Exploring Golang's Reflection: Dynamic Typing and Metaprogramming
In this blog post, we explore the powerful capabilities of Golang's reflection, including dynamic typing and metaprogramming. Discover how to manipulate code and data structures at runtime, making your applications more flexible and extensible.
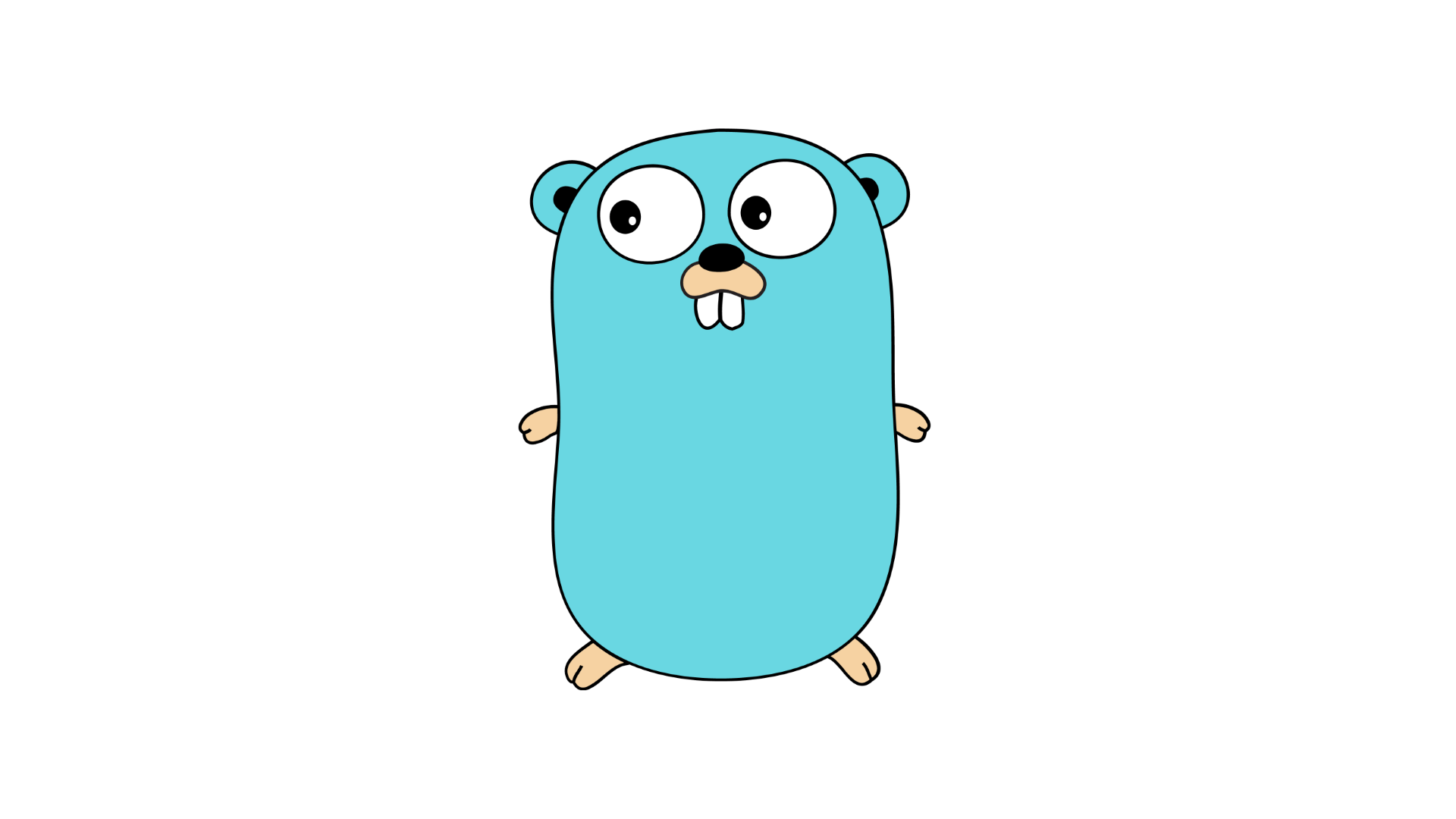
Introduction
Golang, also known as Go, is a popular programming language known for its simplicity, efficiency, and concurrency capabilities. In this article, we will explore two important aspects of Go - reflection and metaprogramming. These features allow Golang to dynamically examine and manipulate its own code and data structures at runtime, making it a powerful language for building flexible and extensible applications.
What is Reflection?
Reflection is a powerful feature in Golang that enables programs to examine and manipulate their own code and data structures at runtime. It allows Go programs to introspect their types, values, and methods, providing a way to dynamically access and modify them.
Dynamic Typing
One of the key benefits of reflection in Go is dynamic typing. Unlike statically typed languages, where types are determined at compile time, Go allows developers to work with types dynamically. This means that you can write code that can handle different types of variables without explicitly knowing their types in advance.
Metaprogramming
Metaprogramming is another powerful capability provided by reflection in Go. It allows developers to write code that can generate or modify other code. This opens up possibilities for dynamically creating functions, methods, and data structures at runtime, giving you the ability to build complex and flexible systems.
How Reflection Works in Go
Under the hood, Go's reflection is based on the reflect
package, which provides a set of functions and types for working with types and values at runtime. The reflect
package allows you to inspect and manipulate types, values, fields, methods, and even function calls.
Examples of Reflection in Go
Let's look at some practical examples of using reflection in Go:
Example 1: Dynamically Creating Structs
With reflection, you can create new instances of structs dynamically, based on given input or conditions. This can be useful when you need to create different types of objects based on runtime data.
Example 2: Invoking a Function Dynamically
Reflection also allows you to call functions dynamically. You can obtain a function's value using reflection and then invoke it with dynamic arguments. This can be handy when you want to execute functions based on runtime conditions.
Example 3: Extracting Field and Method Information
The reflect
package also provides functions to extract information about fields and methods of a struct. This information can be used, for example, to perform serialization or find out what methods are available on a particular object at runtime.
Best Practices for Using Reflection
While reflection can be a powerful tool in your Go toolbox, it should be used judiciously. Here are a few best practices to keep in mind:
Use Reflection Sparingly
Reflection can add complexity and overhead to your code. It's generally recommended to use reflection only when there's no other alternative.
Be Aware of Performance Implications
Reflection operations are slower compared to direct static code. If performance is critical, consider using other techniques to avoid reflection.
Ensure Type Safety
Reflection bypasses the type system, so you should take extra care to ensure type safety when working with reflection. Incorrect use of reflection can lead to runtime errors that can be difficult to debug.
Conclusion
Reflection and metaprogramming are powerful tools in Go that enable developers to write flexible and extensible code. With reflection, you can dynamically examine and modify code and data structures at runtime, opening up new possibilities for building complex and adaptable applications.
By understanding how reflection works and following best practices, you can leverage this feature effectively in your Go projects. So go ahead, explore the world of reflection in Golang, and unlock new levels of programming productivity!