Exploring Golang's Interfaces: Design Patterns and Use Cases
Discover the power of Golang's interfaces. Explore design patterns and use cases that promote code decoupling, flexibility, and reusability. Maximize the potential of your applications with modular and maintainable code.
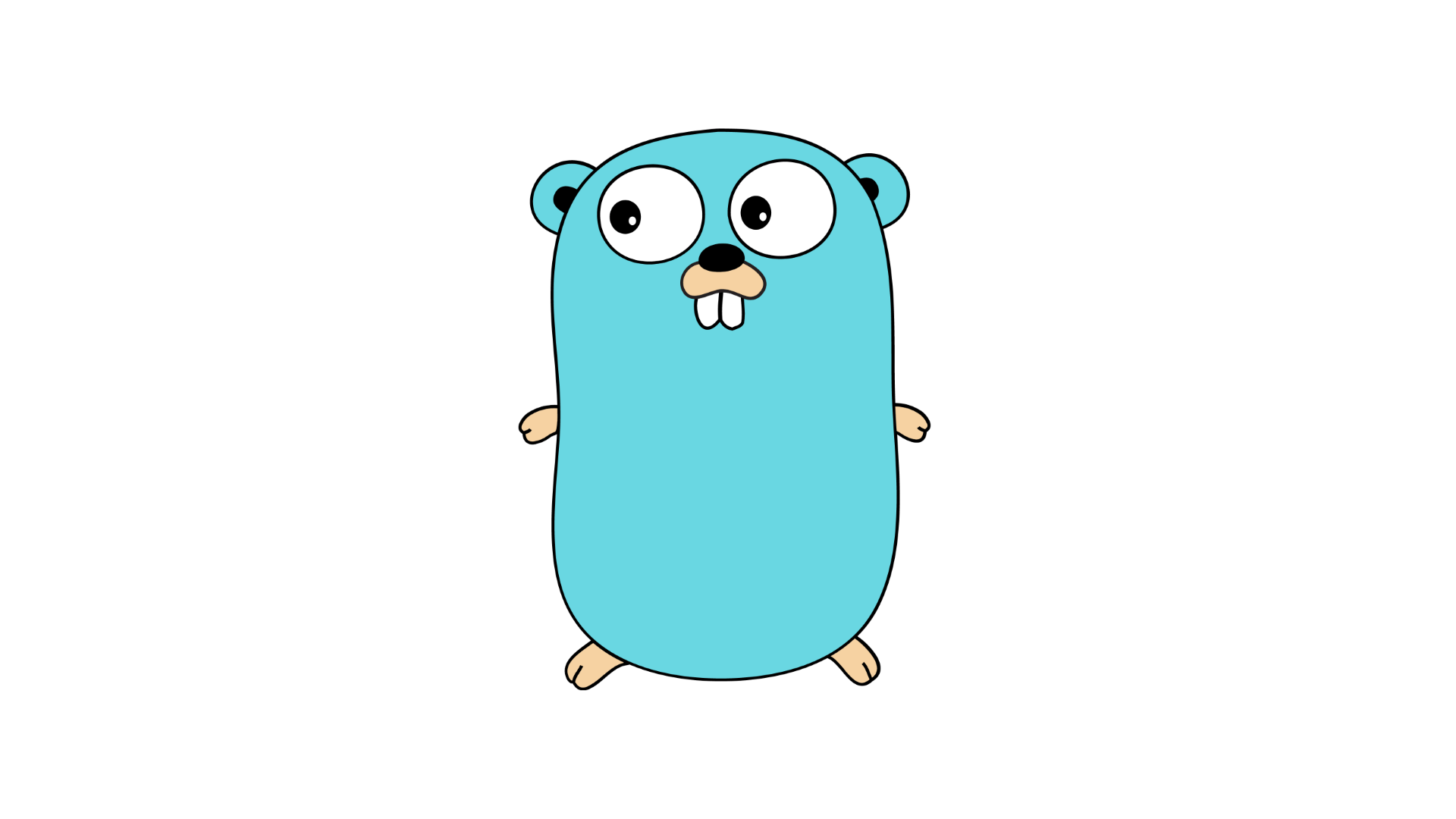
Exploring Golang's Interfaces: Design Patterns and Use Cases
Go (Golang) is a powerful and efficient programming language known for its simplicity and concurrency support. One of the key features that makes Go so versatile is its use of interfaces. Interfaces in Go provide a way to define a set of methods that a type must implement, allowing for flexible and reusable code.
In this section, we will explore the design patterns and use cases for interfaces in Go. Understanding these concepts will help you write more modular and maintainable code.
What Are Interfaces in Go?
In Go, an interface is a collection of method signatures. It defines a set of behaviors that a type must implement. Any type that satisfies the interface's method signatures is said to implement the interface.
Interfaces provide a way to achieve polymorphism in Go. They allow you to write code that is independent of specific types, making your code more flexible and extensible.
Design Patterns and Use Cases for Interfaces
1. Decoupling Dependencies
One of the key use cases for interfaces in Go is decoupling dependencies. By using interfaces, you can define an abstraction for a dependency and then write code that depends on the interface rather than a specific implementation.
This enables you to easily swap out different implementations of a dependency without changing any of the code that depends on it. This makes your code more modular and allows for easier testing and code maintenance.
2. Implementing the Strategy Pattern
The strategy pattern is a design pattern that allows you to define a family of algorithms, encapsulate each one as an object, and make them interchangeable. Interfaces in Go make it easy to implement the strategy pattern.
You can define an interface that represents a particular algorithm and then provide different implementations of that interface. By writing code that depends on the interface, you can switch between different algorithms at runtime without modifying the code that uses the algorithm.
3. Creating Mock Objects for Unit Testing
Interfaces are invaluable when it comes to unit testing. By defining interfaces for dependencies, you can create mock implementations of those interfaces for testing purposes.
Mock objects allow you to simulate the behavior of dependencies, making it easier to test your code in isolation. By using interfaces, you can easily swap out the real implementation with a mock implementation during testing.
4. Implementing the Adapter Pattern
The adapter pattern is a design pattern that allows you to convert the interface of a class into another interface that clients expect. Interfaces in Go make it easy to implement the adapter pattern.
You can define an interface that represents the expected behavior and then provide an implementation that adapts the behavior of another interface. This allows you to use incompatible types together and provides a way to reuse existing code.
Summary
Interfaces in Go are a powerful tool for writing modular and reusable code. By decoupling dependencies, implementing design patterns, and creating mock objects, interfaces allow you to write flexible and maintainable code.
In this section, we explored the design patterns and use cases for interfaces in Go. Understanding these concepts will help you become a more proficient Go developer.
In the next section, we will delve deeper into the implementation details of interfaces in Go and explore some advanced techniques. Stay tuned!