Exploring Golang's Concurrency Features: A Deep Dive
Discover the power of Golang's concurrency features and how they can help you build efficient and scalable applications. Dive into goroutines, channels, select statements, and mutexes for parallelism and scalability.
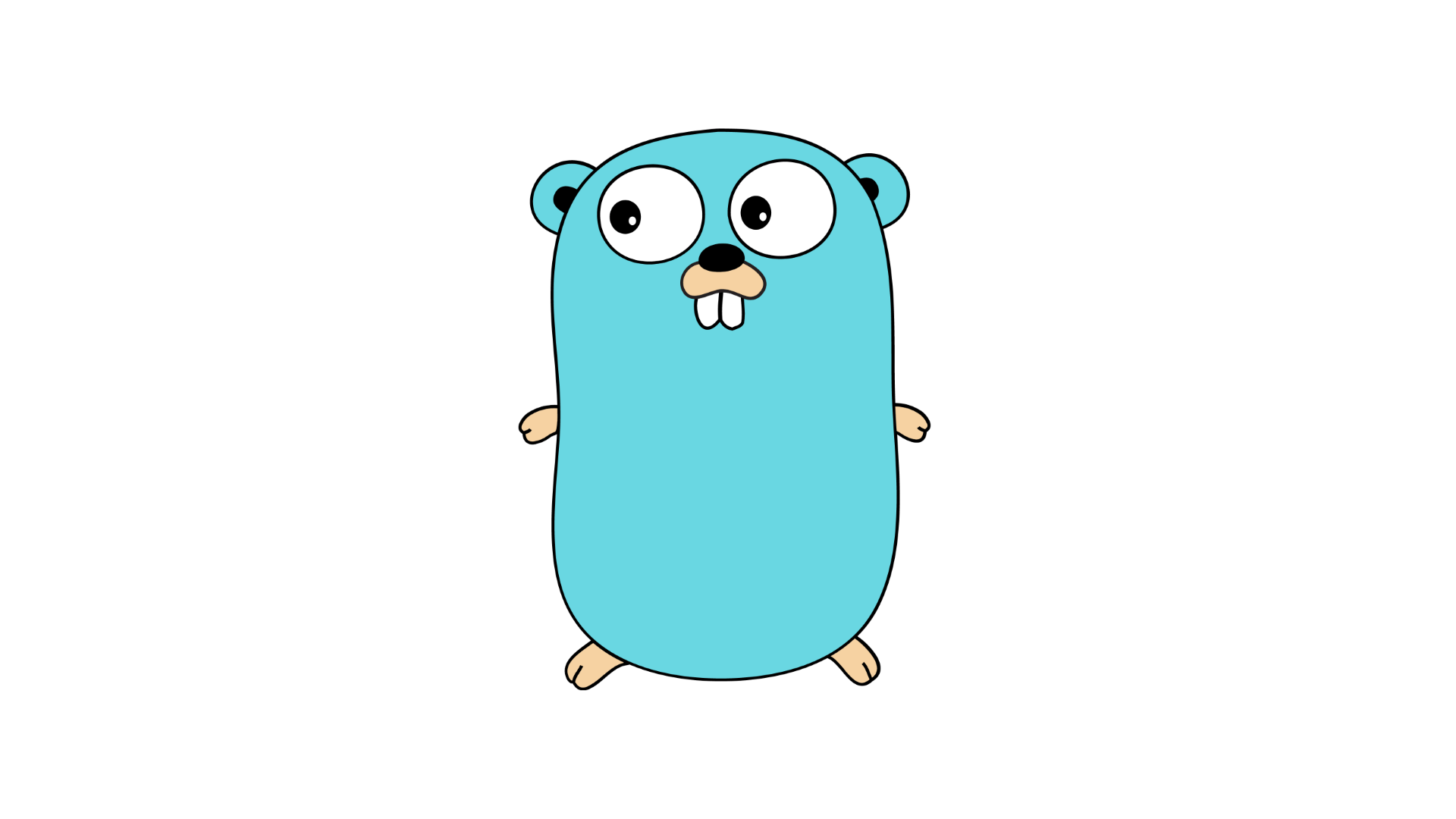
Exploring Golang's Concurrency Features: A Deep Dive
Golang, also known as Go, is a popular programming language known for its simplicity, efficiency, and built-in support for concurrency. Concurrency is the ability of a program to perform multiple tasks simultaneously. This feature is particularly useful for building high-performance applications that can handle multiple requests and tasks simultaneously.
In this article, we will take a deep dive into Golang's concurrency features and explore how they can be used to build efficient and scalable applications.
Goroutines
Goroutines are lightweight threads that allow you to execute functions concurrently. They are an integral part of Go's concurrent programming model and provide a simple and efficient way to achieve concurrency. Goroutines are much lighter than operating system threads and can be created and destroyed quickly, making them an ideal choice for handling concurrent tasks.
To create a goroutine, you simply need to prefix a function call with the keyword "go". The function call will then be executed concurrently in a separate goroutine.
go myFunction()
Channels
Channels are an essential component of Go's concurrency model and are used to facilitate communication and synchronization between goroutines. They provide a safe way to pass data between concurrent tasks and ensure that access to shared data is synchronized.
A channel is defined using the "make" keyword and the "chan" keyword, followed by the data type that the channel will carry.
myChannel := make(chan int)
You can send data to a channel using the "<-<" operator and receive data from a channel using the ">->" operator.
// Sending data to a channel
myChannel <- 42
// Receiving data from a channel
data := <-myChannel
Select Statement
The select statement is used to handle multiple channel operations concurrently. It allows you to listen for events from multiple channels and perform the corresponding actions when any of the channel operations are ready.
select {
case <-channel1:
// handle event from channel1
case <-channel2:
// handle event from channel2
default:
// handle default case
}
Mutex
In Go, the mutex, short for mutual exclusion, is a synchronization primitive used to prevent multiple goroutines from accessing shared resources simultaneously. It ensures that only one goroutine can access the critical section of the code at a time, preventing race conditions and data corruption.
var mutex sync.Mutex
func myFunction() {
mutex.Lock()
// Critical section
mutex.Unlock()
}
Conclusion
Golang's concurrency features, including goroutines, channels, select statements, and mutexes, provide powerful tools for building efficient and scalable applications. By leveraging these features, you can take advantage of the inherent parallelism of modern hardware and build high-performance applications that can handle multiple tasks concurrently.
By understanding and mastering Golang's concurrency features, you'll be able to write concurrent programs that are robust, efficient, and scalable. Happy coding!