Exploring Angular Modules: Organizing Your Angular Application
Learn how to effectively organize your Angular application using modules. Discover the benefits of feature modules, shared modules, and core modules, and explore the power of lazy loading for optimizing performance.
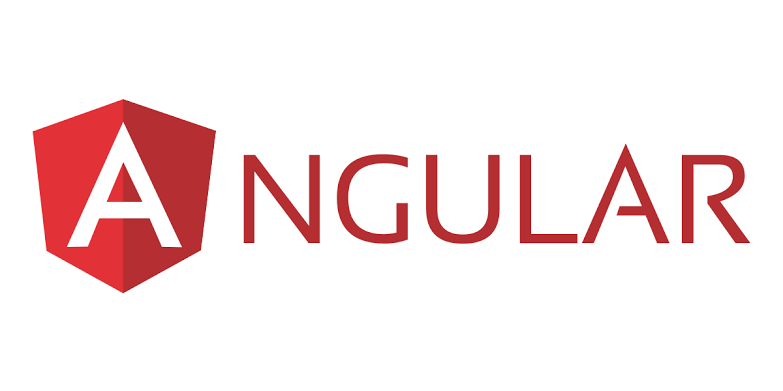
Angular is a powerful front-end framework that allows you to build complex and scalable web applications. As your application grows, it becomes essential to organize your codebase effectively. Angular modules provide a modular structure that helps you manage your application's components, services, and other resources efficiently. In this article, we will explore Angular modules and learn how to organize your Angular application using modules.
Introduction to Angular Modules
An Angular module is a collection of components, directives, pipes, services, and other resources that are related to a specific feature or functionality of an application. It acts as a container that encapsulates all the code and dependencies required for that feature. Angular modules promote code reusability, modularity, and maintainability by allowing you to separate your application into distinct, self-contained units.
Creating an Angular Module
To create a new Angular module, you can use the Angular CLI command:
ng generate module module-name
This command generates a new module file with the name module-name.module.ts
in your project's src/app directory. The generated module file is a TypeScript file that exports a class annotated with the @NgModule
decorator.
Let's take a closer look at the structure of an Angular module file:
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { MyComponent } from './my-component.component';
import { MyService } from './my-service.service';
@NgModule({
declarations: [MyComponent],
imports: [CommonModule],
providers: [MyService]
})
export class MyModule { }
The @NgModule
decorator is used to define the metadata for the module. The declarations
property is an array that includes all the components, directives, and pipes that belong to the module. The imports
property is an array that includes other modules required by the current module. The providers
property is an array that includes the services provided by the module.
In the example above, we have a module named MyModule
that exports a component MyComponent
and uses the MyService
service. It also imports the CommonModule
module, which provides common directives and pipes.
Using an Angular Module
Once you have created a module, you can use it in your application by importing it into another module. For example, let's say you have a module named MyModule
and you want to use its component in another module named AppModule
:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { MyModule } from './my-module/my-module.module';
@NgModule({
declarations: [],
imports: [BrowserModule, MyModule],
providers: [],
bootstrap: []
})
export class AppModule { }
In the example above, we import the MyModule
module into the AppModule
module using the imports
property. Now, the components, services, and other resources of the MyModule
module are available for use in the AppModule
.
Organizing Your Angular Application with Modules
Angular modules provide a powerful mechanism for organizing your application into modules, making it easier to manage and maintain. Here are some best practices for organizing your Angular application using modules:
Feature Modules
Feature modules represent specific features or functionalities of your application. They encapsulate all the related components, services, and other resources required for that feature. By creating feature modules, you can keep your codebase modular and reusable, making it easier to add or remove features as your application evolves.
Shared Module
The shared module is a module that contains components, directives, and pipes that are shared across multiple feature modules. It promotes code reusability and helps avoid duplication of code. By creating a shared module, you can centralize the common resources used by multiple modules.
Core Module
The core module contains singleton services that are used throughout the application. It provides a centralized place to manage and inject these services. By creating a core module, you can separate the core functionality of your application from the feature-specific functionality.
Lazy Loading Modules
Lazy loading is a technique that allows you to load modules on demand, rather than loading them all at once when the application starts. This can significantly improve the initial load time of your application, especially if you have large and complex modules. By lazy loading modules, you can optimize the performance of your application and enhance the user experience.
Conclusion
Angular modules play a crucial role in organizing your Angular application effectively. By creating feature modules, shared modules, and core modules, you can keep your codebase modular, reusable, and maintainable. Additionally, by leveraging lazy loading, you can optimize the performance of your application. So, go ahead and start organizing your Angular application with modules to take full advantage of the power and flexibility of Angular!
Do you want to freshen up your knowledge on Angular CLI? Check out our article on Angular CLI: A Comprehensive Guide to learn more!