Event-Driven Microservices with gRPC and Apache Kafka
Explore the power of event-driven microservices with gRPC and Apache Kafka. Create decoupled systems for large-scale data processing and seamless integration.
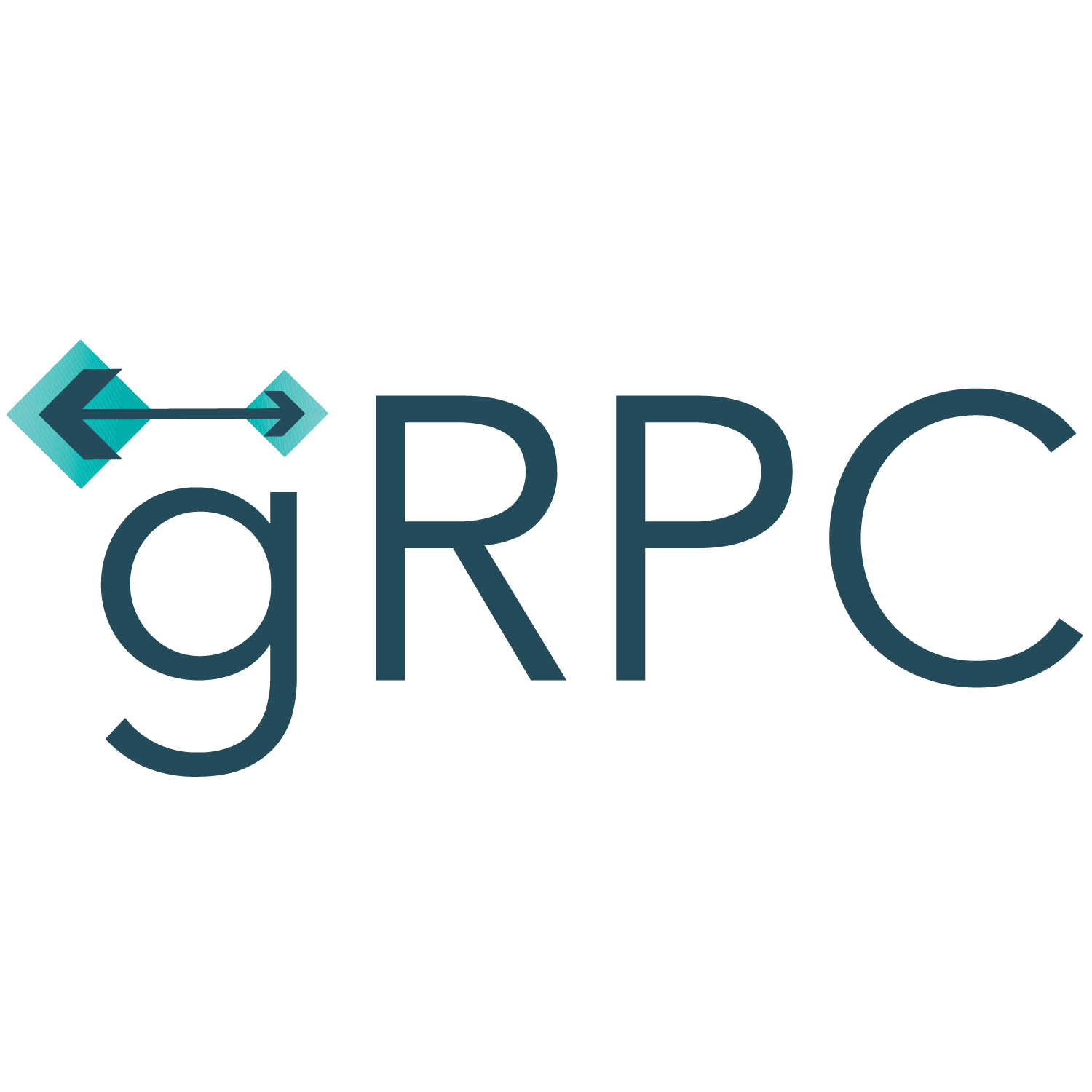
Event-Driven Microservices with gRPC and Apache Kafka
Welcome to the exciting world of event-driven microservices! In this blog post, we will explore the power of combining gRPC and Apache Kafka to build scalable and reliable microservice architectures. By leveraging event-driven communication patterns, we can create loosely coupled and highly decoupled systems that can handle large-scale data processing and seamlessly integrate with other services in your ecosystem.
Understanding Microservices Architecture
Before we dive into event-driven microservices, let's quickly recap what microservices are. Microservices architecture is a software design pattern where a complex application is broken down into smaller, independent services that work together to fulfill a business goal. Each service is responsible for a specific functionality and can be deployed, managed, and scaled independently.
The benefits of microservices architecture include increased development velocity, fault isolation, and scalability. However, as the number of services grows, managing inter-service communication becomes more challenging. This is where event-driven architecture comes in handy.
Event-Driven Architecture Overview
Event-driven architecture decouples services by using asynchronous messaging. Instead of services directly calling each other's APIs, they communicate through events. An event is a small, atomic, immutable piece of information that represents a specific occurrence or fact. Services can publish events to a central event bus, and other services can subscribe to these events to react and perform actions accordingly.
Event-driven architecture brings multiple benefits. It enables loose coupling, allowing services to evolve independently. It also provides fault tolerance and scalability, as services can process events asynchronously and at their own pace. With this architecture, you can build systems that are resilient, responsive, and scalable.
Introducing gRPC
gRPC is a modern, high-performance, open-source remote procedure call (RPC) framework that enables services to communicate with each other efficiently. gRPC uses the Protocol Buffers (protobuf) data serialization format, which provides a language-agnostic way to define and serialize structured data.
gRPC supports multiple programming languages, including Go, Java, Python, and C#, making it an excellent choice for building microservices in different technology stacks. It also provides advanced features like streaming, bidirectional communication, and authentication, making it a powerful tool for microservices communication.
Apache Kafka for Event Streaming
Apache Kafka is a distributed event streaming platform that provides a highly scalable, fault-tolerant, and durable publish-subscribe messaging system. Kafka is designed to handle high throughput and low latency data streaming, making it an ideal choice for building event-driven microservices.
In Kafka, events are stored in log-like structures called topics. Producers write events to topics, and consumers read events from topics. Kafka guarantees the durability of events for a configurable retention period, enabling event replay and fault tolerance.
Combining gRPC and Apache Kafka
Now that we understand the basics of gRPC and Apache Kafka, let's explore how we can combine them to build powerful event-driven microservices.
The typical flow of events in this architecture is as follows:
- A service publishes an event to a Kafka topic using a Kafka producer.
- Other services that are interested in the event subscribe to the Kafka topic and consume the event using a Kafka consumer.
- The consumed event triggers the execution of a gRPC call to another service, passing the event data and any additional parameters.
- The target service receives the gRPC call and performs the necessary actions based on the received event and parameters.
By leveraging this flow, we achieve a fully decoupled and scalable architecture that enables services to communicate asynchronously and efficiently.
Implementing gRPC with Kafka in Go
Let's take a look at a simple example of implementing event-driven microservices using gRPC and Kafka in Go:
package main
import (
"context"
"log"
"time"
"google.golang.org/grpc"
"github.com/segmentio/kafka-go"
)
type Event struct {
Message string
}
type EventPublisher struct {
producer *kafka.Writer
}
func NewEventPublisher() *EventPublisher {
return &EventPublisher{
producer: kafka.NewWriter(kafka.WriterConfig{
Brokers: []string{"localhost:9092"},
Topic: "events",
Balancer: &kafka.LeastBytes{},
}),
}
}
func (p *EventPublisher) Publish(event *Event) error {
return p.producer.WriteMessages(context.Background(),
kafka.Message{
Key: nil,
Value: []byte(event.Message),
},
)
}
type EventConsumer struct {
consumer *kafka.Reader
}
func NewEventConsumer() *EventConsumer {
return &EventConsumer{
consumer: kafka.NewReader(kafka.ReaderConfig{
Brokers: []string{"localhost:9092"},
Topic: "events",
Partition: 0,
MinBytes: 10e3, // 10KB
MaxBytes: 10e6, // 10MB
}),
}
}
func (c *EventConsumer) Consume() (*Event, error) {
message, err := c.consumer.ReadMessage(context.Background())
if err != nil {
return nil, err
}
return &Event{
Message: string(message.Value),
}, nil
}
type SomeService struct {
}
func (s *SomeService) HandleEvent(ctx context.Context, event *Event) error {
log.Printf("Received event: %s", event.Message)
// Perform some actions based on the event
return nil
}
func main() {
publisher := NewEventPublisher()
consumer := NewEventConsumer()
conn, err := grpc.Dial("localhost:50051", grpc.WithInsecure())
if err != nil {
log.Fatalf("Failed to connect to the gRPC server: %v", err)
}
defer conn.Close()
client := pb.NewEventServiceClient(conn)
go func() {
for {
event, err := consumer.Consume()
if err != nil {
log.Printf("Failed to consume event: %v", err)
continue
}
_, err = client.HandleEvent(context.Background(), &pb.EventRequest{
Message: event.Message,
})
if err != nil {
log.Printf("Failed to handle event: %v", err)
continue
}
log.Printf("Successfully processed event: %s", event.Message)
}
}()
// Publish events periodically
for {
err := publisher.Publish(&Event{
Message: "Some event",
})
if err != nil {
log.Printf("Failed to publish event: %v", err)
continue
}
log.Println("Published event: Some event")
time.Sleep(1 * time.Second)
}
}
In this example, we have a simple gRPC server that handles incoming event requests. The server receives events from the Kafka topic, processes them, and sends them to the appropriate service depending on the business logic.
The EventPublisher publishes events to the Kafka topic, while the EventConsumer consumes events from the same topic. The SomeService struct represents the service that handles the event, and the HandleEvent method performs the necessary actions based on the received event.
This code snippet provides a basic structure that you can build upon to implement more complex event-driven microservices.
Conclusion
Combining gRPC and Apache Kafka empowers you to build scalable and efficient event-driven microservices. By leveraging event-driven architecture, you can create loosely coupled systems that can handle large-scale data processing and seamlessly integrate with other services. The gRPC framework and Apache Kafka provide the necessary tools and infrastructure to implement such architectures robustly.
Remember that event-driven microservices require careful design and planning. Properly defining events and their schemas is critical for successful communication between services. Additionally, monitoring and observability tools are essential to ensure the health and reliability of your microservice ecosystem.
So go ahead: explore the power of event-driven microservices with gRPC and Apache Kafka, and unlock the full potential of your architecture!