. os package
The os
package provides functions for interacting with the operating system, including file and directory operations, environment variables, and more. Let's see an example:
``` package main import ( "fmt" "os" ) func main() { dir, err := os.Getwd() if err != nil { fmt.Println("Error getting current directory:", err) return } fmt.Println("Current directory:", dir) files, err := os.ReadDir(dir) if err != nil { fmt.Println("Error reading directory:", err) return } fmt.Println("Files in directory:") for _, file := range files { if file.IsDir() { fmt.Println("[DIR]", file.Name()) } else { fmt.Println(file.Name()) } } fmt.Println("User home directory:", os.Getenv("HOME")) } ```
Networking and HTTP
1. net package
The net
package provides various functions and types for network operations. It allows you to work with TCP/IP, UDP, and Unix domain sockets. Here's an example of establishing a TCP server:
``` package main import ( "fmt" "net" ) func main() { ln, err := net.Listen("tcp", ":8080") if err != nil { fmt.Println("Error creating listener:", err) return } defer ln.Close() fmt.Println("Listening on port 8080...") for { conn, err := ln.Accept() if err != nil { fmt.Println("Error accepting connection:", err) continue } go handleConnection(conn) } } func handleConnection(conn net.Conn) { defer conn.Close() // Handle incoming connections here // ... } ```
2. http package
The http
package provides functions for creating HTTP servers and clients, handling HTTP requests and responses, and more. Here's an example of a simple HTTP server:
``` package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Hello, World!") }) err := http.ListenAndServe(":8080", nil) if err != nil { fmt.Println("Error starting server:", err) } } ```
Concurrency and Parallelism
1. sync package
The sync
package provides synchronization primitives for managing shared resources in concurrent Go programs. It includes Mutexes, WaitGroups, Cond variables, and more. Here's an example using a Mutex to protect a shared resource:
``` package main import ( "fmt" "sync" "time" ) var count int var mutex sync.Mutex func main() { var wg sync.WaitGroup for i := 0; i < 5; i++ { wg.Add(1) go increment(&wg) } wg.Wait() fmt.Println("Final count:", count) } func increment(wg *sync.WaitGroup) { defer wg.Done() mutex.Lock() defer mutex.Unlock() count++ time.Sleep(time.Millisecond) // Simulate some work } ```
2. go package
The go
package allows you to launch goroutines for concurrent execution and use channels for communication between goroutines. Here's an example of a simple producer-consumer pattern using goroutines and channels:
``` package main import ( "fmt" "time" ) func main() { jobs := make(chan int) results := make(chan int) go producer(jobs) go consumer(jobs, results) for result := range results { fmt.Println(result) } } func producer(jobs chan<- int) { for i := 0; i < 5; i++ { jobs <- i time.Sleep(time.Millisecond) // Simulate some work } close(jobs) } func consumer(jobs <-chan int, results chan<- int) { for job := range jobs { results <- job * 2 } close(results) } ```
Data Structures and Utilities
1. container package
The container
package provides efficient container data structures like lists, heaps, and ring buffers. Let's see an example of working with a list:
``` package main import ( "container/list" "fmt" ) func main() { l := list.New() l.PushBack(1) l.PushBack(2) l.PushBack(3) for e := l.Front(); e != nil; e = e.Next() { fmt.Println(e.Value) } } ```
2. sort package
The sort
package provides sorting and searching operations on various data types. It also allows custom sorting with user-defined functions. Here's an example of sorting integers in ascending order:
``` package main import ( "fmt" "sort" ) func main() { numbers := []int{4, 2, 7, 5, 1} sort.Ints(numbers) fmt.Println(numbers) // Prints [1 2 4 5 7] } ```
3. strconv package
The strconv
package provides functions for converting between strings and basic data types, as well as handling numeric conversions. Here's an example:
``` package main import ( "fmt" "strconv" ) func main() { num := 42 str := strconv.Itoa(num) fmt.Println(str) // Prints "42" str2 := "3.14" num2, err := strconv.ParseFloat(str2, 64) if err != nil { fmt.Println("Error parsing float:", err) return } fmt.Println(num2) // Prints 3.14 } ```
Cryptography and Security
1. crypto package
The crypto
package provides cryptographic primitives, including hash functions, symmetric and asymmetric encryption, and more. Here's a simple example of hashing a password:
``` package main import ( "crypto/sha256" "fmt" ) func main() { password := "secretpassword" hash := sha256.Sum256([]byte(password)) fmt.Printf("Hashed password: %x\n", hash) } ```
2. encoding package
The encoding
package allows encoding and decoding data formats like JSON, XML, and more. It also provides functionality for Base64 and binary encoding. Here's an example of encoding and decoding JSON:
``` package main import ( "encoding/json" "fmt" ) type Person struct { Name string `json:"name"` Age int `json:"age"` } func main() { person := Person{ Name: "John Doe", Age: 30, } // Encoding to JSON jsonBytes, err := json.Marshal(person) if err != nil { fmt.Println("Error encoding to JSON:", err) return } fmt.Println(string(jsonBytes)) // Decoding from JSON var decodedPerson Person err = json.Unmarshal(jsonBytes, &decodedPerson) if err != nil { fmt.Println("Error decoding from JSON:", err) return } fmt.Println(decodedPerson) } ```
Summary
Congratulations on exploring Golang's standard library and its essential packages and modules! In this blog post, we covered basic input/output operations with the fmt, bufio, and os packages, networking and HTTP with the net and http packages, concurrency and parallelism with the sync and go packages, data structures and utilities with the container, sort, and strconv packages, and cryptography and security with the crypto and encoding packages.
By utilizing these packages, you can develop efficient and robust applications in Go. Remember to continue experimenting and exploring the rich offerings of Go's standard library as you progress in your journey as a Golang developer.
Stay tuned for the next part of our tutorial series, where we'll further explore Go package management and delve into effective dependency management. Happy coding!